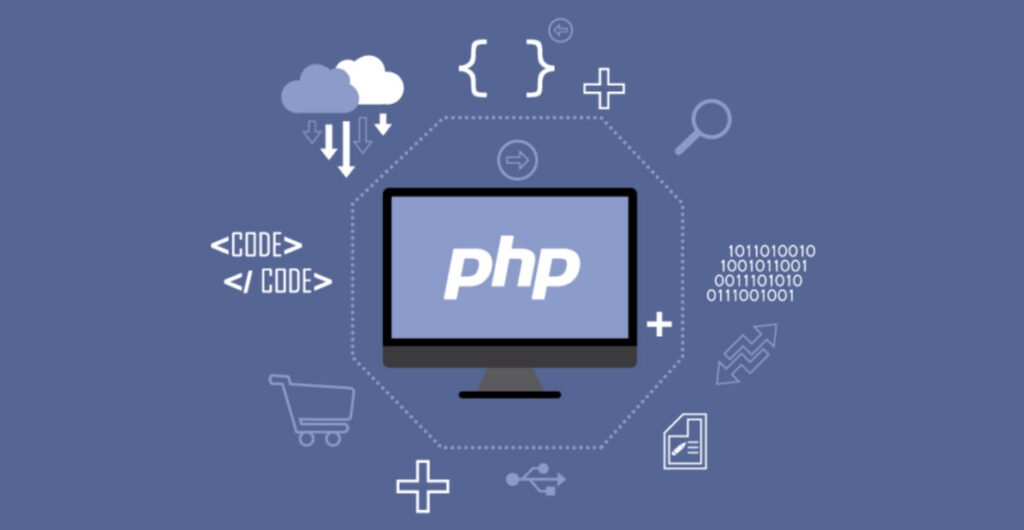
Working with files is a fundamental aspect of many programming tasks. PHP provides a set of functions for file operations, allowing developers to interact with files seamlessly. In this guide, we’ll explore essential PHP file operations, including opening, reading, creating, writing, and closing files.
1. Opening a File with `fopen()`
The `fopen()` function is the gateway to file operations in PHP. It is used to open a file with a specified mode. Here are some common modes:
fopen("filename", "mode");
– `r`: Read-only
– `w`: Write-only
– `a`: Appends data to the end of the file
– `x`: Write-only but returns FALSE if the file already exists
– `r+`: Opens a file for read/write
– `w+`: Opens a file for read/write, overwriting the contents
– `a+`: Opens a file for read/write, appending data to the end of the file
– `x+`: Creates a new file for read/write, returning FALSE if the file already exists
Example:
$file = fopen("example.txt", "w");
fclose($file);
2. Reading a File with `fread()`
The `fread()` function is used to read a specified number of bytes from a file.
fread($filename, filesize)
– `$filename`: Name of the file to read
– `filesize`: Maximum number of bytes to read
Example:
$file = fopen("example.txt", "r");
$data = fread($file, filesize("example.txt"));
fclose($file);
3. Creating a File with `fopen()`
You can use the `fopen()` function to create a new file. Simply specify the file name and the write mode.
Example:
$file = fopen("new_file.txt", "w");
fclose($file);
4. Writing to a File with `fwrite()`
The `fwrite()` function is employed to write data to a file. Remember to open the file before writing and close it afterward.
fwrite($filename, $data);
– `$filename`: Name of the file to write data
– `$data`: Data to write to the file
Example:
$file = fopen("example.txt", "w");
fwrite($file, "Hello, World!");
fclose($file);
5. Closing a File with `fclose()`
It’s crucial to close a file after finishing operations on it. The `fclose()` function accomplishes this.
fclose($filename);
– `$filename`: Name of the file to close
Example:
$file = fopen("example.txt", "r");
// Perform operations
fclose($file);
In summary, PHP provides a robust set of functions for file operations. Whether you’re opening, reading, creating, writing, or closing files, these functions empower developers to handle file-related tasks efficiently.
Integrating these operations into your PHP projects will enhance your ability to manage and manipulate files seamlessly.
You may also like:- 22 Useful JavaScript Functions You Need To Know
- CSS3 nth-child Selector – A Comprehensive Guide
- PHP Loops – A Comprehensive Guide
- Different Types of Functions in PHP
- Various String Types in PHP – Utilizing ctype Functions
- Understanding Conditional Statements in PHP
- Mastering PHP Arrays – A Comprehensive Guide
- Exploring Strings in PHP – A Comprehensive Guide
- Performing CRUD Operations with PHP and MySQL
- How You Can Identify Unused npm Packages in your Project