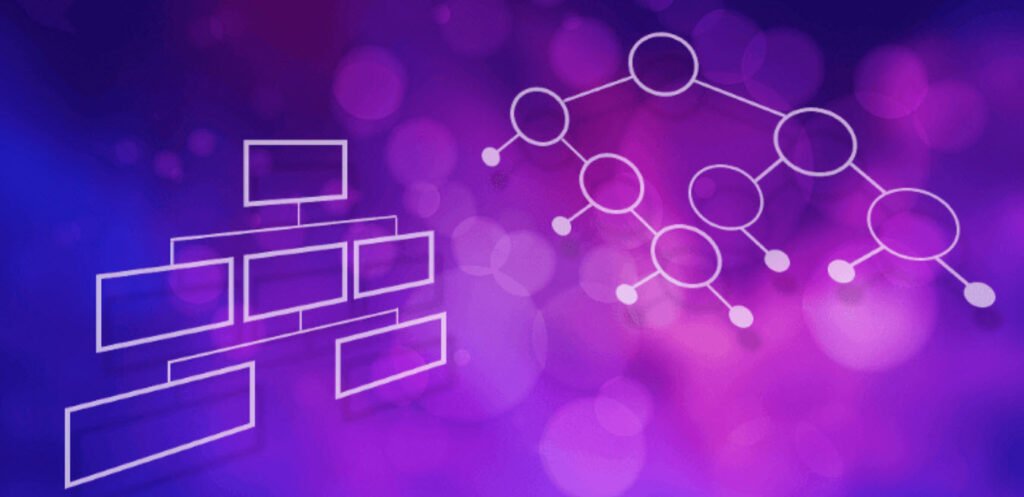
Data structures are the fundamental building blocks of computer science and software development. They are essential for efficiently organizing and managing data in computer programs. Whether you’re a job seeker preparing for a technical interview or a student studying data structures, mastering these concepts is crucial.
In this article, we’ve compiled 200 data structures interview questions to help you understand and practice these important topics.
- What is an abstract data type?
- What abstract data type would be used to store a whole number?
- Explain how a memory address is used to access an abstract data type that is larger than 1 byte.
- What is the difference between a float abstract data type and a double abstract data type?
- What is precision?
- Explain how memory is organized within a computer.
- What is a numbering system?
- Why is the binary numbering system used in computing?
- Why don’t you directly specify the number of bytes to reserve in memory to store data?
- Explain the impact signed and unsigned numbers have on memory.
- What is a user-defined data type?
- How do you determine the size of a structure?
- Why would you use a structure?
- Why happens when you declare an instance of a structure?
- How do you access parts of a structure?
- What is a pointer?
- Why would you use a pointer in a program?
- What is a pointer to a pointer?
- Why would you use a pointer to a pointer in a program?
- What is shown on the screen if you display the content of a pointer variable?
- What is the difference between an array element and a variable?
- What is an array of pointers?
- How do you assign an address to an element of a pointer array?
- What is an array of pointers to pointers?
- How do you assign a value to an element of an array of pointers to pointers?
- How do you display the contents of the memory addresses stored in an element of a pointer array?
- Why would you use an array of pointers to pointers?
- How do you declare an array?
- How do you display the contents of the memory addresses stored in an element of an array of pointers to pointers?
- How are elements of an array stored in memory?
- What is a stack?
- What is the purpose of the push() member method?
- What is the purpose of the pop() member method?
- What is the purpose of the isFull() member method?
- What is the purpose of the isEmpty() member method?
- What kind of value is assigned to the top attribute?
- Why is the top attribute initialized to –1?
- What is the purpose of the keyword private?
- What is the purpose of the keyword public?
- What is the difference between a constructor and a destructor?
- What is a queue?
- What is the relationship between a queue and its underlying array?
- Explain how the index of the front and back of the queue is calculated.
- What is the purpose of the enqueue process?
- What is the purpose of the dequeue process?
- Why is the isFull() member method called?
- Why is the isEmpty() member method called?
- What happens to the data stored on the array when the data is removed from the queue?
- What is the purpose of setting the default size of the queue?
- Why does the C++ version of the queue delete the underlying array from memory using the destructor and the Java version of the queue does not?
- What is a linked list?
- What is the benefit of using a linked list?
- What is a node?
- What are the elements of a node?
- What advantage does a linked list have over an array?
- Can a node reference more than one data element?
- In Java, why can’t you pass a primitive data type to the add() method of the LinkedList class?
- How can a node be inserted in the middle of a linked list?
- What is a doubly linked list?
- What is a single linked list?
- What is a stack-linked list?
- How does a stack-linked list differ from a linked list?
- What is the benefit of using a stack-linked list?
- Where is the front of the stack in a stack-linked list?
- What is the maximum number of nodes that you can have on a stack-linked list?
- Can a node on a stack-linked list have more than one data element?
- Why does the StackLinkedList class inherit the LinkedList class?
- Why is the constructor of the StackLinkedList class empty?
- Why is the destructor of the StackLinkedList class empty?
- What happens when you push a new node onto a stack?
- What is a queue linked list?
- How does a queue linked list differ from an array queue?
- What is the benefit of using a queue linked list?
- Where are new nodes added to the queue?
- Which node is removed from the queue when the dequeue() member method is called?
- Can a node on a queue linked list have more than one data element?
- What form of access is used to add and remove nodes from a queue?
- Why is the constructor of the QueueLinkedList class empty?
- Why does the QueueLinkedList class inherit the LinkedList class?
- What happens when dequeue() is called?
- What is a linked list index?
- What is the value of the first linked list index?
- What is the difference between the removeNode() and deleteNode() functions?
- What is the return value of the findNode() function?
- What is the difference between the insertNodeAt()and the appendNode() functions?
- What happens if an invalid index value is passed to a function?
- Can a linked list store data other than integers?
- Why would you define a getSize() function instead of having the application access the size of the linked list directly?
- Can the insertNodeAt() function place a node at the front or back of a linked list?
- Why is it important to enhance the functionality of the LinkedList class?
- What is a tree?
- What is the relationship between parent node and child nodes?
- What are a key and a value?
- What is a root node?
- What is the purpose of a left child node and a right child node?
- What is a leaf node?
- What is the depth of a tree?
- What is the size of a tree?
- How do you calculate the size of a tree?
- Can a tree have a duplicate key?
- What is hashing?
- Why is it necessary to hash?
- What is a hashtable?
- What is the result of hashing?
- How is a key hashed?
- Can a key entered by an application be directly compared to a key in a hashtable?
- What programming technique is used for hashing in all hashing functions?
- Why are data members of the Hashtable class stored in the private access specifier?
- At what level is hashing performed?
- Is there one hashing function used in all applications for hashing?
- What is the binary numbering system?
- What is the purpose of an abstract data type?
- What is a variable?
- What is the integer abstract data type group?
- What does the term “floating-point” mean?
- What is a character?
- What is the difference between single and double precision?
- What is an instance of a structure?
- What kind of data type is a structure?
- How do you reference an element of an instance of a structure?
- What are three major elements of every class definition?
- What is the difference between a class definition and a structure definition?
- What is the hexadecimal numbering system?
- How do you assign an address to a pointer?
- What value is stored in a pointer variable?
- Why do you use pointer arithmetic?
- What is a pointer-to-pointer variable?
- What is an array of elements?
- What is an index?
- What is a multidimensional array?
- What is the purpose of using a multidimensional array?
- What is the relationship between a pointer and an array?
- What is the relationship between a stack and an array?
- What is the action called that places data on a stack?
- What is the action called that removes data from a stack?
- Using an array, how do you determine whether the stack is empty?
- Using an array, how do you determine whether the stack is full?
- Where is data organized in a queue stored?
- What does the term “circular queue” mean?
- What is the modulus operator used for with respect to circular queues?
- When would you implement a queue using a linked list instead of an array?
- What is the action called that places data on a queue?
- What is the action called that removes data from a queue?
- What is an entry in a linked list called?
- How are nodes linked together in a linked list?
- What is the purpose of a doubly linked list?
- What is used to define a node of a linked list?
- How do you delete an element from the middle of a linked list?
- How do you delete a node from the front of a linked list?
- How do you append a node onto a linked list?
- How do you put a new node onto the front of the linked list?
- What condition tells you the linked list is currently empty?
- What condition tells you there’s only one node on the linked list?
- What is the size limitation on a linked list?
- What is the destructor typically used for in a linked list?
- What is the key used for in a hashtable?
- What is the significance of a hash value?
- What major problem occurs with hashing?
- How do you overcome the major problem that occurs with hashing?
- Ideally, how should a hash function behave with respect to the values it generates? If you feed in a list of keys, what would you expect for the output?
- Many different hashing algorithms have been developed to provide a more even distribution of hash values. What is the essence of the hashing algorithm—in other words, what do these functions typically have in common?
- With a hashtable, suppose your dataset gets unexpectedly large and you have an excessive number of collisions. How could you deal with this?
- How do you insert a node into a hashtable?
- How do you delete a node from a hashtable?
- How do you look up a value in a hashtable?
- How do you list out all the values in a hashtable?
- How would you check if a hashtable is empty?
- What is a binary tree?
- What is the purpose of a branch node in a binary tree?
- What is the starting node of a binary tree called?
- What is the node in a binary tree called that spawns another node?
- What are nodes at the end of a binary tree called?
- If you have 1,000 nodes in a balanced binary tree, approximately how many comparisons do you need to do to find a particular node?
- How is the maximum depth of a tree defined?
- If a binary tree is well balanced, approximately how many nodes are in the tree given the depth of the tree?
- What condition do you check for to see if a node in a binary tree is a leaf node?
- How do you delete a node from a binary tree that has two child nodes?
- How do you delete a node from the binary tree that has one child node?
- How do you delete a leaf node from a binary tree?
- What is the basic rule for where the nodes get placed into a binary tree?
- How do you insert a node into a binary tree?
- How would you check if a binary tree is empty?
- What is a recursive function?
- When searching for a key in a binary tree, what stops the recursive function calls?
- What is the sequence of function calls to do an “in order” traversal of a binary tree?
- What is memory allocation?
- What does the new operator return?
- Does Java use pointers?
- How do you declare an array of pointers?
- How do you declare an array of pointers to pointers?
- If an int pointer is incremented using pointer arithmetic, how many bytes is the pointer incremented?
- How is a binary tree’s depth defined?
- What is a balanced binary tree?
- Must all binary trees be balanced?
- What is the purpose of a key in a binary tree?
- What is metadata?
- What is the purpose of the this operator?
- What does a private access specifier do?
- What is fifo?
- What is the purpose of a public access specifier?