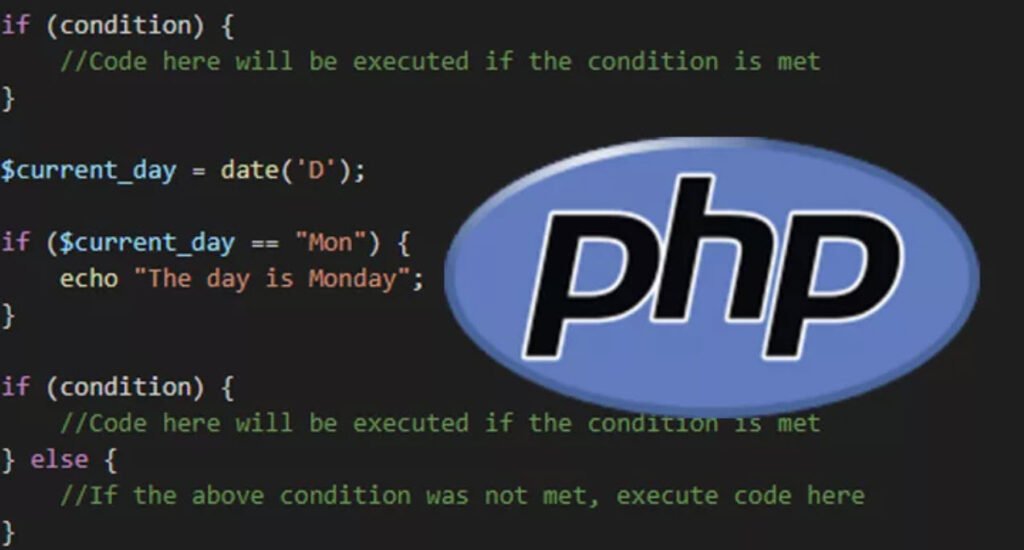
Conditions and loops are fundamental aspects of programming that enable developers to control the flow of code execution and repeat tasks efficiently. In PHP, conditions allow you to execute certain blocks of code based on specific conditions, while loops enable you to iterate through arrays or perform actions multiple times until a certain condition is met.
In this article, we will explore PHP conditions and various looping methods, highlighting their syntax and use cases.
PHP Conditions:
1. If/Elseif/Else:
The if/elseif/else statements are used for conditional branching in PHP. They allow you to execute different blocks of code based on the evaluation of conditions.
if ($i > 10) {
// Code block executed when $i is greater than 10
} elseif ($i > 100) {
// Code block executed when $i is greater than 100, but not greater than 10
} else {
// Code block executed when none of the above conditions are met
}
2. Ternary Operator:
The ternary operator is a shorthand way of writing simple if/else statements. It’s used when you want to assign a value to a variable based on a condition.
$string = $state == 'Running' ? 'He is running' : 'I don\'t know';
In the example above, if the variable `$state` is equal to ‘Running’, the string ‘He is running’ will be assigned to `$string`. Otherwise, ‘I don’t know’ will be assigned.
3. Null Coalescing Operator:
The null coalescing operator (`??`) is used to assign a default value to a variable if it is null.
$string = $startDate ?? '';
In this example, if the variable `$startDate` is not null, its value will be assigned to `$string`. Otherwise, an empty string will be assigned.
Different Ways of Looping:
1. Foreach Loop:
The foreach loop is used to iterate over elements in an array, assigning each element’s key and value to specified variables.
foreach ($arr as $key => $value) {
// Code block for each iteration
// $key will contain the key of the current element
// $value will contain the value of the current element
}
2. For Loop:
The for loop is used for numerical iteration, providing more control over the loop index.
for ($i = 0; $i < count($arr); $i++) {
// Code block for each iteration
// $i is the loop index, and $arr[$i] contains the current element
}
3. While Loop:
The while loop is used to repeat a block of code as long as a specific condition remains true.
$i = 0;
while ($i < count($arr) - 1) {
// Code block for each iteration
// $arr[$i] contains the current element
$i++;
}
4. Do-While Loop:
The do-while loop is similar to the while loop but ensures that the code block executes at least once before checking the condition.
$i = 0;
do {
// Code block for each iteration
// $arr[$i] contains the current element
$i++;
} while ($i < count($arr));
5. Switch Statement:
The switch statement is used to perform different actions based on the value of a variable or an expression.
switch ($variable) {
case 1:
// Code block executed when $variable equals 1
break;
case 2:
// Code block executed when $variable equals 2
break;
case 3:
// Code block executed when $variable equals 3
break;
default:
// Code block executed when none of the above cases are met
}
Conclusion
In PHP, conditions and loops are powerful tools that provide control and flexibility to your code. You can use conditions to execute specific code blocks based on various conditions, while different loop types allow you to iterate through arrays and perform tasks repeatedly.
Understanding these PHP constructs will greatly enhance your ability to build dynamic and interactive web applications effectively. By utilizing conditions and loops wisely, you can create more efficient and organized code that meets the needs of your projects.
This Post Has One Comment