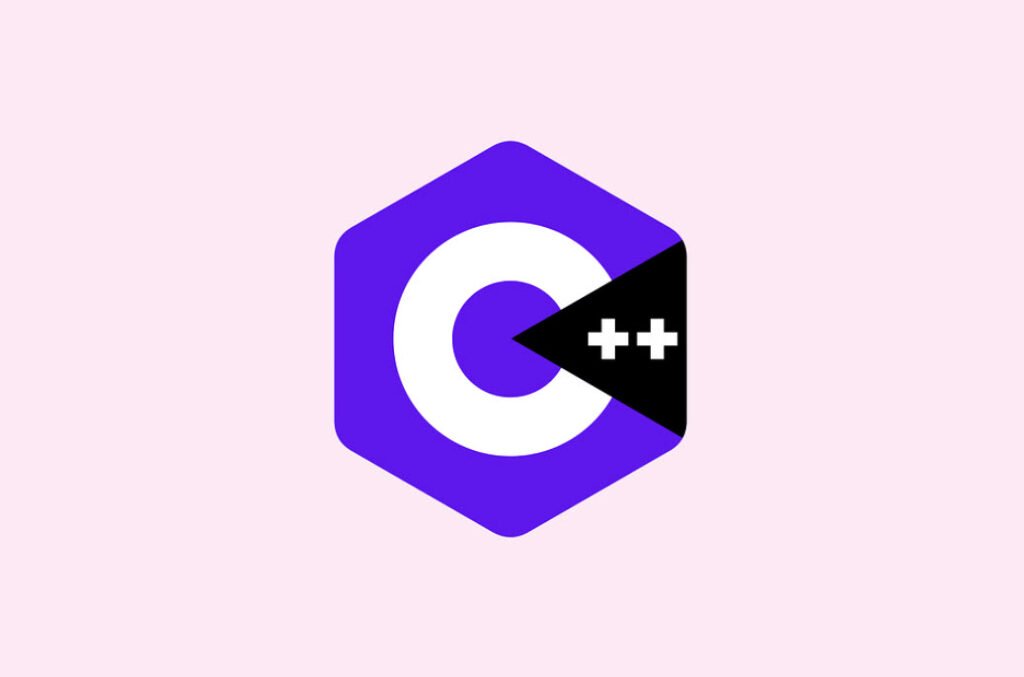
Inheritance is one of the key concept in the Object Oriented Programming language like C++, that enables you to organize classes in a hierarchical form. Just like a child inherits the characteristics of his parents and add certain new characteristics of his own.
Index:
- [#1] – Introduction to C++ – Questions
- [#2] – Preparing and Running C++ Program – Questions
- [#3] – C++ Fundamentals – Questions
- [#4] – Data Input and Output – Questions
- [#5] – Control Structures – Questions
- [#6] – Functions – Questions
- [#7] – Arrays and Strings – Questions
- [#8] – Structures and Unions – Questions
- [#9] – Pointers – Questions
- [#10] – Object and Classes – Questions
- [#11] – Constructors and Destructors – Questions
- [#12] – Operator Overloading and Type Conversion – Questions
- [#13] – Inheritance – Questions
- [#14] – Virtual Functions – Questions
- [#15] – C++ Streams and Files – Questions
- [#16] – Templates – Questions
- [#17] – Exception Handling – Questions
In the similar way in C++, a new class can inherit the data members and member functions of the existing class and can also add members of its own.
This process of creating a new class from an existing class is known as Inheritance.
Key Points To Remember –
- Inheritance is a process of creating a new class from an existing one. The new class is known as a derived class and the existing class as a base class. All the data members and member functions in the base class are automatically included in the derived class along with its own members.
- Reusability is the key advantage of inheritance which results in saving time and increases the reliability of the program.
- A base class can be inherited using public, private or protected access specifier.
- Inheritance only works in one direction. The derived class has a very clearly defined relationship to base class but the base class does not know anything about the derived class. This means that objects of the base cannot access even the public members of the derived class.
- In public inheritance, the public members of the base class becomes public members of the derived class and protected members of the base class becomes protected member of the derived class. However, the private member of the base class remain private in the base class.
- In private inheritance, all the public and protected members of the base class becomes private members of the derived class and hence are accessible only in the derived class and not outside the base and derived classes. However, the private members of the base class remain private in the base class.
- In protected inheritance, all the public and protected members of the base becomes protected members of the derived class. The private members of the base class remain private in the base class and are inaccessible directly to its derived class and other parts of the program.
- The main difference between public and private inheritance is that in case of public inheritance, the public members of base and derived classes can be accessed directly by the object(s) of the derived class, whereas in case of private inheritance only the public members of the derived class can be accessed directly by the objects of the derived class.
- The main difference between protected and private inheritance is that in the former case continued access to the base class members further down the descendent derived classes which you might have derived from an existing derived class is possible. This is not so in case of private inheritance.
- When the member function with the same exists in both the base class as well as in the derived class, then the object of the derived class will invoke the member function defined in the derived class with the same name and parameters as that defined in the base class. This is known as overriding member function.
- In single inheritance, a class is derived from only one base class.
- In multilevel, a class can be derived from an existing derived class.
- In hierarchical inheritance, a single base class can be used to derive multiple classes which in turn can act as a base class for lower level multiple derived classes and so on.
- In multiple inheritance, a class can be derived from two or more unrelated base classes.
- Combining two or more forms of inheritance to design a program is known as hybrid inheritance.
- When a class is inherited as virtual, the compiler takes essential caution to avoid duplicate copies of its members. Thus multiple classes derived from it share only one copy of its member.
- When a base class and derived class both have constructors then the constructors are executed in the order of derivation i.e. execution of construction takes place from base class to derived class.
- In multiple inheritance, the base class constructors are executed in the same order as specified in the derived class’s derivation list.
- Destructors are executed in the reverse order as compared to constructors i.e. from derived class to base class.
- When a class contains objects of other classes as its data members then this known as object composition.
Viva Voce Questions –
Here are commonly asked questions with answers:
- How can you access the public members of the base through an object of derived class from main() ?
- It is possible only if the class is derived publicly. This is because with public inheritance, public members of the base class become public of the derived class.
- What is the difference between public and private inheritance ?
- The difference is that in case of public inheritance, the public member of the base and derived classes can be accessed directly by the objects of the derived class whereas in case of private inheritance only the public members of the derived class can be accessed directly by the objects of the derived class.
- What is the difference between protected and private inheritance ?
- In the protected inheritance, continued access to the base class members further down the descendent derived classes which you might have derived from an existing derived class is possible. This is not so in the case of private inheritance.
- When should base class data members be made protected rather than private or public ?
- If we went that certain members of the base class to be accessed directly in the base class as well as its derived class, but not from outside the base or derived classes (i.e. main() then such members of the base class should be declared as protected.
- What is the size of the object of derived class ?
- This size of the object of the derived class is equal to the sum of sizes of data members of the base class and derived class.
- If a class is derived publicly from a base class and both classes contain a same named public member function f1() then can an object of the derived class the base’s same named member function from main() ?
- Yes, using the scope resolution operator as
derv_obj.base_class_name::();
- Yes, using the scope resolution operator as
- If both base class and derive class contains same named public member function f1(), then which version of f1() will be invoked when called through an object of the derived class ?
- The object of derive class invokes the fI() version defined in the derived class as compiler always first searches the same named member function in the derived class and then in the base class.
- Is there any kind of relationship exists between classes that form the Inheritance ?
- Yes there is kind of relationship exist between a derive class and a base class.
- What is order of execution of constructors and destructors in inheritance ?
- Constructors are executed in the order of derivation in inheritance whereas destructors are executed in the reserve order of their creation.
- What kind of relationship exists between classes involved in composition ?
- has a relationship
Other Similar Questions –
- What do you mean by Inheritance ? Explain the need of inheritance with example.
- Describe base class and derived class ?
- How would you declare a derived class ?
- What is the significance of access specifiers for deriving classes ?
- What do you mean by public inheritance ? Explain using an example.
- Explain private inheritance using an example.
- Explain the concept of protected inheritance using example ?
- Can a base class access members of a derived class ? If no, give reasons .
- What are the different types of access specifiers used for deriving classes supported by C++ ?
- Compare public and private Inheritance using an example.
- What is the access control mechanism of the members of the base class if the base class is inherited as
- public
- private
- protected
- Can we access the private members of the base by objects of derived class ?
- What are the difference between inheriting a class with public and protected access specifiers ?
- Why do you declare members of the base class as protected ?
- Can private members of base class be accessible in derived class ?
- Explain how base class member functions can be invoked in a derived class if a derived class also has a member function with a same name .
- What are the different forms of inheritance ? Explain them with examples.
- What is the difference between multilevel and multiple Inheritance ?
- Does multiple inheritance leads to ambiguity ? how can it be removed ?
- What is Hybrid Inheritance ?
- What is virtual base class ? Why are they needed ?
- Using an example, explain the concept of virtual Inheritance .
- In what order the class constructors are called when a derived class object is created ?
- How are the constructors executed in
- Multiple Inheritance
- Multilevel Inheritance
- How are the arguments passed to the base class constructor in multiple inheritance ?
- Explain the calling sequence of destructors in inheritance.
- What is composition ? How does it different from inheritance ?
- Describe the process of creating an object of a class that contain objects of other classes as data members.
- What are the advantages and disadvantages of inheritance ?
- State with reasons whether the following statements are TRUE or FALSE
- Only derived class can nave constructors.
- Constructors are executed in the order starting from the top base class to derived classes in multilevel inheritance.
- Destructors are executed in the reverse order of constructors.
- When a derived class is instantiated only the derived class constructors are invoked.
- If a base class has parameterized constructors only then it must be explicitly invoked from a derived class.
- No parameter constructor of the base class is invoked when a derived class in instantiated.
- 75 Important Cybersecurity Questions (MCQs with Answers)
- 260 One-Liner Information Security Questions and Answers for Fast Learning
- Top 20 HTML5 Interview Questions with Answers
- 80 Most Important Network Fundamentals Questions With Answers
- 100 Most Important SOC Analyst Interview Questions
- Top 40 Cyber Security Questions and Answers
- Top 10 React JS Interview Theory Questions and Answers
- CISSP – Practice Test Questions – 2024 – Set 20 (53 Questions)
- Part 2: Exploring Deeper into CCNA – Wireless (145 Practice Test Questions)
- Part 1: Mastering CCNA – Wireless (145 Practice Test Questions)