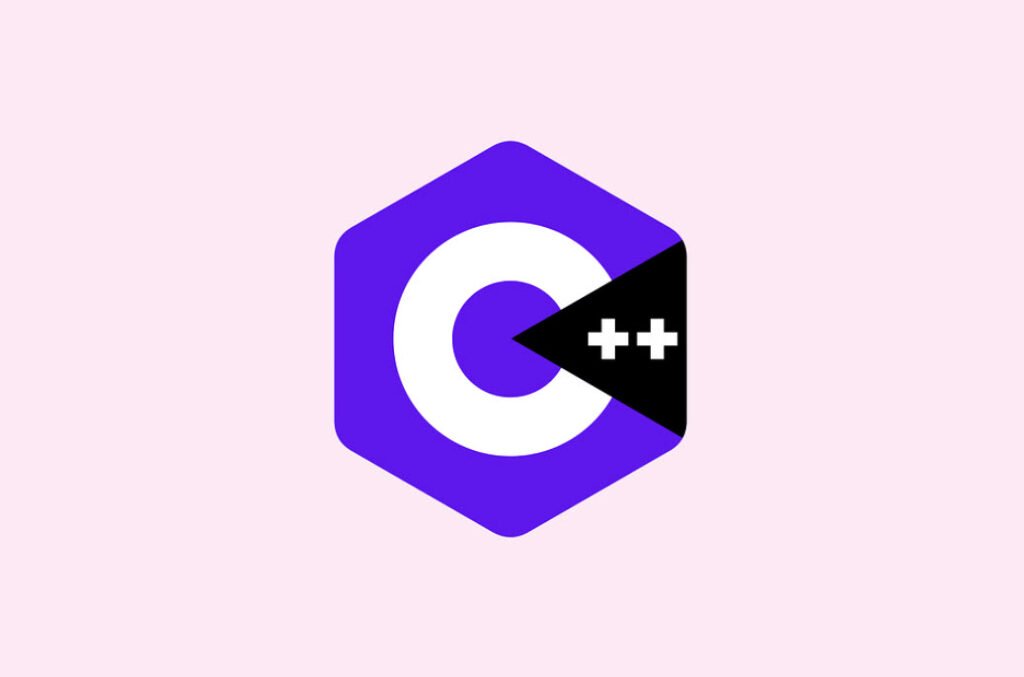
A program’s fundamental job is to accept some data as input and produce the required data as output. All languages provide some mechanism for performing Input/Output operations through which use communicates with a program. A good I/O system must support input and output through a variety of devices such as keyboard, printer, monitor, disk etc. and also handles inputting and outputting different types of data.
Index:
- [#1] – Introduction to C++ – Questions
- [#2] – Preparing and Running C++ Program – Questions
- [#3] – C++ Fundamentals – Questions
- [#4] – Data Input and Output – Questions
- [#5] – Control Structures – Questions
- [#6] – Functions – Questions
- [#7] – Arrays and Strings – Questions
- [#8] – Structures and Unions – Questions
- [#9] – Pointers – Questions
- [#10] – Object and Classes – Questions
- [#11] – Constructors and Destructors – Questions
- [#12] – Operator Overloading and Type Conversion – Questions
- [#13] – Inheritance – Questions
- [#14] – Virtual Functions – Questions
- [#15] – C++ Streams and Files – Questions
- [#16] – Templates – Questions
- [#17] – Exception Handling – Questions
In addition to these properties, I/O system must be capable of handling error conditions that normally occur during I/O operations like incorrect input data etc.
C++ supports a rich set of I/O functions for performing I/O operations. It can also use all the I/O functions of C language in C language in the C++ program. But we normally avoid use of C’s I/O functions like scanf() and printf() etc. because of their inefficiency to handle errors properly, not flexible enough to handle user-defined data types such as classes and in the worst case they are not object oriented at all.
Key Points To Remember –
- In C++ input/output operations occurs in the form of streams. A stream refers to the flow of data. It acts as an intermediator between a wide variety of 1/O derives and programs by providing a consistent and device independent interface to the programmer.
- Streams can be classified as Input and Output stream. In input stream, the data flows from the input device to the program and in case of output stream, data flows from the program to the output device.
- C++1/O system supports a hierarchy of stream classes that manage both the console and disk files 1/O operations. At the top of the hierarchy we have ios base class. The istream and ostream classes derived from ios base class control the stream input and output operations.
- The classes istream and ostream are derived virtually from ios base class so that only a single copy of the numbers of ios class are inherited by the iostream class.
- The istream object cin is connected to the standard input device normally the keyboard and ostream object cout is connected to the standard output device normally the monitor.
- The permanent storage of data an a disk can be done using the concept of files. A file is collection of related data stored on a disk.
- Files in C++ are associated with objects of various classes. Typically, ifstream object for input, ofstream object for output and fstream object for both input and output.
- There are two ways of opening a file. Either using the open() function of the class or constructor of the appropriate stream class.
- Files which are opened can be closed either explicitly using the close() function or implicitly by the destructor of the appropriate stream class.
- The open() function consists of two arguments first represents the file name and the second represents the file open mode.
- For ofstream object, the file open mode can either be ios:: out to output data to a file or ios:: app to append data at the end of the file.
- Files can be of two types-text files and binary files. A text file is a stream of characters with some characters to mark the end of each line. But a binary file, stores data to disk in the same form in which it is represented in memory.
- The ostream member function write() outputs to a specified stream a number of bytes beginning at a designated location in memory and istream member function read() extracts specified member of bytes from the specified stream to an area in memory beginning with a designated address. These functions can perform input or output operations with entire object at a time.
- In C++, files can be accessed either sequentially or randomly. In case of sequential files, the file pointer used for accessing the data moves to the next byte following the number of bytes read. However in case of random access file. we can access any information by skipping as many bytes as required from the current position of the file.
- Every file is associated with get and put pointer. The get pointer specify the location in the file from where the next input operation will occur. The put pointer on the other hand specify the location in the file from where the next output operation will occur.
- Both ifstream and ostream classes provide member functions for moving the file pointer from one position to another position to another position within the file. These function are seekg() and seekp() that moves the get pointer and put pointer within a file respectively.
- tellg() and tellp() are the member functions of class istream and ostream respectively that return the current position of the get and put pointers respectively.
- There may be a number of errors that may occur while working with a file. C++ I/O system provides a number of functions like fail(), eof(), bad(), good() etc. which are used to deal with errors.
- The arguments which are passed on to the main() by the user at the commend prompt are known as Command Line Arguments. They must be separated by an while space. The first argument argc receives the number of command line arguments and the second argument argv is an array of char*‘s pointing to strings in which actual command line arguments are shown.
- The insertion and extraction operator can be overloaded to input or output an object to condole I/O derives and disk files.
Viva Voce Questions –
Here are commonly asked questions with answers:
- Which of the predefined stream objects are automatically opened when the program execution starts ?
- cin, cout, cerr and clog.
- How is the cascading of insertion operator performed ?
- Consider the statement,
cout<<a<<b;
The result of the expression ‘cout<<a’ is the cout object itself which is then reapplied to the operand b, which is then computed.
- Consider the statement,
- What is the difference between predefined stream object cerr and clog ?
- Both cerr and clog pre-defined objects are used to display error messages on the screen. The difference is that error messages to be displayed using cerr are unbuffered whereas it is fully buffered in the case of clog.
- When does the expression while (cin>>val) become false ?
- When Ctrl+z is pressed, end of file is read or an invalid value has been encountered ?
- How does the insertion operator works with different types of data ?
- The insertion operator (<<) is a member function of class ostream where it is overloaded to work with different types of data.
- Do the following statements execute properly or not ? If not, why ?
cin.get(a,50);
cin.get(b,30);
where ‘a’ and ‘b’ are both character arrays.- No, The reason is that when we input an ayyay of characters a using get () function from input atream then the newline character ‘n’ itself is not read but is left as next character of the stream, which is then read by bext get() statement so these consective get () statement can result in error.
Inorder to get rid of this pproblem, remove ‘n’ character from the stream using the member function ignore () as follows
cin.get(a,50) ;
cin.ignore ();
cin.get(b,50) ;
- No, The reason is that when we input an ayyay of characters a using get () function from input atream then the newline character ‘n’ itself is not read but is left as next character of the stream, which is then read by bext get() statement so these consective get () statement can result in error.
- Why getline() is preferred over get() for inputting multiline strings ?
- Since getline () functions discards the delimiter rather than leaving it in the input stream until the next input operation. This is not in the caseof get() function.
- What is the drawback of extraction operator while taking input for character array ?
- The problem is that multiword strings cannot be inputted using the extraction operator as it discard characters after the first white space. This problem can be solved by using get function.
- Can we cascade put() function ?
- Yes, as follows,
cout.put(‘a’) .put(‘b’) ;
- Yes, as follows,
- Which header file needs to be included for performing file I/O operation ?
- fstram.h
- What are different ways of opening a file ?
- 1. Using one argument constructor of appropriate stream class Eg.
ifstream in(“abc. txt”) ;
2. Using open () member function. Eg
- 1. Using one argument constructor of appropriate stream class Eg.
- Is it necessary to close the file explicitly ?
- Yes, if the programmer wants to open the file in different modes later on.
- Is there any limit to the number of files be opened ?
- Yes, but it is depends on the operating system on which the program executes. In MS-DOS, the config.sys file contains the statement files=n;
when n specifies the maximum number of files to opened which can be changed.
- Yes, but it is depends on the operating system on which the program executes. In MS-DOS, the config.sys file contains the statement files=n;
- What is the purpose of ‘nocreate’ and ‘noreplace’ opening modes while opening a file ?
- The nocreate opening mode ensures that file must exist before opening it and noreplace opening mode takes care that while opening the file for output, it doesnot get overridden with the newone unless ate or app is set.
- What is the default file opening mode of ofstream class object ?
- It is ios:: trunc
- What is basic difference between text and binary files ?
- A text file is the one in which data is stored in the form of ASCII characters and ‘n’ is converted int ‘r’ and ‘n’. But in the binary file, data is stored in the same way as it is stored in main() memory and no conversions takes place.
- What is the difference between tellg() and tellp() ?
- tellg() is a number of class ifstream and returns the current position of get pointer and tellp() is a member and returns the current position of put pointer.
- What does the following statement mean,
ifstream_obj.seekg(-2,ios::end);- It means move the get pointer two positions backward from the end.
- How will you check whether input data is valid or invalid ?
- It is possible uing the fail() function.
Other Similar Questions –
- Define stream. Explain different types of streams
- Explain the predefined streams present in C++ I/O system.
- Design and explain the hierarchy of console stream classes.
- Describe various input functions present in ‘istream’ class.
- Explain the following ostream member functions
put(), write(), flush() - Distinguish between get() and getline() function ?
- Discuss the following formatted member functions of ‘ios’ class.
width(), fill() , precision(). - What is a manipulator ? Compare the various manipulators declared in ‘iomanip.h’ with equivalent functions of ‘ios’ class.
- Can a user create his own manipulator ? If yes, then how ?
- Defines files ? Why do we need it ?
- Discuss various file stream classes and how they are related to each other in a stream classes hierarchy.
- Describe two methods of opening a file ? Which one should be preferred over the other ?
- What are the steps involved for opening a file ?
- Is it necessary to close a file explicitly ? If no, why ?
- What is a file mode ? Describe the various modes available for opening a file.
- What are possible reasons for failure of open() function ?
- Discuss the default opening modes of various file stream classes.
- Distinguish a text file and a binary file.
- How do we perform reading and writing of a class object ?
- How is a binary file opened ?
- What are file pointers ? Describe get and put pointers.
- Distinguish between sequential and random files.
- Write short notes on: seekg(), seekp(), tellg(), tellp()
- When do we need to create an object of fstream class ?
- What are the different types of errors that may occur while working with files.
- Describe the various error handling functions.
- Discuss the various error status flags present in ‘ios’ class.
- What are the command line arguments ?
- How do we run a program using command line arguments.
- What are the advantages of saving data in a binary file ?
- How do you count total number of objects stored in a file ?
- Can we overload insertion and extraction operators to input and output an object to console I/O devices and disk files ? If yes, how ?
- How can we send output to the printer ?
- Write statements using seekg() to perform the following tasks ?
- to move the pointer by 10 positions forward from the beginning.
- to go to the end of file.
- to move the pointer 5 positions forward from current position.
- to move the pointer 3 positions backward from the end.
- Penetration Testing Quiz – 20 Questions to Test Your Skills and Learn
- Top 30 Linux Questions (MCQs) with Answers and Explanations
- 75 Important Cybersecurity Questions (MCQs with Answers)
- 260 One-Liner Information Security Questions and Answers for Fast Learning
- Top 20 HTML5 Interview Questions with Answers
- 80 Most Important Network Fundamentals Questions With Answers
- 100 Most Important SOC Analyst Interview Questions
- Top 40 Cyber Security Questions and Answers
- Top 10 React JS Interview Theory Questions and Answers
- CISSP – Practice Test Questions – 2024 – Set 20 (53 Questions)