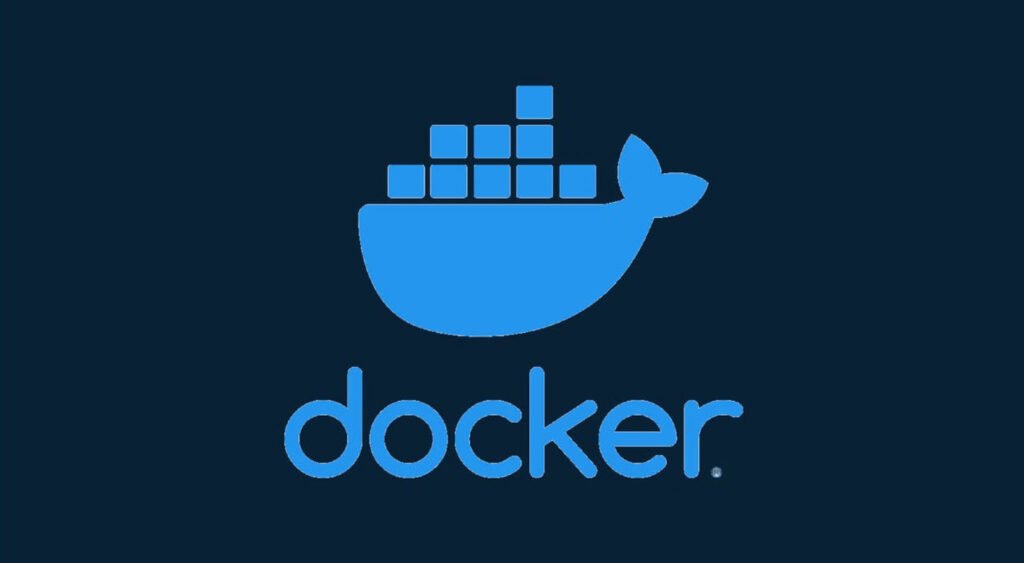
Docker has revolutionized the way we develop, deploy, and run applications by providing a consistent and efficient platform for containerization. With Docker, you can package your application and its dependencies into a container, ensuring that it runs consistently across different environments.
To help you navigate this powerful tool, we have compiled a comprehensive guide to essential Docker commands.
Building and Running Containers
1. `docker build -t friendlyname .`
This command is used to build a Docker image from the Dockerfile present in the current directory (`.`). The `-t` flag allows you to tag the image with a friendly name (`friendlyname` in this example).
2. `docker run -p 4000:80 friendlyname`
This command starts a container from the specified image (`friendlyname`) and maps port 4000 on the host to port 80 in the container. This is useful for accessing web applications running inside the container.
3. `docker run -d -p 4000:80 friendlyname`
Similar to the previous command, but with the addition of the `-d` flag, which runs the container in detached mode. The container will run in the background, and you’ll get the command prompt immediately.
4. `docker exec -it [container-id] bash`
This command allows you to enter a running container (`[container-id]`) and access its shell interactively (`bash`). It is particularly helpful for debugging and executing commands inside the container.
5. `docker ps`
To list all the running containers, this command comes in handy. It displays information such as the container ID, names, and ports being used.
6. `docker stop <hash>`
To gracefully stop a specific container, use this command, replacing `<hash>` with the container ID or its name.
7. `docker ps -a`
If you want to see a list of all containers, including the ones not running, this command is useful.
8. `docker kill <hash>`
This command forcefully shuts down the specified container by stopping its processes.
9. `docker rm <hash>`
To remove a stopped container from your system, use this command. It deletes the specified container (`<hash>` can be the container ID or name).
10. `docker rm -f <hash>`
This is similar to the previous command but forcefully removes a running container.
11. `docker rm $(docker ps -a -q)`
To clean up your system and remove all containers (both running and stopped) at once, use this command.
Managing Docker Images
12. `docker images -a`
This command shows a list of all images present on your system, including intermediate and dangling images.
13. `docker rmi <imagename>`
To remove a specific image from your system, use this command, replacing `<imagename>` with the image’s name or ID.
14. `docker rmi $(docker images -q)`
This command removes all images from your system, including dangling images (untagged or unreferenced images).
Logging and Authentication
15. `docker logs <container-id> -f`
This command allows you to view the logs of a specific container (`<container-id>`) in real-time. The `-f` flag ensures that the log output keeps streaming to your terminal.
16. `docker login`
Before pushing your Docker images to a registry, you need to log in to your Docker account using this command.
17. `docker tag <image> username/repository:tag`
To tag an image with the username, repository, and a version (`tag`), use this command. It is necessary for uploading images to a Docker registry.
18. `docker push username/repository:tag`
This command uploads the tagged image to a Docker registry, making it available for others to use.
19. `docker run username/repository:tag`
To run an image from a Docker registry, use this command, replacing `username/repository:tag` with the appropriate image information.
Docker Compose
Docker Compose is a tool for defining and running multi-container Docker applications using a YAML file. It simplifies managing complex multi-container setups.
20. `docker-compose up`
This command creates and starts containers defined in the `docker-compose.yml` file.
21. `docker-compose up -d`
Similar to the previous command, but it runs containers in detached mode.
22. `docker-compose down`
To stop and remove containers, networks, images, and volumes created by Docker Compose, use this command.
23. `docker-compose logs`
To view the output from containers defined in the `docker-compose.yml` file, this command comes in handy.
24. `docker-compose restart`
This command restarts all the services defined in the `docker-compose.yml` file.
25. `docker-compose pull`
To pull all the images required for services defined in the `docker-compose.yml` file, use this command.
26. `docker-compose build`
This command builds all the images required for services defined in the `docker-compose.yml` file.
27. `docker-compose config`
To validate and view the Compose file, use this command.
28. `docker-compose scale <service_name>=<replica>`
This command scales a specific service (`<service_name>`) to have the desired number of replicas (`<replica>`).
29. `docker-compose top`
To display the running processes of services defined in the `docker-compose.yml` file, use this command.
30. `docker-compose run –rm -p 2022:22 web bash`
This command starts the `web` service defined in the `docker-compose.yml` file and runs the `bash` command in it, with the `–rm` flag to remove the container after it exits.
Docker Services
Docker Services are used when working with Docker in a swarm mode, enabling you to manage a cluster of Docker nodes.
31. `docker service create <options> <image> <command>`
This command creates a new service with specified options, using the provided image and command.
32. `docker service inspect –pretty <service_name>`
To display detailed information about a service, use this command.
33. `docker service ls`
This command lists all the services running in the Docker swarm.
34. `docker service ps`
To list the tasks associated with the services in the Docker swarm, use this command.
35. `docker service scale <service_name>=<replica>`
This command scales a specific service (`<service_name>`) in the Docker swarm to the desired number of replicas (`<replica>`).
36. `docker service update <options> <service_name>`
To update the options of a specific service in the Docker swarm, use this command.
Docker Stack
Docker Stack is used to deploy a complete application stack to a Docker swarm.
37. `docker stack ls`
This command lists all the running applications (stacks) on the Docker swarm.
38. `docker stack deploy -c <composefile> <appname>`
To deploy a Docker Compose file as a stack in the Docker swarm, use this command. The `<composefile>` parameter specifies the path to the Compose file, and `<appname>` is the name you want to assign to the stack.
39. `docker stack services <appname>`
This command lists all the services associated with a specific stack (`<appname>`) in the Docker swarm.
40. `docker stack ps <appname>`
To list the running containers associated with a specific stack (`<appname>`) in the Docker swarm, use this command.
41. `docker stack rm <appname>`
To tear down an entire application stack in the Docker swarm, use this command. It stops and removes all the services, containers, and networks associated with the stack.
Docker Machine
Docker Machine is a tool for provisioning and managing multiple Docker hosts.
42. `docker-machine create –driver virtualbox myvm1`
This command creates a new virtual machine (VM) with Docker installed using the VirtualBox driver.
43. `docker-machine create -d hyperv –hyperv-virtual-switch “myswitch” myvm1`
For Windows 10 users, this command creates a VM using Hyper-V as the driver and connects it to a specific virtual switch named “myswitch.”
44. `docker-machine env myvm1`
To view basic information about your Docker node (`myvm1`), use this command. It provides the necessary environment variables to interact with the Docker daemon on that node.
45. `docker-machine ssh myvm1 “docker node ls”`
This command allows you to SSH into the VM (`myvm1`) and run Docker commands directly on the Docker node.
46. `docker-machine ssh myvm1 “docker node inspect <node ID>”`
To inspect a specific Docker node (`<node ID>`) from the VM, use this command.
47. `docker-machine ssh myvm1 “docker swarm join-token -q worker”`
This command is used to get the join token for worker nodes, enabling them to join the Docker swarm.
48. `docker-machine ssh myvm1`
To open an SSH session with the VM (`myvm1`), use this command. Type “exit” to end the session.
49. `docker-machine ssh myvm2 “docker swarm leave”`
This command makes the worker node (`myvm2`) leave the Docker swarm.
50. `docker-machine ssh myvm1 “docker swarm leave -f”`
To forcefully make the master node (`myvm1`) leave the swarm, use this command. It will also kill the swarm.
51. `docker-machine start myvm1`
This command starts a VM (`myvm1`) that is currently not running.
52. `docker-machine stop $(docker-machine ls -q)`
To stop all running VMs, use this command. It selects all the VMs returned by `docker-machine ls -q` and stops them.
53. `docker-machine rm $(docker-machine ls -q)`
To delete all VMs and their disk images, use this command. It selects all the VMs returned by `docker-machine ls -q` and removes them.
54. `docker-machine scp docker-compose.yml myvm1:~`
This command copies the `docker-compose.yml` file to the home directory (`~`) of the VM (`myvm1`).
55. `docker-machine ssh myvm1 “docker stack deploy -c <file> <app>”`
To deploy a Docker Compose file as a stack on a specific node (`myvm1`) in the Docker swarm, use this command.
Docker offers a vast array of commands to manage containers, images, stacks, and services efficiently. Learning these commands can significantly boost your productivity and make the process of working with Docker smoother and more enjoyable. Whether you are a developer, system administrator, or DevOps engineer, mastering these commands will empower you to harness the full potential of Docker and containerization for your projects and applications.
Happy Dockering!
You may also like:- A Comprehensive Guide to File System Commands in Linux
- Essential File Compression Commands in Linux
- Secure Shell (SSH) Protocol – A Comprehensive Guide
- Monitoring Active Connections in Kali Linux Using Netstat
- Manage Time and Date in Linux with timedatectl
- How to Add a User to Sudoers on Ubuntu
- 25 Popular Linux IP Command Examples
- Top 11 Nmap Commands for Remote Host Scanning
- 9 Useful w Command Examples in Linux
- 25 Useful Linux SS Command Examples to Monitor Network Connections