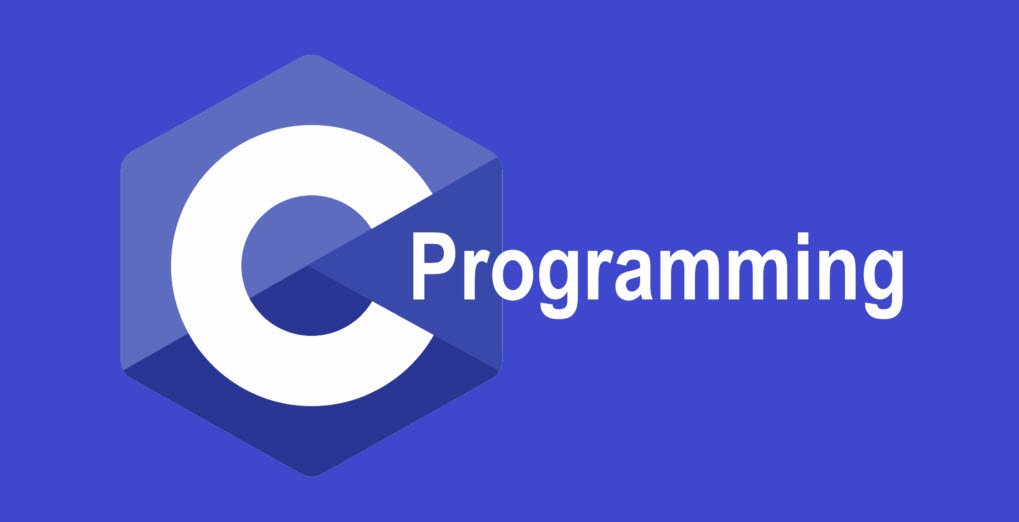
C is a mid-level and procedural programming language. The Procedural programming language is also known as the structured programming language is a technique in which large programs are broken down into smaller modules, and each module uses structured code. This technique minimizes error and misinterpretation. More details.
C is a low-level programming language that can be directly interfaced with the processor. It offers minimal abstraction and maximal control, making it an attractive option for developers who want to write efficient code.
This article is mainly focused on the most asked and the latest updated interview questions that are appearing in most of the job interviews.
A list of 80+ top frequently asked C programming interview questions and answers are given below.
1Q. Who developed C Programming Language ?
Dennis Ritchie at AT & T’s Bell Laboratories in 1972.
2Q. What is the meaning of constant in C ?
Constant is an entity whose value remains fixed.
3Q. What is a variable in C ?
Variable is an entity that acts as storage place for a value.
For ex. int a = 20; here, a is variable
4Q. What are Keywords ?
Keywords are the special words that provide specific meaning to the language compiler. There are 32 keywords in C language.
5Q. Can we use a keyword as a variable-name ?
No.
6Q. Explain comments in C ?
Comments are the user defined statements that ensures the better readability and makes code more understandable. Comments are ignored by compiler. Comments can be single line or even multi line.
7Q. Can we do nesting of comments in C ?
No, not allowed.
8Q. What are escape sequence ?
Escape sequences are basically control characters used for formatting the output. These are combinations of a backslash and a character in lowercase. We use ‘\n‘ for newline.
9Q. Can a program be compiled without main() ?
Yes, but it will not run.
10Q. Is main a keyword ?
No.
11Q. Can you differentiate between variable declaration & variable definition ?
- Variable declaration : assigning data type to a variable. For ex. : int x;
- Variable definition : assigning a value to a variable. For ex. : int x = 10;
12Q. What is modular programming ?
A. It is an approach in which we divide a program into subprograms where each subprogram has its own set of statements for a task.
13Q. What are Tokens ?
Token refers to the smallest lexical unit of a program. It may be a identifier,keyword, constant etc.
14Q. Explain Identifiers ?
Identifiers refers to the legal name given to a variable or a function. It cannot be a keyword and cannot start with a number.
15Q. What could be maximum length of an identifier in C ?
32 characters.
16Q. What do you know by scope of a variable ?
Scope defines the area over which the variable’s declaration has an effect.
17Q. What are Data Segments ?
Data Segments contains initialized data.
18Q. Why header files are enclosed inside < > while some are enclosed under ” ” ?
If any file is enclosed under < >, then compiler searches it in built-in path.
If any file is enclosed under ” “, then given file is user defined and hence current working space is checked.
19Q. How an integer with negative sign is stored in memory ?
Let us assume that we have to store x = -5 to the memory, so :
1. Find 1’s complement of that number.
2. Add 1 to it.
20Q. What is a Pointer ?
Pointer is a special type of variable that holds the memory address of another variable. It is denoted as * (var_name).
21Q. What is a NULL pointer ?
Null pointer is a type of pointer that points to nothing.
For ex : char *p = NULL;
22Q. What are Dangling pointers ?
A pointer pointing to a memory address that has been deleted or not available. To solve this, allocate it to NULL.
23Q. When we use void pointers ?
When we don’t the know the type of value that has to be stored in a variable, in that case we go for void pointers.
24Q. Explain printf, sprintf, fprintf ?
printf : standard output stream (stdout)
fprintf : prints content in file
sprintf : prints formatted output onto char array.
25Q. t++ or t=t+1, which one is recommended more ?
t++, as it is a single machine instruction ( INS ), hence it is faster.
26Q. What is the purpose of using typedef ?
It is used to define alternative names to existing datatype.
Example : typedef long long int ll;
27Q. What is a function ?
Function represents the block of statements that perform a specific task.
Syntax : return-type function-name( parameters )
28Q. What are actual & formal parameters ?
Actual parameters : Parameters sent at calling end.
Formal Parameters : Parameters at the recieving end.
29Q. When we mention the prototype of a function, are we defining it or declaring it ?
We are declaring it.
30Q. What is size_t ?
It is the result of sizeof() operator. sizeof() operator returns the size of a variable that belongs to a particular type.
31Q. What will happen if we include same header file twice ?
Error.
32Q. Explain Recursion ?
Recursion is the technique in which a function is called by itself again and again.
33Q. Which data structure is used during the process of recursion ?
Stack for proper function scheduling.
34Q. What is Preprocessor ?
It processes the source code, before compilation, by adding external libraries, unzipping macros etc.
35Q. What are macros ?
Macro is a name given to a block of C statements as a pre-processor directive. Being a pre-processor, the block of code is communicated to the compiler before entering into the actual coding (main () function). A macro is defined with the preprocessor directive, #define.
36Q. What is the basic difference between a function and a macro ?
The basic difference is that function is compiled and macro is preprocessed.
37Q. How to define multiline macros ?
Using ‘ \ ‘ (backslash).
38Q. Function vs Macros – which is better ?
It depends. Usually macros make the program run faster but increases the program size, whereas function makes program compact and smaller.
39Q. What is an Array ?
Array is a linear data structure having collection of homogenous data items.
40Q. What is the base address of an array ?
Base address represents the address of first element of an array.
41Q. What will be the equivalent pointer expression for following : a[i][j] ?
*(*(a+i)+j)
42Q. Highlight the difference between Structures and Union ?
- Structure is a user defined data type containing heterogenous type of data. Union is also a user defined data type containing heterogenous type of data.
- Structure is achieved using struct keyword.Union is achieved using union keyword.
- Memory size of structure is equal to the sum of memory taken by its data members individually. Memory size of union is equal to the size of largest data member.
43Q. What is Self Referential structure ?
A Structure is recognized as self-referential structure, if it has one or more pointers pointing to itself.
44Q. What are Command Line Arguments ?
The arguments which we pass to the main() function during the execution of a program are command line arguments.
Syntax : int main( int argc, char *argv[])
argc = argument count
argv = argument value
45Q. What is a String ?
String represents a sequence of characters, ending with ‘\0’ (null).
46Q. Explain the utility of stricmp() ?
It compares two strings by ignoring their case.
47Q. Explain goto statements ?
It is used for unconditional branching within a program.
48Q. What is FILE in FILE *fp; ?
FILE is a structure in stdio.h, where as fp is a file pointer.
49Q. What is a NULL statement ?
It is a non-executable statement.
50Q. What are static function ?
Functions having static as keyword are static function. We make static function, when we want to restrict access to function. By default, all functions are static.
51Q. Explain the utility of ellipses operator ?
It is used to recieve the variable number of arguments for a function. It is denoted as ‘ … ‘ .
Syntax : void func(int k, …)
52Q. What is Enumeration ?
It is the user defined data type. It assigns names to integer constants. It is achieved through keyword enum. Enum names can have same values. But no two enums can have same values. Enums are automatically assigned to 0.
53Q. Explain the use of fseek() in File Handling ?
It is used for moving the file pointer internally.
54Q. What is dynamic memory allocation ?
It is the process in which memory is allocated during runtime or execution of a program.
55Q. How we achieve dynamic memory allocation in C ?
We achieve dynamic memory allocation using functions like malloc(), calloc(), free(), realloc().
56Q. Explain malloc() ?
malloc() is one of the functions used for dynamic memory allocation. It takes up single arguments, which denotes the number of bytes to be allocated. malloc() is faster than calloc().
Syntax : int *p = (int *)malloc(sizeof(int))
57Q. Explain calloc() ?
calloc() is one of the functions used for contiguous dynamic memory allocation. It takes up two arguments, in which first argument denotes the number of bytes to be allocated, and second argument denotes size of each block. It initializes allocated memory by 0.
Syntax : int *p = (int *)calloc(10,sizeof(int))
58Q. malloc() vs calloc() – which is faster ?
malloc() is faster than calloc(), because of one extra step of initializing the allocated memory by 0, in case of calloc().
59Q. Explain the functionality of realloc() ?
It resizes the memory block that was previously allocated with a call to malloc or calloc. It takes two arguments : first parameter is a pointer to a memory block that was previously allocated with malloc, or calloc, second parameter represents the size of new memory block.
60Q. When we use free() ?
It is use to free the memory block that had been allocated dynamically.
61Q. What are wild pointers ?
Wild pointers are uninitialized pointers.
62Q. Are null pointers and wild pointers same ?
No.
63Q. What are Bit Fields ?
Bit Fields are used to save space in structures having several binary flags or other small fields.
64Q. Can we define an array of bit field ?
No.
65Q. What are the valid operations that can be performed on pointers ?
1. Comparison
2. Addition
3. Subtraction
66Q. Explain the concept of #undef ?
#undef is used to undefine the existing macro.
67Q. What you can tell me about far pointers and near pointers ?
- Near pointers can access upto 216 memory space.
- Far pointers can access upto 232 memory space.
68Q. Can we have nested comments in C ?
No.
69Q. Can we left main() function empty ?
Yes.
70Q. Name some entry and exit control loops ?
Entry control loops : for, while
Exit control loops : do
71Q. What if we insert ‘ ; ‘ ( semi colon ) after a header file ?
Program will compile and run with a warning.
72Q. Why preprocessors does not have ‘ ; ‘ at the last ?
Semicolon is needed by compiler, for compiling a program. As the name itself suggests that preprocessor directives are processed before compilation of a program, hence it is not needed.
73Q. Explain the various phases in the process of recursion ?
Recursion has two phases :
- Winding Phase : This phase runs until desired condition is fulfilled.
- Unwinding Phase : Once winding phase gets over, control is transferred back to original call, this is unwinding phase.
74Q. What is the basic difference between getch() & getche() ?
- getch() : reads single character from keyboard.
- getche() : reads single character but output is displayed on screen.
75Q. Can we call main() function recursively ?
Yes.
76Q. Is main() function a user defined function ?
Yes.
77Q. When we use ‘ %p ‘ inside printf() ?
For printing address location of a variable.
78Q. What is __STDC__ ?
It is a predefined macro which is used to determine whether your compiler is ANSI STANDARD or not.
79Q. What are the different types of streams in C File Handling ?
Basically, there are two types of file handling streams : Text and Binary.
80Q. Can a function in C return more than 1 values ?
No, not possible.
81Q. Name some predefined macros in C ?
__LINE__ , __STDC__ , __FILE__ , __DATE__ , __TIME__ .
82Q. What is #include<pragma.h> ?
The #pragma directive is the method specified by the C standard for providing additional information to the compiler, beyond what is conveyed in the language itself.
83Q. How to compile & run your C code on Linux ?
$ gcc filename.c
$ ./a.out
- 80 Most Important Network Fundamentals Questions With Answers
- 100 Most Important SOC Analyst Interview Questions
- Top 40 Cyber Security Questions and Answers
- Top 10 React JS Interview Theory Questions and Answers
- CISSP – Practice Test Questions – 2024 – Set 20 (53 Questions)
- Part 2: Exploring Deeper into CCNA – Wireless (145 Practice Test Questions)
- Part 1: Mastering CCNA – Wireless (145 Practice Test Questions)
- [1z0-1085-20] Oracle Cloud Infrastructure Foundations 2020 Associate MCQ Questions – Part 3
- [1z0-1085-20] Oracle Cloud Infrastructure Foundations 2020 Associate MCQ Questions – Part 2
- [1z0-1085-20] Oracle Cloud Infrastructure Foundations 2020 Associate MCQ Questions – Part 1