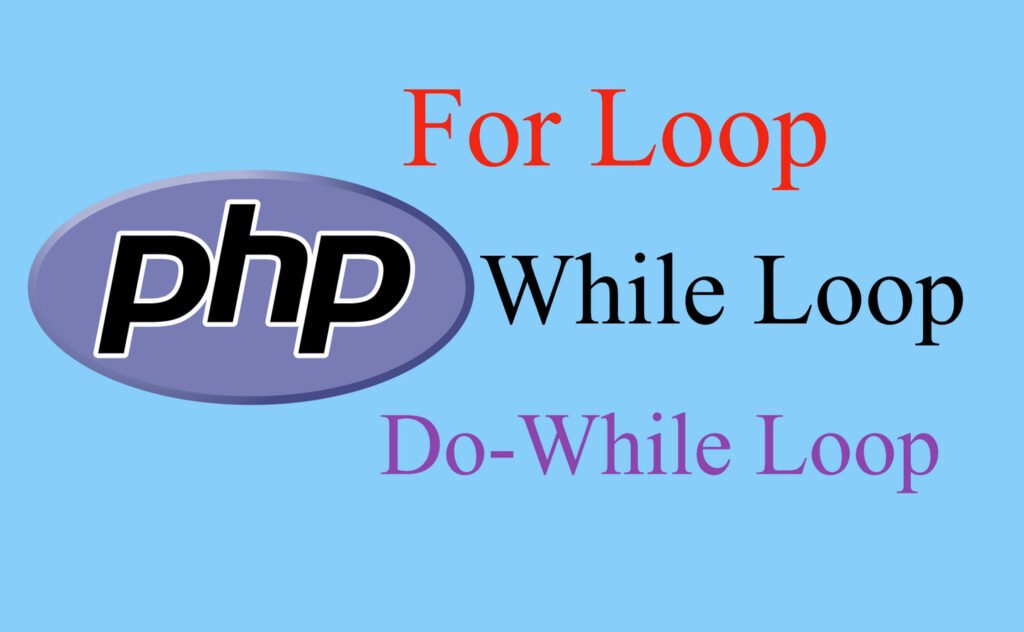
Loops play a fundamental role in programming by allowing the repetitive execution of a set of statements. PHP offers several loop constructs, each serving different purposes.
In this guide, we’ll explore four essential types of loops in PHP: For, Foreach, While, and Do-While.
1. For Loop
Syntax
for(Initialization; Condition; Increment/decrement){
//code
}
Example
<?php
for ($i = 1; $i <= 10; $i++) {
echo("$i\n");
}
?>
Output:
1
2
3
4
5
6
7
8
9
10
2. Foreach Loop
Syntax
foreach ($array as $value) {
//code
}
Example
<?php
$directions = array("East", "West", "North", "South");
foreach ($directions as $value) {
echo "$value \n";
}
?>
Output:
East
West
North
South
3. While Loop
Syntax
while(condition){
//code
}
Example
<?php
$i=1;
while ($i <= 10) {
echo("$i\n");
$i++;
}
?>
Output:
1
2
3
4
5
6
7
8
9
10
4. Do-While Loop
Syntax
do{
//code
}while(condition);
Example
<?php
$i=1;
do {
echo("$i\n");
$i++;
} while ($i<=10);
?>
Output:
1
2
3
4
5
6
7
8
9
10
Understanding when to use each type of loop is crucial for efficient coding. While the For loop is ideal for a known number of iterations, the Foreach loop simplifies array traversing. While and Do-While loops are suitable when the number of iterations is not known in advance, with Do-While ensuring at least one execution.
By mastering these loop constructs, you gain the flexibility and control needed to handle various scenarios in PHP programming. Experimenting with these loops will enhance your proficiency in designing dynamic and responsive PHP applications.