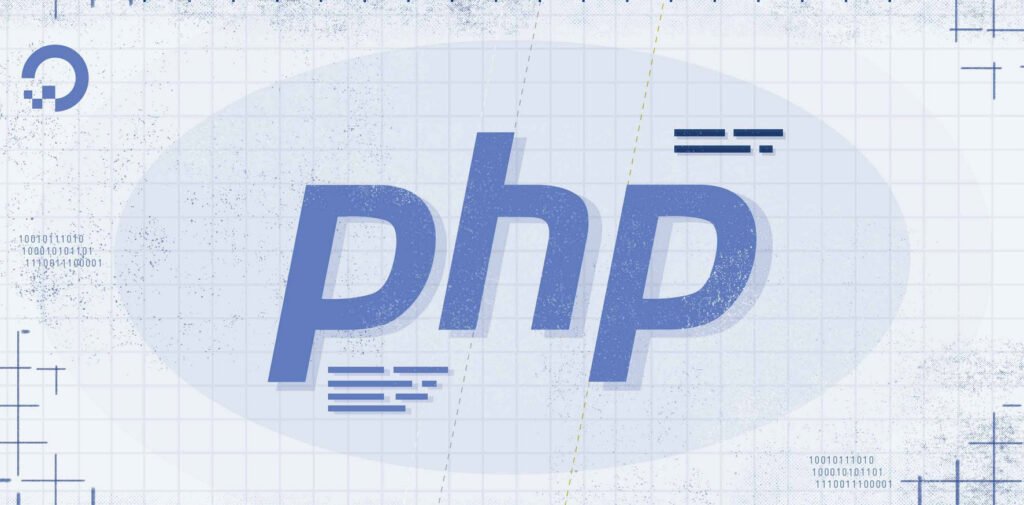
Data types in PHP play a pivotal role in defining the nature of variables and the kind of data they can store. Unlike strictly-typed languages, PHP is loosely typed, meaning that variable types are interpreted at runtime. In this article, we’ll explore the various data types in PHP and understand how they influence the manipulation of data.
1. Integer Data Type
The `int` data type is used to store whole numbers within the range of -2147483648 to 2147483647.
<?php
$x = 999999;
echo $x;
?>
2. String Data Type
The `string` data type represents a sequence of characters.
<?php
$str = "Good morning..";
echo $str;
?>
3. Float Data Type
`float` is used to represent decimal values.
<?php
$x = 79.22;
echo $x;
?>
4. Boolean Data Type
The `boolean` data type is used when a value can only be either `true` or `false`.
<?php
$isAvailable = true;
echo $isAvailable;
?>
5. Array Data Type
The `array` data type allows the storage of multiple values in a single variable. Arrays can contain mixed-type values.
<?php
$arr = array("iPhone", 1000, true);
var_dump($arr);
?>
6. Object Data Type
The `object` data type is employed to store data and information on how to process that data. Objects are declared by explicitly defining classes and creating instances of those classes.
7. Null Data Type
The `null` data type is special and can store only the `null` value.
<?php
$str = "OneCompiler";
$str = null;
var_dump($str);
?>
8. Resource Data Type
The `resource` data type is used to store references to external resources, such as database connections.
Understanding PHP data types is crucial for writing robust and efficient code. Whether you are dealing with numbers, text, or complex structures like arrays and objects, knowing how PHP handles data types allows you to manipulate and process data effectively in your web applications.
This Post Has One Comment