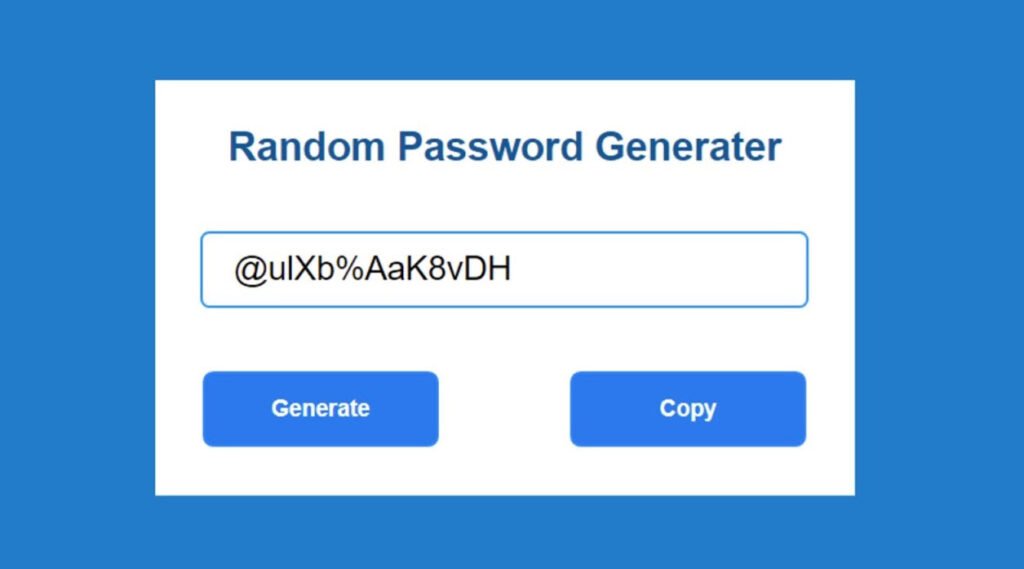
In today’s digital age, password security is more important than ever. With data breaches and cyber-attacks becoming increasingly common, it’s important to ensure that your passwords are strong and secure. One way to achieve this is by using a random password generator. In this article, we’ll show you how to generate random passwords in Python.
Random Password Generator
A random password generator is a tool that generates a password with a random sequence of characters. This ensures that the password is unique and difficult to guess. In Python, we can create a random password generator using the secrets and string modules.
The secrets module provides functions to generate cryptographically strong random numbers. The string module provides string constants that we can use to define the alphabet.
Here’s an example of a simple random password generator in Python:
import secrets
import string
letters = string.ascii_letters
digits = string.digits
special_chars = string.punctuation
selection_list = letters + digits + special_chars
password_len = 10
password = ''
for i in range(password_len):
password+= ''.join(secrets.choice(selection_list))
print(password)
In this example, we first import the secrets and string modules. We then define the letters, digits, and special_chars variables, which contain the characters that can be used to generate the password.
The selection_list variable is a concatenation of letters, digits, and special_chars, which contains all the characters that can be used to generate the password.
The password_len variable is used to set the length of the password. In this example, the length is set to 10.
The password variable is initialized as an empty string. A for loop is used to generate the password. In each iteration of the loop, a random character is chosen from the selection_list using the secrets.choice() function and is added to the password string.
Finally, the generated password is printed to the console.
The above example is a simple random password generator. However, you can customize it to meet your specific needs. Here are some ways to customize the random password generator:
Special characters can make a password stronger, but they can also make it difficult to remember. If you want to limit the use of special characters, you can modify the special_chars variable to include only the special characters you want to use.
To ensure that the password includes at least one digit and one special character, you can modify the for loop to include conditions that check for the presence of digits and special characters. If the password doesn’t contain a digit or a special character, the loop can continue until it does.
To ensure that the password doesn’t contain the same character more than once, you can modify the for loop to check for the presence of each character in the password string before adding it. If the character already exists in the password string, the loop can continue until it finds a different character.
Conclusion
In this article, we showed you how to generate random passwords in Python. By using a random password generator, you can ensure that your passwords are strong and secure. You can customize the random password generator to meet your specific needs by limiting the use of special characters, ensuring the use of at least one digit and one special character, and limiting the use of each character in a password only once.
You may also like:- How To Fix the Crowdstrike/BSOD Issue in Microsoft Windows
- MICROSOFT is Down Worldwide – Read Full Story
- Windows Showing Blue Screen Of Death Error? Here’s How You Can Fix It
- A Guide to SQL Operations: Selecting, Inserting, Updating, Deleting, Grouping, Ordering, Joining, and Using UNION
- Top 10 Most Common Software Vulnerabilities
- Essential Log Types for Effective SIEM Deployment
- How to Fix the VMware Workstation Error: “Unable to open kernel device ‘.\VMCIDev\VMX'”
- Top 3 Process Monitoring Tools for Malware Analysis
- CVE-2024-6387 – Critical OpenSSH Unauthenticated RCE Flaw ‘regreSSHion’ Exposes Millions of Linux Systems
- 22 Most Widely Used Testing Tools