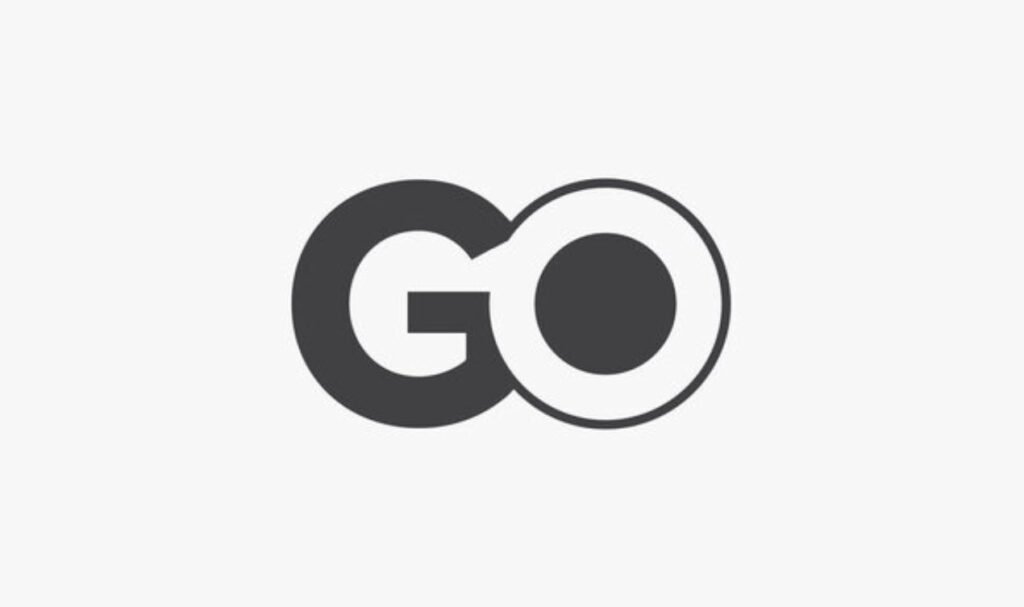
Conditional statements are essential constructs in any programming language that allow developers to control the flow of execution based on specific conditions. In Go, a statically-typed, compiled language, you can use various conditional statements like if/else and switch to make decisions and handle different scenarios efficiently.
In this article, we will explore the different types of conditional statements available in Go and how they can be effectively used in your programs.
1. If/Else Statements:
The if/else statement is a fundamental conditional construct that executes a block of code based on a given condition. If the condition evaluates to true, the code inside the if block executes; otherwise, the code inside the else block executes.
i := 1
if i > 0 {
// Condition is true! i is greater than zero
} else {
// Condition is false! i is lower or equal to zero
}
2. Else If Statements:
The else if statement extends the if/else construct to handle multiple conditions. It allows you to check additional conditions when the first condition is false.
i := 1
if i > 0 {
// Condition is true! i is greater than zero
} else if i > 0 && i < 2 {
// Condition is true! i is greater than zero and lower than two
} else if i > 1 && i < 4 {
// Condition is true! i is greater than one and lower than four
} else {
// None of the above conditions is true, so it falls here
}
3. If with Short Statements:
Go allows you to declare a variable inside the if statement and use it within the block. This feature is useful when you need a temporary variable for a specific condition.
i := 2.567
if j := int(i); j == 2 {
// Condition is true! j, the integer value of i, is equal to two
} else {
// Condition is false! j, the integer value of i, is not equal to two
}
4. Switch Statements:
The switch statement provides an elegant way to handle multiple conditions. It works with constants and expressions and allows you to match the value of a variable against a set of predefined cases.
text := "hey"
switch text {
case "hey":
// "Hello!"
case "bye":
// "Byee"
default:
// "Ok"
}
5. Switch Without Condition:
Go allows you to use a switch statement without a condition, and each case can have an expression. The first matching case will be executed.
value := 5
switch {
case value < 2:
// "Hello!"
case value >= 2 && value < 6:
// "Byee"
default:
// "Ok"
}
Conclusion
Go provides a variety of conditional statements that enable developers to control the flow of their programs based on different conditions. Whether it’s a simple if/else statement, a more complex else if construct, or the versatility of the switch statement, Go offers powerful and flexible tools for making decisions in your code.
By using these conditional statements effectively, you can create more dynamic and interactive applications, handle different scenarios, and improve the overall user experience. As you gain more experience with Go, mastering conditional statements will become second nature, allowing you to write more efficient and well-structured code for your projects.
You may also like:- 22 Useful JavaScript Functions You Need To Know
- CSS3 nth-child Selector – A Comprehensive Guide
- PHP Loops – A Comprehensive Guide
- Different Types of Functions in PHP
- Various String Types in PHP – Utilizing ctype Functions
- Understanding Conditional Statements in PHP
- Mastering PHP Arrays – A Comprehensive Guide
- Exploring Strings in PHP – A Comprehensive Guide
- Performing CRUD Operations with PHP and MySQL
- A Guide to PHP File Operations – Opening, Reading, Creating, Writing, and Closing Files