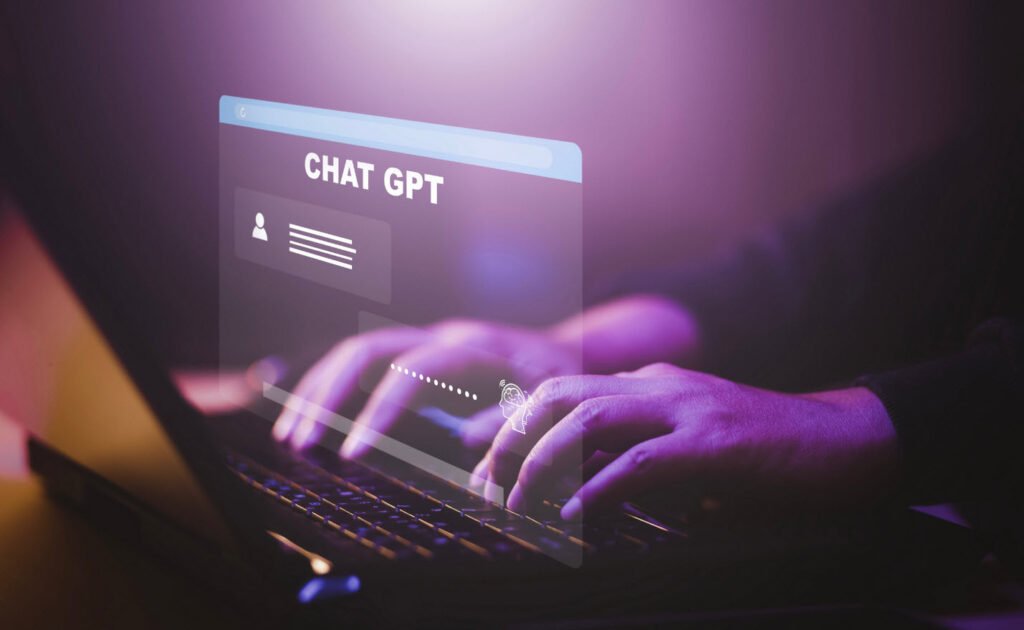
Embarking on coding endeavors can be both challenging and exhilarating. To fuel your programming creativity, here’s a collection of ChatGPT prompts designed to inspire your coding projects:
1. Palindrome Checker Function:
Write a function in your preferred programming language that determines whether a given string is a palindrome.
2. Fibonacci Sequence Calculator:
Create a program that calculates and displays the Fibonacci sequence up to a specified number. Explore different approaches for optimization.
3. Sorting Algorithm Implementation:
Implement a sorting algorithm of your choice (e.g., bubble sort, merge sort) and provide a clear explanation of how it works. Compare its efficiency to other sorting methods.
4. Tic-Tac-Toe Game Simulator:
Develop a program that simulates a simple game of tic-tac-toe between two players. Consider implementing different levels of AI for player versus computer scenarios.
5. Decimal to Binary Converter:
Design a function that converts a decimal number to its binary representation. Explore various methods, and optimize for efficiency.
6. Random Password Generator:
Create a program that generates a random password of a specified length. Ensure it includes a mix of letters, numbers, and special characters for enhanced security.
7. Stack Data Structure Implementation:
Implement a stack data structure and showcase its usage with a practical example. Explore scenarios where a stack can be particularly useful.
8. Recursive Factorial Calculator:
Write a recursive function to calculate the factorial of a given number. Explore the efficiency and limitations of recursive approaches.
9. Bank Account Class Design:
Design a class that represents a bank account, complete with methods for deposit, withdrawal, and balance inquiry. Ensure security measures are considered.
10. CSV Data Analysis Program:
Create a program that reads a CSV file and performs data analysis or manipulation based on the data. Explore libraries that facilitate CSV operations.
11. Binary Search Algorithm:
Implement a binary search algorithm to find a specific value in a sorted array. Compare its efficiency to linear search methods.
12. Multiplication Table Generator:
Write a program that generates a multiplication table up to a specified number. Consider optimizing for readability and aesthetics.
13. Linked List Class Implementation:
Design a class that represents a linked list and implement basic operations like insertion, deletion, and traversal. Explore the advantages of linked lists over arrays.
14. Email Address Format Validator:
Create a program that validates whether a given email address is in the correct format. Consider incorporating regular expressions for precision.
15. Prime Number Checker Function:
Develop a function that checks whether a given number is prime. Optimize for efficiency and explore various prime-checking algorithms.
16. Shopping Cart System Simulation:
Write a program that simulates a basic shopping cart system, allowing users to add, remove, and view items. Consider implementing features like discounts and order summaries.
17. Queue Data Structure Implementation:
Implement a queue data structure and demonstrate its usage with a practical example. Explore real-world scenarios where a queue is advantageous.
18. Library Book Class Design:
Design a class that represents a book in a library, complete with methods for borrowing and returning books. Consider handling multiple copies and due dates.
19. Image Processing Program:
Create a program that performs basic image processing operations, such as resizing or applying filters. Explore libraries that facilitate image manipulation.
20. Tower of Hanoi Solver:
Write a recursive function to solve the Tower of Hanoi puzzle with a specified number of disks. Optimize for efficiency and explore iterative solutions.
Feel free to adapt and customize these prompts to suit your coding preferences and goals. Happy coding!
This Post Has One Comment