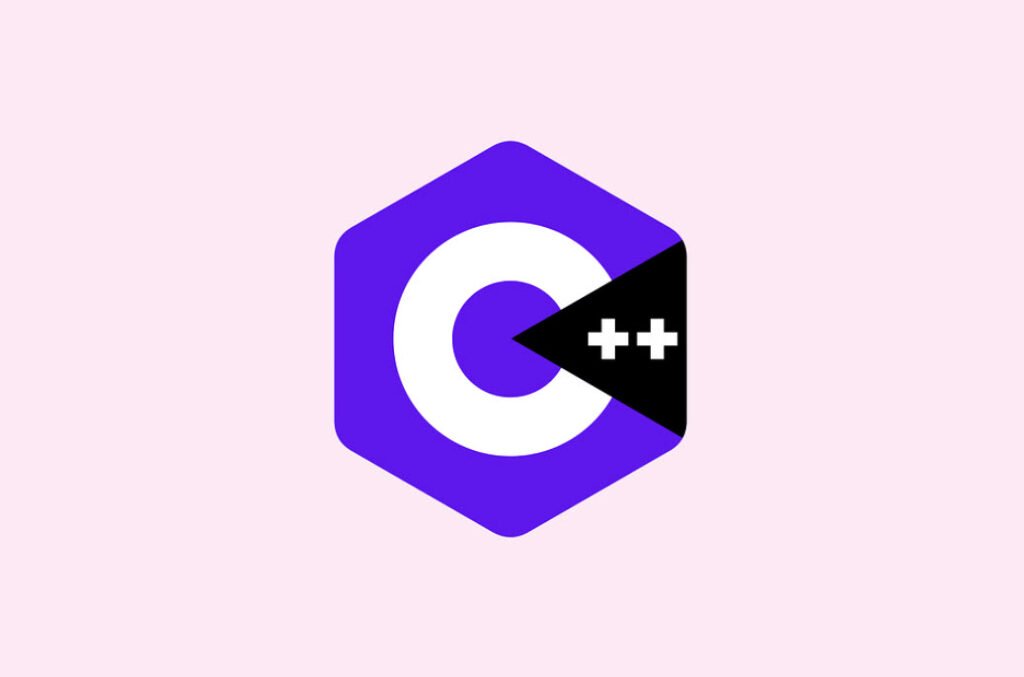
A C++ program is made up of structured sequence of statements. If a program is very large and complex, there are certain group of statements which are repeated again and again at different places in a program. Such programs are very difficult to write, debug, understand, test and maintain. To implement such programs efficiently, we need to break down a large program into smaller sub-programs which can easily be designed, implemented and maintained.
Index:
- [#1] – Introduction to C++ – Questions
- [#2] – Preparing and Running C++ Program – Questions
- [#3] – C++ Fundamentals – Questions
- [#4] – Data Input and Output – Questions
- [#5] – Control Structures – Questions
- [#6] – Functions – Questions
- [#7] – Arrays and Strings – Questions
- [#8] – Structures and Unions – Questions
- [#9] – Pointers – Questions
- [#10] – Object and Classes – Questions
- [#11] – Constructors and Destructors – Questions
- [#12] – Operator Overloading and Type Conversion – Questions
- [#13] – Inheritance – Questions
- [#14] – Virtual Functions – Questions
- [#15] – C++ Streams and Files – Questions
- [#16] – Templates – Questions
- [#17] – Exception Handling – Questions
These sub-programs are called functions. In C++, programmers use functions as a building blocks to construct large programs. The functions are compiled along with the main program to form a complete program. This process of dividing a large program into functions which work independently is known as Modular Programming. Using this technique, we can group together repeated set of instructions which can be placed within a single functions. This can be accessed throughout the program whenever, it is needed.
Key Points To Remember –
- A function is a self contained block of statements that perform a specific task. A function groups a number of repeated statements in a program into a single unit and is identified by a name. Thus, function helps to reduce the size of program by calling and using functions at different places in a program.
- Functions can be categorized as library and user-defined functions. The library functions are predefined and precompiled functions that are designed to perform some specific tasks. The user-defined functions are defined by the user which consists of three components: Function Definition, Function Declaration and Function call.
- Function Definition is that part of function where the actual code of the function is defined, he function call transfer control to the function and function declaration gives the compiler the details about the functions such as the number, a type of arguments and the return type.
- The arguments used in the function call are called the actual arguments. The arguments represented in the called function are called the formal arguments.
- On calling a function, the control is transferred from the calling function to the called function. When the function being called completes its execution, the control is transferred back to the calling function.
- Arguments can be passed to the function(s) by value, by address or by reference. In Pass by value mechanism, subsequent changes to the formal parameter do not affect the actual argument. The function works with the copy of the argument. In pass by address or reference, the function works with the original argument, subsequent changes made to the formal parameter affect the actual parameter.
- Function can return only ne value but it is possible to return more than one value using the concept of references.
- A reference variable must be initialized when it is declared.
- C++ provides the concept of function overloading in which a family of functions share a common name that perform multiple activities. The compiler matches the function call on the basis of number of argument(s) passed and if the number of argument(s) are same then depending upon the type of argument(s) passed to the function.
- When the function is specified as inline, the compiler replaces the function call with the corresponding function code. Normally, the inline mechanism is meant to optimize frequently called small functions.
- An inline function must be defined before it is called.
- C++ provides the facility to use default value(s) for the argument(s) which are specified in function declaration when they are omitted in the function call. These arguments are known as default arguments which are specified left to right.
- Every variable in C++ is associated with a scope and a storage class in addition to its data type. The scope or visibility of a variable is the portion of the program where a variable can be used. The storage class of variable specify how long a variable remains in memory. It determines the lifetime during which memory is associated with a variable.
- There are four types of storage class specification: auto, extern, static, and register.
- auto is the most common storage class specification. Local variables have the auto storage class by default. The scope of an automatic variable is limited to the block or function in which it is defined. Automatic variable(s) are created automatically when the control enters the function (or block) and are destroyed automatically when the control exits the function (or block).
- In multi-file program, the global variable(s) is defined only once in the source file and must be declared using extern keyword in every file that uses it. The extern keyword informs the compiler that the variable thus qualified is actually one of the same name defined in some other file.
- A variable with a static storage class specification has scope limited to the function (or block) in which it is defined and preserves its value for the entire duration of the program.
- Automatic variables in a function are normally stored in the memory, but in order to speed up the access to the variable we store it in registers using register storage class specification.
- Recursion is a process in which a function calls itself repeatedly, until some specified condition is met. It is useful for writing repetitive problems where each action is stated in terms of the previous results.
- A local variable is a variable which is defined inside a function block.
- A global variable is a variable defined above all the functions in a program. A global variable can be defined once.
Viva Voce Questions –
Here are commonly asked questions with answers:
- What does a header file contain ?
- A header file contains the declarations of library functions whose definitions are present in the library file. For example . The header file “math.h” contains declaration of various mathematical library function such as sqrt(), pow () etc.
- Is it necessary to include a function declaration for user-defined function ?
- If the function call precedes the function definition then we must declare the function before using it. However, if the function definition proceeds the function call(s) then there is no need to include function declaration.
- What are the different ways of passing arguments to a function in C++ ?
- Passing by Value
- Passing by Reference
- Passing by Pointers
- What is the output of the following program segment ?
int i=10;
int &j=i;
j=5;
cout<<i;- 5 as ‘j’ is a reference variable of i.
- What is the use of reference variables ?
- Reference variables are used to implement pass by reference parameter passing mechanism in C++
- What is the difference between passing argument by value to that of reference ?
- In case of pass by value, any modification to the formal argument(s) in the called function are not reflected to the actual argument in the calling program whereas in case of pass by reference , modification made in the called function are reflected in the calling program.
- Can we return more than one value from a function ?
- Using return statement, a function can return only one value. However, a function can return more than one value, using pass by Reference or pass by pointer parameter passing techniques.
- If the user simply uses a return statement without any value then what will be the return type of the function ?
- void
- Can you call a function in the cout statement ?
- Yes, you can call a function in the cout statement provided function doesn’t have void return type i.e. function should return something.
- Can two functions with same name and parameter list be distinguished on the basis of their return type ?
- No, Overloaded functions cannot be distinguished on the basis of their return type.
- What is name mangling ?
- It is the process in which name of the functions in the source file is converted to internally different used names. It is usually done in function overloading where the compiler changes the name of all overloaded function definitions so as to implement function overloading.
- Is there any ambiguity while overloading functions ?
- Yes, when two overloaded functions have an argument of same type but differ in technique of parameter passing. If we call these functions like int k=10, the compiler in no way distinguish which version of the overloaded function will be called.
- What is the difference between iterative and recursive programming ?
- An iterative function is a function that uses a loop to repeat an action whereas a recursive function is a function that calls itself repeatedly.
- What is the output of the following program containing macro ?
# include< iostream.h>
#define SQUARE (x) x*x
int main ()
{
int b = square (4.5+3.5);
cout<<b;
getch();
return 0;
}- 45.75
Other Similar Questions –
- What is a function ? Why do we need it ?
- Discuss the advantages of using functions ?
- What are library functions ? Give examples of few library functions ?
- What is a user- defined function ? Why are they needed ?
- What is the difference between a library and an user-defined function ? Why are they needed ?
- What is the purpose of function declaration ? Can we omit it ?
- What do you mean by a function call ? From what parts of a program can a function be called ?
- What are different ways of writing function declarations ?
- What do you mean by actual and formal arguments ? explain the relationship between them ?
- Describe some alternate terms used in place of formal argument(s) and actual argument(s) ?
- When is function declaration required ?
- List out the rules governing the use of return statement ?
- What are various techniques used for passing arguments ?
- What is the difference between Pass by value and Pass by Reference ? Give Examples.
- What are the advantages and disadvantages of Pass by Reference ?
- How can you return more than one value from a function ?
- How will you return a value by reference ?
- List some important points while using functions ?
- What do you mean by function overloading ? When do we need it ?
- In which ways declaration of function overloading differs from normal functions ?
- What are the advantages of function overloading ?
- Give the steps for selection of appropriate overloaded function ?
- Define inline function ? When will you make a function inline ?
- How inline function is different from a preprocessor macro ?
- Does inline function improve the execution speed of program ? Discuss.
- Discuss the advantages and disadvantages of inline functions ?
- Discuss with an example how effect of default arguments can be alternatively achieved by function overloading ?
- When do we need to use default arguments in a function ?
- How is the function containing default arguments evaluated ?
- What are the restrictions on the use of inline functions ?
- Define scope and lifetime of a variable.
- Explain different storage class specifications ?
- Define static variable ? Discuss its lifetime and visibility ?
- Distinguish between the following:
- Actual and Formal arguments
- Global and local variables
- Automatic and static variables
- Global and extern variables
- How a user-defined function differs from other user defined functions ?
- What is the return type of main() ? Why ?
- What are the register variables ? Why do we use it ?
- In a multi-file program, how many times global variables and extern variables needs to be defined and declare ? Why ?
- What is an automatic variable ? When do we use it ?
- Define Recursion ? What type of programs need to solved recursively.
- What are advantages and disadvantages of recursion ?
- 75 Important Cybersecurity Questions (MCQs with Answers)
- 260 One-Liner Information Security Questions and Answers for Fast Learning
- Top 20 HTML5 Interview Questions with Answers
- 80 Most Important Network Fundamentals Questions With Answers
- 100 Most Important SOC Analyst Interview Questions
- Top 40 Cyber Security Questions and Answers
- Top 10 React JS Interview Theory Questions and Answers
- CISSP – Practice Test Questions – 2024 – Set 20 (53 Questions)
- Part 2: Exploring Deeper into CCNA – Wireless (145 Practice Test Questions)
- Part 1: Mastering CCNA – Wireless (145 Practice Test Questions)