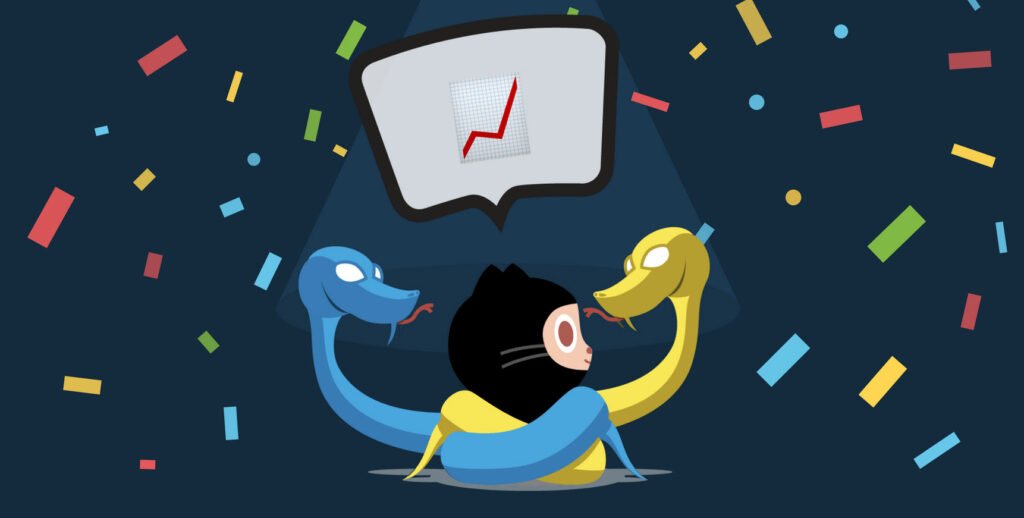
Python is a versatile and powerful programming language that is widely used for various applications, ranging from web development to data analysis and artificial intelligence. One of the reasons for its popularity is its flexibility in handling different types of data.
In Python, data types define the nature of data that a variable can hold. It helps the interpreter understand how the data should be processed and what operations can be performed on it.
Python provides several built-in data types, and in this article, we’ll explore some of the basic ones.
- int (Integer)
- float (Floating-Point)
- char (Character)
- str (String)
- bool (Boolean)
- complex (Complex Numbers)
1. int (Integer)
The `int` data type in Python represents whole numbers, both positive and negative, without any fractional part. Examples of integers are 0, 1, -2, and 3. Python allows you to perform arithmetic operations on integers, such as addition, subtraction, multiplication, and division.
x = 10
y = -5
sum = x + y
print(sum) # Output: 5
2. float (Floating-Point)
The `float` data type is used to represent real numbers with a fractional part. It is essential when precision is required in mathematical calculations. Floats are represented with a decimal point. Examples of floats are 0.1, 4.532, and -5.092.
a = 3.14
b = 2.718
result = a * b
print(result) # Output: 8.539112
3. char (Character)
Unlike some other programming languages, Python does not have a separate data type for individual characters. Instead, characters are simply represented as strings of length 1. So, any single character enclosed in single quotes or double quotes is considered a string in Python.
character_1 = 'a'
character_2 = '@'
print(character_1) # Output: a
print(character_2) # Output: @
4. str (String)
The `str` data type is used to represent sequences of characters, i.e., text. Strings are enclosed within single quotes or double quotes. They are widely used for text manipulation and are immutable, meaning they cannot be changed after creation.
message = "Hello, World!"
name = 'John'
print(message) # Output: Hello, World!
print(name) # Output: John
5. bool (Boolean)
The `bool` data type represents the truth values `True` and `False`. Booleans are used to control the flow of a program through conditional statements like `if`, `else`, and `while`. They are also a result of comparisons and logical operations.
is_raining = True
is_sunny = False
if is_raining:
print("Remember to take an umbrella.")
else:
print("Enjoy the sunny day!")
6. complex (Complex Numbers)
The `complex` data type is used to represent complex numbers in Python. A complex number is a combination of a real part and an imaginary part, represented as `real + imaginary*j`. Complex numbers are mainly used in scientific and engineering applications.
z1 = 2 + 3j
z2 = 4 - 1j
result = z1 * z2
print(result) # Output: (11+10j)
Understanding the basic data types in Python is essential for writing efficient and error-free code. Python’s dynamic typing allows variables to change their data type during runtime, making it a flexible and expressive language.
As you progress in Python programming, you will encounter more advanced data types and structures, but a solid grasp of these fundamental data types is a great starting point for your journey in Python development.
Happy coding!
This Post Has 2 Comments