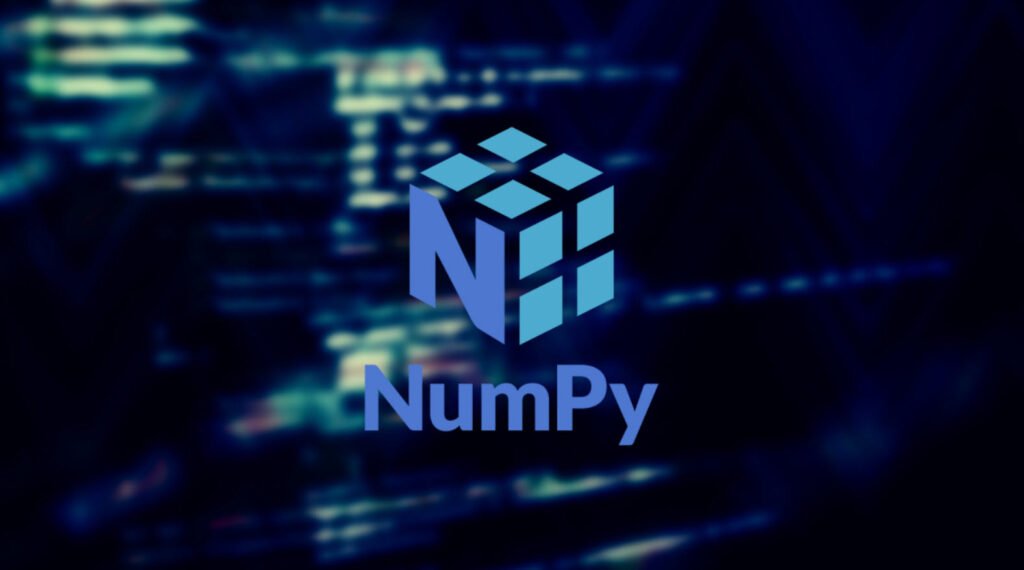
NumPy, short for Numerical Python, is a powerful array processing library in Python. It provides a high-performance multidimensional array object and a set of tools for working with these arrays. NumPy is a cornerstone of scientific computing in Python, offering advanced capabilities for numerical operations and data manipulation.
In this article, we will explore the fundamentals of NumPy and delve into a set of interview questions to deepen your understanding.
1. What exactly is NumPy?
NumPy is a Python-based array processing library that includes a high-performance multidimensional array object and utilities for manipulating these arrays. It is a fundamental module for scientific computing in Python, providing an N-dimensional array object with powerful and sophisticated broadcasting functions.
2. What is the purpose of NumPy in Python?
NumPy is designed for scientific computing in Python. It introduces a multidimensional array called `ndarray` (NumPy Array) that holds values of the same data type. This array allows efficient indexing and supports various mathematical functions for operations on arrays, making it a key tool for tasks in numerical computing.
3. What does Python’s NumPy stand for?
NumPy (pronounced /NUM-py/ or /NUM-pee/) is a Python library that adds support for large, multi-dimensional arrays and matrices. It also provides a wide range of high-level mathematical functions to operate on these arrays.
4. Where does NumPy come into play?
NumPy is a free, open-source Python library dedicated to numerical computations. It introduces multi-dimensional array and matrix data structures and enables the execution of various operations on arrays, including trigonometric, statistical, and algebraic algorithms. NumPy serves as an extension of Numeric and Numarray.
5. Installation of NumPy into Windows?
Installation Steps:
1. Install Python on your Windows 10/8/7 computer by downloading the Python executable binaries from the official Python website.
2. Install Python using the Python executable installer.
3. Download and install `pip` for Windows 10/8/7.
4. Open the terminal and type the following command to install NumPy:
pip install numpy
This process ensures that NumPy is successfully installed on your Windows machine.
6. What is the best way to import NumPy into Python?
The conventional way to import NumPy into Python is by using the alias `np`:
import numpy as np
This alias is widely adopted in the Python community and simplifies the use of NumPy functions and classes.
7. How can I make a one-dimensional (1D) array?
To create a 1D array in NumPy, you can use the following example:
num = np.array([1, 2, 3])
print("1D array:", num)
8. How can I make a two-dimensional (2D) array?
To create a 2D array in NumPy, you can use the following example:
num2 = np.array([[1, 2, 3], [4, 5, 6]])
print("\n2D array:", num2)
9. How do I make a 3D or ND array?
To create a 3D array in NumPy, you can use the following example:
num3 = np.array([[[1, 2, 3], [4, 5, 6], [7, 8, 9]]])
print("\n3D array:", num3)
10. What is the best way to use the shape in a 1D array?
The shape of a 1D array can be accessed as follows:
if not defined, print("Shape of 1D:", num.shape)
11. What is the best way to use shape in a 2D array?
The shape of a 2D array can be accessed as follows:
if not added, print("Shape of 2D:", num2.shape)
12. What is the best way to use shape in 3D or ND Array?
The shape of a 3D array can be accessed as follows:
if not added, print("Shape of 3D:", num3.shape)
13. What is the best way to identify the data type of a NumPy array?
The data type of a NumPy array can be identified as follows:
print("\nData type of Num 1:", num.dtype)
print("Data type of Num 2:", num2.dtype)
print("Data type of Num 3:", num3.dtype)
14. Can you print 5 zeros?
To create an array with 5 zeros, you can use the `np.zeros` function:
arr = np.zeros(5)
print("Single array:", arr)
15. Print zeros in a two-row, three-column format?
To create a 2D array with zeros in a two-row, three-column format:
arr2 = np.zeros((2, 3))
print("\nPrint 2 rows and 3 cols:", arr2)
16. Is it possible to utilize `eye()` diagonal values?
Yes, you can create an array with diagonal values using the `np.eye` function:
arr3 = np.eye(4)
print("\nDiagonal values:", arr3)
17. Is it possible to utilize `diag()` to create a square matrix?
Yes, you can use the `np.diag` function to create a square matrix:
arr3 = np.diag([1, 2, 3, 4])
print("\nSquare matrix:", arr3)
18. Printing range: Show 4 integers random numbers between 1 and 15.
To generate 4 random integers between 1 and 15:
rand_arr = np.random.randint(1, 15, 4)
print("\nRandom numbers from 1 to 15:", rand_arr)
19. Print a range of 1 to 100 and show four integers at random.
To generate 4 random integers between 1 and 100:
rand_arr3 = np.random.randint(1, 100, 20)
print("\nRandom numbers from 1 to 100:", rand_arr3)
20. Print range between random numbers: 2 rows and three columns, select integer’s random numbers.
To generate a 2×3 array of random integers:
rand_arr2 = np.random.randint([2, 3])
print("\nRandom numbers 2 rows and 3 cols:", rand_arr2)
21. What is an example of the `seed()` function? What is the best way to utilize it? What is the purpose of `seed()`?
The `seed()` function is used to seed the random number generator, ensuring reproducibility. Here’s an example:
np.random.seed(123)
rand_arr4 = np.random.randint(1, 100, 20)
print("\nseed() showing the same numbers only:", rand_arr4)
22. What is one-dimensional indexing?
One-dimensional indexing in NumPy allows accessing elements at specific positions. For example:
num = np.array([5, 15, 25, 35])
print("My array:", num)
23. Print the first, last, second, and third positions.
To print specific positions in a NumPy array:
num = np.array([5, 15, 25, 35])
print("\nFirst position:", num[0]) # 5
print("Third position:", num[2]) # 25
24. How do you find the final integer in a NumPy array?
To find the last integer in a NumPy array:
num = np.array([5, 15, 25, 35])
print("\nFourth position:", num[3])
25. How can we prove it pragmatically if we don’t know the last position?
Using negative indexing to access the last position:
num = np.array([5, 15, 25, 35])
print("\nLast indexing done by -1 position:", num[-1])
These interview questions cover fundamental concepts and practical aspects of using NumPy in Python. Mastering NumPy is crucial for anyone involved in scientific computing, machine learning, or data analysis using Python.
You may also like:- 80 Most Important Network Fundamentals Questions With Answers
- 100 Most Important SOC Analyst Interview Questions
- Top 40 Cyber Security Questions and Answers
- Top 10 React JS Interview Theory Questions and Answers
- CISSP – Practice Test Questions – 2024 – Set 20 (53 Questions)
- Part 2: Exploring Deeper into CCNA – Wireless (145 Practice Test Questions)
- Part 1: Mastering CCNA – Wireless (145 Practice Test Questions)
- [1z0-1085-20] Oracle Cloud Infrastructure Foundations 2020 Associate MCQ Questions – Part 3
- [1z0-1085-20] Oracle Cloud Infrastructure Foundations 2020 Associate MCQ Questions – Part 2
- [1z0-1085-20] Oracle Cloud Infrastructure Foundations 2020 Associate MCQ Questions – Part 1