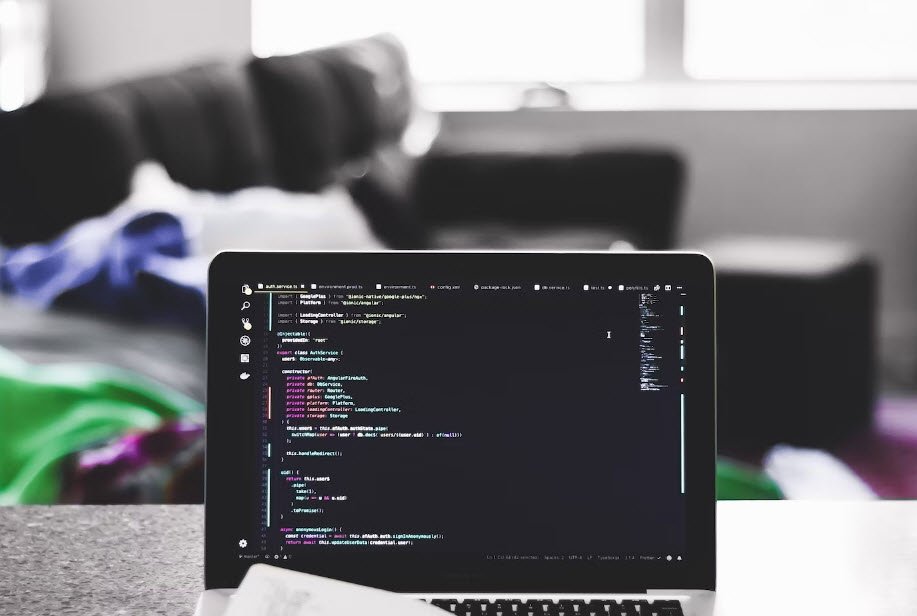
Java Programming Questions are always the deciding factor in any Java job interview. Whether you are a beginner in Java or an expert programmer, you will be tested for your coding skills in the interview.
So, it’s a good idea to brush up your coding skills before you face the interview.
A list of the most popular Java Programming interview questions and answers are explained below and these questions will help you to clear any Java Interview successfully.
1Q. What do you know about Java Programming Language ?
Java is a general purpose, object oriented programming language, designed by James Gosling in 1991. First version of Java was launched on May 23, 1995. Earlier, Java was named as Oak.
2Q. Tell me some important features of Java.
Features of JAVA :
- Java is class based object oriented, compiled & Interpreted programming language.
- Platform Independent & Portable.
- Java uses the concept of Multithreading.
- Java is dynamic – as it can multiple programs and applications dynamically. Ex- Libraries.
- Java is Robust & Secure.
3Q. What are Identifiers in Java ?
Identifiers are the name given to a class, method, or a variable. Identifiers can start from alphabets, $ sign, but cannot start with numbers or any other sign, except underscore.
4Q. Explain the procedure of compilation of a Java Code ?
For compiling a source code, Java uses both compiler as well as interpreter. Java program takes place at two stages: Compiler & Interpreter.
Compiler changes a source code to byte code (.class file) and Interpreter changes byte code to machine code.
5Q. What are access modifiers in Java ?
In Java, Access modifiers, specifies the accessibility or scope of a field, method, constructor, or a class.
6Q. Name some access modifiers in Java ?
There are four access modifiers in Java Programming Language :
- public
- protected
- default.
- private.
7Q. Name some non access modifiers ?
“final, abstract, strictfp, synchronized, volatile” are non access modifiers.
8Q. Explain me the role of JVM.
JVM stands for Java Virtual Machine. JVM is a software that provides run time environment in which java byte code can be executed.
9Q. Explain JRE ?
JRE is Java Runtime Environment. It is the implementation of JVM.
10Q. What is JDK ?
JDK is Java Development kit. It is the collection of programming tools, JRE, JVM.
11Q. What is Applet ?
It is a small application written in Java or any other language, that compiles in Byte code. It is basically a small internet based program embedded inside a pattern.
12Q. What is a Class ?
A Class is a specification or a template of an object. Class contains variables and methods.
13Q. What is an Object ?
Object is an instance (dynamic memory allocation) of a class. The process of creating objects out of class is known as instantiation. Object has a state, behaviour and an identity.
14Q. Explain OOPS methodology ?
The entire OOPS methodology has been derived from a single root called object. In OOPS, all programs involve creation of classes and object. Four important features of OOPS are :
- Encapsulation
- Abstraction
- Inheritance
- Polymorphism
15Q. What is Procedural oriented programming ?
It is the methodology where programming is done using procedures & functions.
16Q. Is Java an object oriented or object based programming language ?
Object Based programming language does not support all features of OOPs like Polymorphism and Inheritance. Java is an object oriented programming language. Some of object based programming languages are JavaScript, Visual Basics.
17Q. Explain Encapsulation with example ?
Encapsulation is a mechanism where the data and the code that act on the data will bind together.
Example : Class is an example of encapsulation. As class is the collection of data members and member functions, hence class binds them together.
18Q. Explain Abstraction with example ?
Abstraction refers to the act of hiding the background details and providing only necessary details to the user.
Example : Let us the example of a car. While driving car, driver(user) only knows basic operations like gearing system, brakes, but is totally unaware about the internal happenings and processes. This is abstraction.
19Q. Explain Polymorphism with example ?
Polymorphism represents the ability to assume several different forms of a program.
Example : Overloading of methods is an example of Polymorphism. Other examples include, abstract classes and interfaces.
20Q. How we achieve the concept of polymorphism in Java?
Inheritance, Overloading and Overriding are used to achieve Polymorphism in java.
21Q. Explain the different forms of Polymorphism.
Polymorphism is divided into two forms :
1. Compile time Polymorphism
2. Run time Polymorphism
Compile time polymorphism is method overloading. Runtime time polymorphism is done using inheritance and interface.
22Q. Explain Inheritance with example ?
Inheritance refers to the process in which child class inherits the properties of its parent class. A class that is inherited is called a superclass. The class that does the inheriting is called a subclass. Inheritance is done by using the keyword extends.
Example : Suppose we are having two classes A and B, class B extends A -> Child class B, is inheriting the property of its parent class.
23Q. What are the types of Inheritance shown by Java ?
There are four types of Inheritance in Java :
1. Single Inheritance
2. Multilevel Inheritance
3. Hybrid Inheritance
4. Hierarchical Inheritance
24Q. Explain different types of Inheritance ?
- Single Inheritance : It is a type of inheritance, where a single child class inherits the property of its parent class.
- Multilevel Inheritance : A class inherits properties from a class which again has inherits properties.
- Hybrid Inheritance : A hybrid inheritance is a combination of more than one types of inheritance.
- Hierarchical Interitance : In this type of Inheritance, more than one classes are derived from a single base class or parent class.
25Q. Why Java Doesn’t Support multiple Inheritance ?
This is due to the ambiguity problem.
26Q. What happens if super class and sub class having same field name ?
Super class fields will be hidden, meanwhile fields of Subclass will be visible. However, you can also access super class fields with super keyword.
27Q. Can a children class inherit a constructor of its parent class ?
No.
28Q. What is Method Overloading ?
Method Overloading means to have two or more methods with same name in the same class with different arguments. A method can be overloaded in the same class or in a subclass.
29Q. What is Method Overriding ?
Method overriding occurs when sub class declares a method that has the same type arguments as a method declared by one of its superclass.
30Q. Can we override a method marked as final ?
No, we can not override a method marked with final.
31Q. Can we override a method marked as static ?
No, we can not override a method marked with static.
32Q. Is it possible to override the main method ?
No, main is a static method. A static method can’t be overridden in Java.
33Q. Explain the role of implements keyword ?
implements keyword is used to developed inheritance between interface and class.
34Q. Is String a keyword in java?
No. String is not a keyword in java. String is a final class in java.lang package which is used to represent the set of characters in java.
35Q. Can we overload a static method in Java?
Yes.
36Q. Can we override a static method in Java?
No.
37Q. Can a class implement more than one interface in Java?
Yes.
38Q. Can a class extend more than one class in Java?
No.
39Q. How we can create object without using new operator ?
Using factory methods:
NumberFormat obj = NumberFormat. getNumberInstance( );
Here, we are creating NumberFormat object using the factory method getNumberInstance().
40Q. What are reference variables ?
Reference variable usually points to object. In other words, a reference variable is associated with an object. For example :
A ob = new A();
Here, ob is a reference variable for a class A. Reference variable are like pointers in C.
41Q. Explain Mutable and Immutable object in Java ?
- Mutable objects are the objects which can be modified. For example : StringBuffer.
- Immutable objects are the objects which can not be modified. For example : String.
42Q. Where objects are stored in memory ?
Objects are stored in heap memory.
43Q. What is memory leak ?
A Memory Leak is a situation when there are objects present in the heap that are no longer used, still, garbage collector is unable to remove them from memory and, thus they are unnecessarily maintained.
44Q. Explain the difference between an object and an instance ?
An Object may or may not have a class definition.
Example – int arr[] where arr is an array.
An Instance should have a class definition.
Example – Solution sol = new Solution(); Here, sol is an instance.
45Q. Explain the concept of Singleton Class ?
In object-oriented programming, a singleton class is a class that can have only one object at a time.
46Q. What is the role of super keyword ?
Super keyword is used to access the method or member variables from the superclass. If a method overrides one of the methods in its superclass, the method can invoke the overridden method through the use of the super keyword.
47Q. What is an Interface ?
An Interface in the Java programming language is an abstract type that is used to specify a behavior that classes must implement.
Defining an Interface :
access interface name {
return-type method-name1();
return-type method-name2();
type final var1 = value;
}
An Interface cannot extend anything but another interfaces. Interfaces can not be final.
48Q. Can we instantiate an interface ?
No, You can’t instantiate an interface directly, but you can instantiate a class that implements an interface.
49Q. What is an abstract class ?
Abstract classes are the classes that usually contain one or more abstract methods. An abstract method is a method that is declared, but contains no implementation. Whenever abstract keyword is used in front of a class, then it becomes an abstract class. If even a single method is abstract, the whole class must be declared abstract.
50Q. Can we instantiate an abstract class ?
No.
51Q. Can a class be marked with abstract & final ?
No, not possible.
52Q. When you declare a method as abstract, can other non abstract methods access it?
Yes.
53Q. Can there be an abstract class with no abstract methods in it ?
Yes.
54Q. Explain Constructor ?
Constructor is defined as a special method that is used for initializing the objects of a class. Constructor has same name as its class. Constructor does not have any return type.
55Q. How are this() and super() used with constructors ?
Constructors use this to refer to another constructor in the same class with different parameters. Constructors use super keyword to invoke the superclass’s constructor.
56Q. What is final modifier?
Whenever a variable is marked with final, it means that its value can not be changed.
57Q. What is static block?
Static block is a block of code, which is executed exactly once i.e when the class is first loaded into JVM. Before main method the static block executes.
Syntax :
static
{
System.out.println(” This is a static block.”);
}
58Q. What are Static variables ?
It is a variable which belongs to the class and initialized only once at the start of the execution.
59Q. A static method can only call other static methods. True or False ?
True.
60Q. A static method can reference to the current object using keywords super or this. True or False ?
False.
61Q. What are instance variables ?
Instance variables are the variables used within a class but outside a method. In this, each instance of class, contains its own copies of these variables.
62Q. What if we include a return type to a constructor ?
No error, as it will be considered as a method.
63Q. Can a method name be same as class name ?
Yes.
64Q. What is Constructor Overloading ?
Constructor overloading is the process of defining more than one constructors, each one having different parameters.
65Q. Do we have destructors in java ?
No. Same job is done through Garbage Collection.
66Q. Explain the role of garbage collector ?
When no reference to an object exists, that object is needed to be no longer needed, and the memory occupied by the object can be reclaimed. There is no need to explicitly destroying the objects. This is the whole process of garbage collection in java.
67Q. What are packages in Java ?
Package in Java is a mechanism to encapsulate a group of classes, sub packages and interfaces. It is achieved through package keyword.
68Q. What is S.C.P ?
String Constant Pool is the memory space in heap memory specially allocated to store the string objects created using string literals. In String Constant Pool, there will be no two string objects having the same content.
69Q. Which is the final class in these three classes – String, StringBuffer and StringBuilder?
All three are final.
70Q. Which one will you prefer among == and equals() method to compare two string objects?
equals() method because it compares two string objects based on their content. If you use == operator, it checks only references of two objects are equal or not.
71Q. Which class will you recommend among String, StringBuffer and StringBuilder classes if I want mutable and thread safe objects?
StringBuffer.
72Q. What is the similarity and difference between String and StringBuffer class?
The main similarity between String and StringBuffer class is that both are thread safe. The main difference between them is that String objects are immutable where as StringBuffer objects are mutable.
73Q. What is the similarity and difference between StringBuffer and StringBuilder class ?
The main similarity between StringBuffer and StringBuilder class is that both produces mutable string objects. The main difference between them is that StringBuffer class is thread safe where as StringBuilder class is not thread safe.
74Q. What is an exception ?
An exception is an event, which occurs during the execution of a program, that disrupts the normal flow of the program’s instructions.
75Q. What is an error ?
An Error indicates that a non-recoverable condition has occurred that should not be caught.
76Q. Which is superclass of Exception ?
Throwable Class, is the parent class of all exception related classes.
77Q. How exceptions can be handled in Java ?
Using try, catch, throw, throws.
78Q. Explain the role of finally block ?
It creates a block of code, that will be executed after a try/catch block or before. It will be executed everytime, no matter whether an exception is thrown or not.
79Q. What are the types of Exceptions ?
Checked and Unchecked.
80Q. Can we have the try block without catch block ?
Yes, we can have the try block without catch block, but finally block should follow the try block.
81Q. What is a Thread ?
A Thread refers to the smallest unit of processing.
82Q. How can you gain the concept of multithreading in Java ?
In either of two ways :
- Extending Thread class
- Implementing Runnable interface.
83Q. Explain Thread Synchronization ?
When two or more threads needs access to a shared resource, they need some way to ensure that the resource will be used by only one thread at a time. This is Synchronization. It is achieved through synchronized keyword in Java.
84Q. What is the difference between yield() and sleep()?
- yield() allows the current thread to release its lock from the object and scheduler gives the lock of the object to the other thread with same priority.
- sleep() allows the thread to go to sleep state for x milliseconds. When a thread goes into sleep state it doesn’t release the lock.
85Q. What is the difference between wait() and sleep()?
wait() is a method of Object class. sleep() is a method of Object class. sleep() allows the thread to go to sleep state for x milliseconds. When a thread goes into sleep state it doesn’t release the lock. wait() allows thread to release the lock and goes to suspended state. The thread is only active when a notify() or notifyAll() method is called for the same object.
86Q. What is difference between notify() and notifyAll()?
notify( ) wakes up the first thread that called wait( ) on the same object. notifyAll( ) wakes up all the threads that called wait( ) on the same object. The highest priority thread will run first.
87Q. What is volatile keyword ?
Volatile keyword is used to modify the value of a variable by different threads. It is also used to make classes thread safe.
88Q. What are the daemon threads?
Daemon threads are special threads that run in the background, however, these not used to run the application code generally.
89Q. How many locks does an object have?
Each object has only one lock.
90Q. What are Collections in Java ?
The Collection in Java is a framework that provides an architecture to store and manipulate the group of objects. Java Collections can achieve all the operations like searching, sorting, insertion, manipulation, and deletion.
91Q. Explain checked & unchecked exceptions ?
Checked Exception : Exceptions are checked by the compiler at the time of compilation, are checked exceptions.
Example – ClassNotFound Exception
Unchecked Exception : These exceptions are not checked during the compile time by the compiler.
Example – ArrayIndexOutOfBounds Exception
92Q. What is the role of finalize() ?
finalize() method is a protected and non-static method of java.lang.Object class. This method is used to perform some final operations or clean up operations on an object before it is removed from the memory.
You may also like:- 80 Most Important Network Fundamentals Questions With Answers
- 100 Most Important SOC Analyst Interview Questions
- Top 40 Cyber Security Questions and Answers
- Top 10 React JS Interview Theory Questions and Answers
- CISSP – Practice Test Questions – 2024 – Set 20 (53 Questions)
- Part 2: Exploring Deeper into CCNA – Wireless (145 Practice Test Questions)
- Part 1: Mastering CCNA – Wireless (145 Practice Test Questions)
- [1z0-1085-20] Oracle Cloud Infrastructure Foundations 2020 Associate MCQ Questions – Part 3
- [1z0-1085-20] Oracle Cloud Infrastructure Foundations 2020 Associate MCQ Questions – Part 2
- [1z0-1085-20] Oracle Cloud Infrastructure Foundations 2020 Associate MCQ Questions – Part 1