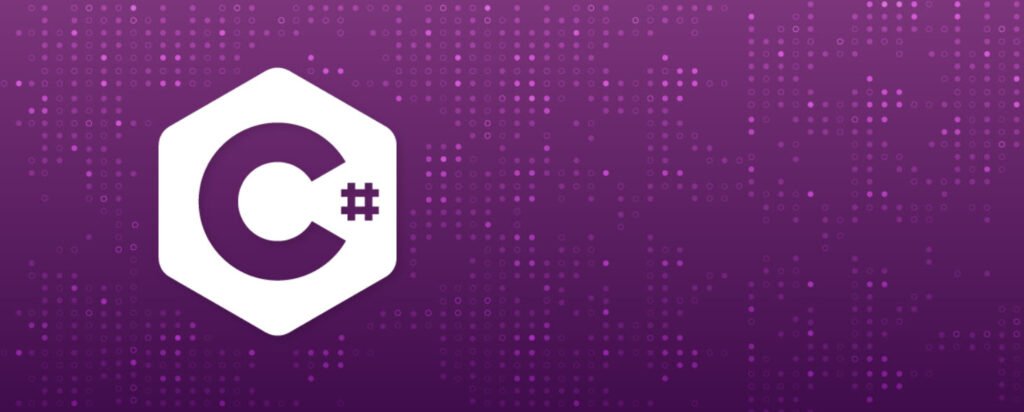
Strings are a fundamental data type in programming that represent sequences of characters. In C#, strings are objects of the `String` class, and they offer a wide range of operations to manipulate and process textual data efficiently.
In this article, we will explore various string operations in C# that allow us to concatenate, format, extract substrings, replace, search, split, and convert strings to character arrays.
- Concatenation and Addition of Strings
- Concatenation with the Plus Operator
- Adding Strings with the `+=` Operator
- Conversion and Formatting
- Using `ToString()` for Conversion
- String Formatting with `String.Format()`
- Substring Operations
- Extracting Substrings with `Substring()`
- Replacing and Searching
- Replacing Substrings with `Replace()`
- Searching Substrings with `IndexOf()` and `LastIndexOf()`
- Splitting and Converting to Character Arrays
- Splitting a String with `Split()`
- Converting String to Character Array with `ToCharArray()`
1. Concatenation and Addition of Strings
1.1 Concatenation with the Plus Operator
string firstName = "Erin";
string lastName = "Roger";
string fullName = firstName + " " + lastName;
C# allows us to concatenate strings using the plus (`+`) operator. In this example, the `fullName` string will contain “Erin Roger” after the concatenation of `firstName` and `lastName`.
1.2 Adding Strings with the `+=` Operator
string secondLastName = "Green";
fullName += secondLastName;
We can also use the `+=` operator to add one string to another. In this case, the `secondLastName` is added to the `fullName`, resulting in “Erin Roger Green.”
2. Conversion and Formatting
2.1 Using `ToString()` for Conversion
object someObject = 42;
string objectAsString = someObject.ToString();
The `ToString()` method is available for all objects in C#. It converts the object into its string representation, making it suitable for display or further processing. In this example, we convert an `int` value (42) into a string.
2.2 String Formatting with `String.Format()`
string formattedString = String.Format("Hello, {0}. Today is {1}.", "John", "Monday");
The `String.Format()` method allows us to create formatted strings with placeholders indicated by curly braces and an index number. The additional arguments passed to the function are substituted into the placeholders based on their index. In this example, we format the string to display a personalized greeting and the day of the week.
3. Substring Operations
3.1 Extracting Substrings with `Substring()`
string originalString = "Hello, World!";
string extractedSubstring1 = originalString.Substring(7); // "World!"
string extractedSubstring2 = originalString.Substring(7, 5); // "World"
The `Substring()` method is used to extract parts of a string. It takes two parameters: the starting index of the substring and, optionally, the length of the substring. If the length is not specified, the method returns the remaining part of the string starting from the specified index.
4. Replacing and Searching
4.1 Replacing Substrings with `Replace()`
string oldStr = "The old brown dog";
string newStr = oldStr.Replace("old", "new");
The `Replace()` method allows us to replace occurrences of a specified substring with a new string. In this example, we replace “old” with “new,” resulting in “The new brown dog.”
4.2 Searching Substrings with `IndexOf()` and `LastIndexOf()`
string sentence = "The quick brown fox jumps over the lazy dog";
int firstOccurrence = sentence.IndexOf("fox"); // 16
int lastOccurrence = sentence.LastIndexOf("the"); // 35
The `IndexOf()` method searches for the first occurrence of a specified substring within a string and returns its index. If the substring is not found, it returns -1. The `LastIndexOf()` method works similarly but searches the string from the end, finding the last occurrence.
5. Splitting and Converting to Character Arrays
5.1 Splitting a String with `Split()`
string fruits = "apple,orange,banana";
string[] fruitArray = fruits.Split(',');
The `Split()` method is used to break a delimited string into substrings based on a specified separator. In this example, the `fruits` string is split into an array of three elements: “apple,” “orange,” and “banana.”
5.2 Converting String to Character Array with `ToCharArray()`
string str = "AaBbCcDd";
char[] chars = str.ToCharArray();
char[] selectedChars = str.ToCharArray(2, 2); // {'B', 'C'}
The `ToCharArray()` method converts a string into a character array. In the first example, the entire string is converted into an array of individual characters. In the second example, we specify a starting index (2) and the number of characters to copy (2), resulting in a character array containing ‘B’ and ‘C’.
Conclusion
String operations in C# are powerful tools that allow us to manipulate textual data efficiently. With string concatenation, formatting, substring extraction, replacement, and searching, you can perform various text-related tasks in your C# programs.
Additionally, the ability to split strings into substrings and convert them into character arrays provides further flexibility when dealing with text-based information. Mastering these string operations will undoubtedly enhance your C# coding skills and enable you to work with textual data effectively.
You may also like:- 22 Useful JavaScript Functions You Need To Know
- CSS3 nth-child Selector – A Comprehensive Guide
- PHP Loops – A Comprehensive Guide
- Different Types of Functions in PHP
- Various String Types in PHP – Utilizing ctype Functions
- Understanding Conditional Statements in PHP
- Mastering PHP Arrays – A Comprehensive Guide
- Exploring Strings in PHP – A Comprehensive Guide
- Performing CRUD Operations with PHP and MySQL
- A Guide to PHP File Operations – Opening, Reading, Creating, Writing, and Closing Files