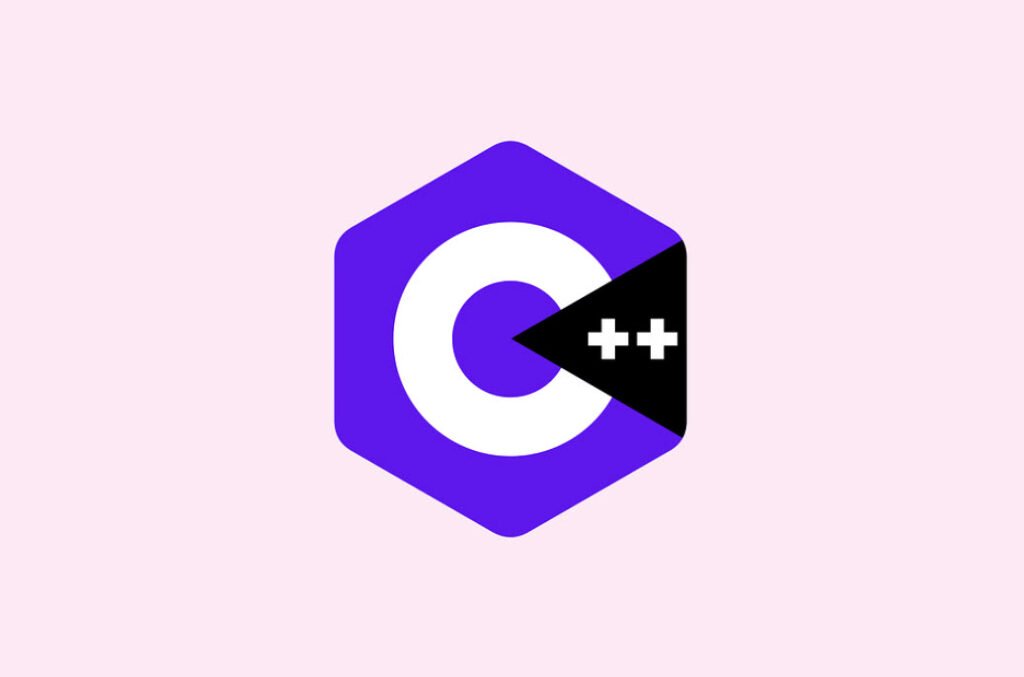
An array is a collection of logically related variables of identical data type that share a common name. It is used to handle large amount of data, without the need to declare many individual variables separately. The array elements are stored in a contiguous memory locations. All the array elements must be either of any primitive data types like int, float, char, double etc. or they can be of any user-defined data types like structure and objects.
Index:
- [#1] – Introduction to C++ – Questions
- [#2] – Preparing and Running C++ Program – Questions
- [#3] – C++ Fundamentals – Questions
- [#4] – Data Input and Output – Questions
- [#5] – Control Structures – Questions
- [#6] – Functions – Questions
- [#7] – Arrays and Strings – Questions
- [#8] – Structures and Unions – Questions
- [#9] – Pointers – Questions
- [#10] – Object and Classes – Questions
- [#11] – Constructors and Destructors – Questions
- [#12] – Operator Overloading and Type Conversion – Questions
- [#13] – Inheritance – Questions
- [#14] – Virtual Functions – Questions
- [#15] – C++ Streams and Files – Questions
- [#16] – Templates – Questions
- [#17] – Exception Handling – Questions
Each array element is accessed and manipulated using an array name followed by a unique index or subscript that specify the position within the array. A single subscript is required to access an element of one-dimensional array and two subscripts are required to access an element of two dimensional array. All the arrays whose elements are accessed using two or more subscripts are called multidimensional arrays. It should be kept in mind that each subscript is enclosed in square brackets and it should be expressed as a non-negative integer constant or expression.
Key Points To Remember –
- An array is a collection of logically related variables of identical data type that share a common name. The array elements are stored in a contiguous memory locations. Each array element is accessed and manipulated using an array name followed by a unique index that specifies the position within the array.
- The storage space allocated to an array is equal to the maximum number of elements multiplied by the size of datatype used.
- Arrays are of two types- one dimensional array and multi-dimensional array.
- When an array is passed to a function, it is passed by reference and not by value which means that elements of an array are not copied to the function but the array name is interpreted as the address of its first element. Any changes made are reflected back to original array.
- A two-dimensional array are generally used to store table of values in such a way that information is arranged in ‘rows’ and ‘columns’.
- Two dimensional array can also be initialized with list of values in such a way that elements of first row are initialized first followed by elements of second row and so on.
- A string is a collection of characters terminated by a null character ( ‘\0’) which specifies where the string terminates in memory. A string can be represented as a one-dimensional char type array where each character of the string is stored as an element of an array.
- strlen(), strrev(), strcpy(), strcmp() are some of the commonly used string handling functions. The prototypes of these functions are specified in the header file string.h.
- An array of strings can be represented by a two-dimensional array where the first size specification tells the number of strings and the second size specification tells the number of characters in each string.
Viva Voce Questions –
Here are commonly asked questions with answers:
- Why is it better to use symbolic names for size specification in arrays ?
- It becomes easier to modify a program by simply making changes in the value of symbolic constant.
- What is the benefit of contiguous memory allocation in arrays ?
- By knowing the address of the first elements Of the array.
- Why do array subscripts start from 0 instead of 1 ?
- Starting the subscript from 0 simplifies the job of the compiler for handling arrays. Also, it makes the array subscripting marginally faster.
- Is it possible to specify a character as an array subscript ?
- Yes, because every character has an ASCII value associated with it which is an integer.
- If a and b are two arrays of same type, can the statement a=b, work ?
- No, two arrays can be copied using the statement a=b; It can be done as for (int i=0;i<n;i++)
a[i]=b[i];
- No, two arrays can be copied using the statement a=b; It can be done as for (int i=0;i<n;i++)
- What will be the output of following program segment.
main()
{
…………………………..
int i=1;
int a[4]={1,2,3,4};
f1(a[i], i++);
…………………………..
}
void f1 (int a, int b)
{
cout<<a<<‘\t'<<b;
}- 3 2
This is because during function call, a stack is maintained in which actual arguments are pushed from right to
left f(a[i],i++) ;
First, i++=2 Then a[i=a[2]=3
- 3 2
- Is it essential for strings to end with ‘\0’ ?
- Yes, because this is the only way the compiler comes to know that end of string is encountered.
- What are different ways by which strings can be assigned values ?
- Through initialization. E.g. char sl[]=” Ankur”;
- Using strcpy() function. E.g. strcpy (sl,”Helo”),
- Using input functions. E.g. get() or extraction operator functions
- What is the difference between inputting a string using get() function and extraction operator (>>) function ?
- get() can input multi line string whereas extraction operator cannot input multi word, multi line string.
Other Similar Questions –
- What are arrays ? Why do we need it ?
- How do we define an array ?
- Why is it better to use symbolic names for size specifications ?
- How is one-dimensional array initialized ?
- What values are automatically assigned to those array elements that are not explicitly initialized ?
- How are arrays passed to a function ?
- What are two-dimensional arrays ? How are their elements accessed ?
- State the order in which values are initialized to a two dimensional array .
- Prove that elements of array are passed by reference .
- What are strings ? How are they different from normal character variables ?
- How are strings initialized ? State different methods and advantage of one over the other.
- Can you enter a multi line string ? If yes, how ?
- Can you enter a multi word string ? If yes, how ?
- Discuss the various string library functions available in C++ ?
- What is a string terminator ? What is its purpose ?
- Discuss array of characters ? Where are they used.
- How is character array different from a string ?
- 75 Important Cybersecurity Questions (MCQs with Answers)
- 260 One-Liner Information Security Questions and Answers for Fast Learning
- Top 20 HTML5 Interview Questions with Answers
- 80 Most Important Network Fundamentals Questions With Answers
- 100 Most Important SOC Analyst Interview Questions
- Top 40 Cyber Security Questions and Answers
- Top 10 React JS Interview Theory Questions and Answers
- CISSP – Practice Test Questions – 2024 – Set 20 (53 Questions)
- Part 2: Exploring Deeper into CCNA – Wireless (145 Practice Test Questions)
- Part 1: Mastering CCNA – Wireless (145 Practice Test Questions)