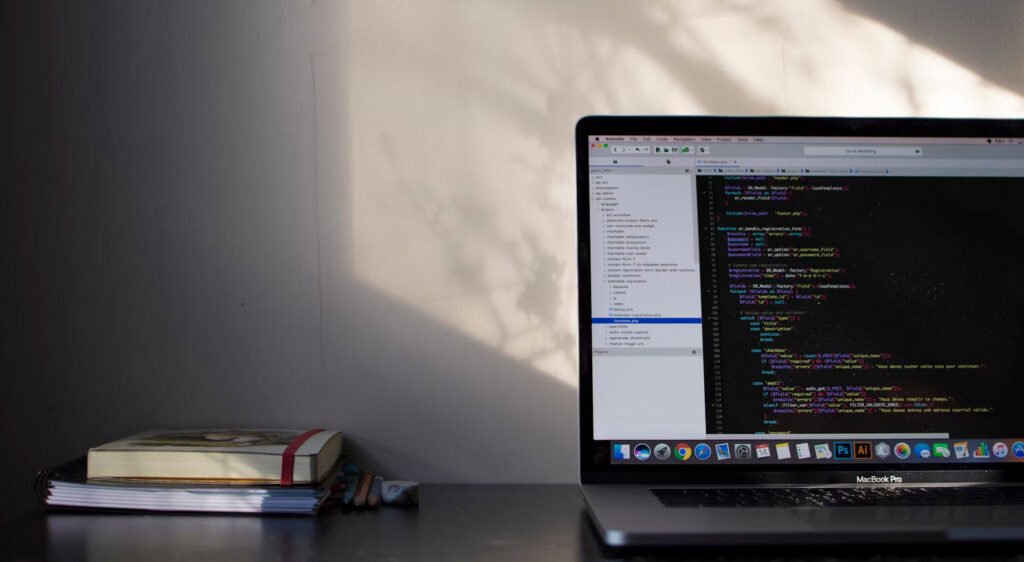
When working with PHP, it’s not uncommon to encounter scenarios where you need to validate or identify the type of a string. Whether it’s checking if a string is numeric, alphabetic, or contains specific characters, PHP offers a set of functions prefixed with `ctype_` that simplifies these tasks.
In this article, we’ll explore various `ctype` functions that allow you to effortlessly check the type of a string.
The ctype Functions Overview
The `ctype` functions in PHP have been available since version 4.0.4 and provide a convenient way to examine the characteristics of strings. Let’s explore into some of these functions:
1. Checking if the String is Alphabetic
To determine if a string contains only alphabetic characters, use `ctype_alpha`:
ctype_alpha('Hello World'); // true
ctype_alpha('Hello World 123'); // false
2. Checking if the String is Numeric
To check if a string consists solely of numeric characters, employ `ctype_digit`:
ctype_digit('123'); // true
ctype_digit('Hello World'); // false
3. Checking if the String is Alphanumeric
To ascertain whether a string contains only alphanumeric characters, use `ctype_alnum`:
ctype_alnum('Hello World 123'); // true
ctype_alnum('Hello World 123!'); // false
4. Checking if the String is Binary
To verify if a string comprises only binary characters, utilize `ctype_xdigit`:
ctype_xdigit('0F'); // true
ctype_xdigit('0FZ'); // false
5. Checking if the String is Whitespace
To determine if a string consists solely of whitespace characters, employ `ctype_space`:
ctype_space("\n\r\t"); // true
ctype_space("Hello World"); // false
6. Checking if the String is Printable
To check if a string contains only printable characters, use `ctype_print`. A string is considered non-printable if it contains control characters like `\n`, `\r`, `\t`, etc.:
ctype_print("Hello World"); // true
ctype_print("Hello World\n"); // false
7. Checking if the String is a Control Character
To verify if a string comprises only control characters, utilize `ctype_cntrl`:
ctype_cntrl("\n\r\t"); // true
ctype_cntrl("Hello World"); // false
8. Checking if the String is a Graph Character
To check if a string contains only graph characters (printable characters excluding space), use `ctype_graph`:
ctype_graph("Hello World"); // true
ctype_graph("Hello World\n"); // false
9. Checking if the String is Lowercase
To determine if a string contains only lowercase characters, use `ctype_lower`:
ctype_lower("hello world"); // true
ctype_lower("Hello World"); // false
10. Checking if the String is Uppercase
To verify if a string comprises only uppercase characters, utilize `ctype_upper`:
ctype_upper("HELLO WORLD"); // true
ctype_upper("Hello World"); // false
11. Checking if the String is Punctuation
To check if a string contains only punctuation characters, use `ctype_punct`:
ctype_punct("*&$()!"); // true
ctype_punct("Hello World!"); // false
In conclusion, PHP’s `ctype` functions provide a straightforward way to validate and classify strings based on their content. By leveraging these functions, developers can easily enhance their string processing and validation workflows, ensuring data integrity and accuracy in PHP applications.
You may also like:- 22 Useful JavaScript Functions You Need To Know
- CSS3 nth-child Selector – A Comprehensive Guide
- PHP Loops – A Comprehensive Guide
- Different Types of Functions in PHP
- Understanding Conditional Statements in PHP
- Mastering PHP Arrays – A Comprehensive Guide
- Exploring Strings in PHP – A Comprehensive Guide
- Performing CRUD Operations with PHP and MySQL
- A Guide to PHP File Operations – Opening, Reading, Creating, Writing, and Closing Files
- How You Can Identify Unused npm Packages in your Project