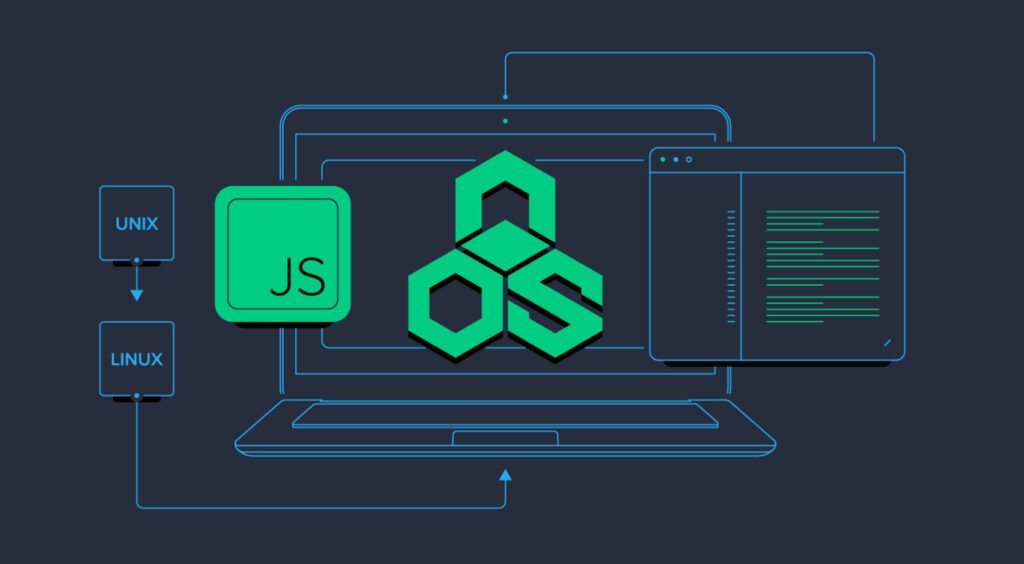
Node.js, often simply referred to as Node, has revolutionized server-side development. It’s a runtime environment that enables developers to use JavaScript for building server-side applications, opening up new horizons for both front-end and back-end developers.
This article will provide a comprehensive overview of Node.js, covering its key features, modules, tools, and practical use cases.
1. Introduction to Node.js
JavaScript Runtime for Server-Side Development
Traditionally, JavaScript was primarily used for client-side web development. Node.js, however, extends JavaScript’s capabilities to server-side development, allowing developers to build scalable, efficient, and high-performance applications. It is built on the V8 JavaScript engine, developed by Google, which compiles JavaScript directly into machine code, resulting in impressive speed and performance.
Non-Blocking I/O
One of Node.js’s defining features is its non-blocking I/O, which allows applications to handle multiple operations concurrently. This asynchronous programming model is a game-changer for applications that require high concurrency and real-time interactions, such as chat applications, online gaming platforms, and streaming services. Node.js leverages an event-driven architecture and a single-threaded event loop to manage I/O operations efficiently.
2. Setting Up Node.js
Getting started with Node.js is a straightforward process:
Installing Node.js and NPM
Node.js comes bundled with npm (Node Package Manager), a powerful tool for managing packages and dependencies. You can download Node.js and npm from the official website, and they are available for various platforms, making it accessible to developers across the board.
Package.json Configuration
Package.json is a crucial file in Node.js projects. It stores metadata about the project, as well as a list of dependencies. You can create it manually or use `npm init` to generate one, which is particularly helpful when sharing your project with others.
Node Version Manager (NVM)
Managing different Node.js versions for various projects is simplified with NVM. This tool allows developers to switch between Node.js versions seamlessly, ensuring compatibility with their projects.
3. Node.js Modules
Node.js encourages modular development, allowing you to break down your code into manageable units. There are three types of modules in Node.js:
CommonJS Modules (require, module.exports)
CommonJS modules are the most common way to create modular code in Node.js. They allow you to split your code into smaller files and include them where needed using `require`. You can export functions, objects, or variables using `module.exports`.
ES6 Modules (import, export)
Node.js introduced support for ES6 modules, making it more compatible with modern JavaScript development practices. You can use `import` and `export` to structure your code in a more organized manner.
Built-in Modules (e.g., fs, http, events)
Node.js comes with a variety of built-in modules that provide essential functionality for various purposes. For example, the `fs` module lets you work with the file system, while the `http` module facilitates the creation of web servers.
4. Core Concepts
Understanding the core concepts of Node.js is crucial for effective development:
Event Loop
The event loop is at the heart of Node.js’s asynchronous nature. It continuously listens for events and executes associated callbacks, allowing Node.js to handle multiple requests efficiently without blocking.
Callbacks and Asynchronous Programming
Callbacks are essential in Node.js for handling asynchronous operations. They ensure that the program doesn’t block and can continue processing other tasks while waiting for I/O operations to complete.
Streams and Buffers
Streams and buffers are fundamental for working with data in Node.js. Streams allow you to read and write data in chunks, while buffers provide a way to manipulate binary data efficiently.
5. Core Modules
Node.js offers various core modules for performing specific tasks:
- fs (File System) – The `fs` module is used for interacting with the file system. It enables you to read, write, and manipulate files and directories.
- http and https (HTTP Modules) – Node.js’s HTTP modules empower developers to create web servers and interact with HTTP and HTTPS protocols, making it ideal for building web applications and APIs.
- events (Event Emitter) – The `events` module is the foundation of event-driven programming in Node.js. It enables you to create custom events and handle them as needed.
- util (Utilities) – The `util` module provides a collection of utility functions and classes for simplifying common programming tasks.
- os (Operating System) – The `os` module gives you access to information about the operating system your Node.js application is running on. This can be helpful for various system-related tasks.
- path (Path Module) – The `path` module simplifies working with file and directory paths, ensuring compatibility across different operating systems.
6. NPM (Node Package Manager)
Node Package Manager (NPM) is a powerful tool for managing dependencies, creating and sharing packages, and streamlining the development process:
Installing Packages
NPM allows you to install packages easily by running `npm install package-name`. It resolves dependencies and keeps track of them in the `package.json` file.
Creating and Managing package.json
The `package.json` file specifies your project’s metadata and dependencies. NPM provides commands like `npm init` and `npm install` to help you manage it.
Semantic Versioning
NPM follows semantic versioning, which helps developers understand how package updates affect their projects. Versions are structured as major.minor.patch, and dependencies can be configured accordingly.
NPM Scripts
NPM allows you to define custom scripts in the `package.json` file. These scripts can automate various tasks, such as running tests, building your application, or deploying it.
7. Asynchronous Programming in Node.js
Asynchronous programming is a fundamental aspect of Node.js development, and several techniques are used to handle it:
Callbacks
Callbacks are the traditional way of handling asynchronous operations in Node.js. They ensure that code execution doesn’t halt while waiting for I/O operations to complete.
Promises
Promises provide a more structured and readable way to manage asynchronous tasks. They help avoid callback hell and make error handling more straightforward.
Async/Await
Introduced in ES6, async/await provides a more synchronous-style code structure for managing asynchronous operations. It simplifies error handling and promotes clean, readable code.
Error-First Callbacks
Node.js conventions suggest using error-first callbacks, where the first parameter of a callback function is used to propagate errors. This pattern enhances error handling consistency.
8. Express.js Framework
Express.js is a widely-used web application framework for Node.js. It simplifies the process of building web applications and APIs, offering various features:
Routing
Express.js provides a robust routing system, enabling you to define routes, request handlers, and middleware for different URL endpoints.
Middleware
Middleware functions can be added to the request-response cycle to perform tasks like authentication, logging, and data validation.
Templating Engines (Pug, EJS)
Express.js supports multiple templating engines like Pug and EJS, making it easy to generate dynamic HTML content.
RESTful APIs
Creating RESTful APIs with Express is straightforward, thanks to its routing capabilities and support for HTTP methods.
Error Handling Middleware
Express allows you to implement error-handling middleware to gracefully handle unexpected errors in your application.
9. Working with Databases
Node.js is well-suited for database interactions, and it supports a variety of databases:
Connecting to Databases (MongoDB, MySQL)
Node.js has libraries and modules for connecting to both SQL and NoSQL databases. For example, Mongoose is a popular choice for MongoDB, while Sequelize is commonly used for MySQL.
Database Migrations and Seeders
Managing database schema changes and populating databases with initial data is simplified using migration and seeder tools.
10. Authentication and Authorization
Node.js offers several options for implementing authentication and authorization:
JSON Web Tokens (JWT)
JWT is a popular method for secure user authentication. Node.js libraries like `jsonwebtoken` simplify the generation and verification of JWTs.
Passport.js Middleware
Passport is a widely-used authentication middleware for Node.js that supports various authentication strategies like local, OAuth, and OpenID.
OAuth and OAuth2
Node.js can act as an OAuth 2.0 provider or consumer, enabling secure authentication and authorization with third-party services.
11. Security
Node.js security is of paramount importance, and several tools and practices can enhance it:
Helmet.js (Security Middleware)
Helmet.js is a middleware package that helps secure your Express application by setting various HTTP headers to prevent common security vulnerabilities.
Input Validation and Sanitization
Properly validating and sanitizing user inputs is critical for preventing security vulnerabilities, such as SQL injection and cross-site scripting (XSS) attacks.
Secure Headers
Setting secure HTTP headers in your application can help protect it from various web security threats.
Cross-Origin Resource Sharing (CORS)
CORS is a common security consideration when your application needs to interact with resources on different domains. Node.js has modules to manage CORS.
12. Testing and Debugging
Node.js provides a variety of tools and libraries for testing and debugging:
Unit Testing (Mocha, Chai)
Mocha is a popular test framework, while Chai is an assertion library. Together, they enable the creation of comprehensive unit tests.
Debugging Tools (Node Inspector)
Node Inspector is a debugging tool that simplifies the process of finding and fixing bugs in your Node.js applications.
Load Testing (Artillery, Apache Bench)
Load testing tools like Artillery and Apache Bench help ensure your application can handle heavy traffic without performance degradation.
13. API Documentation
Properly documenting your APIs is essential for their usability. Node.js offers several tools for this purpose:
Swagger
Swagger provides a powerful framework for designing, building, and documenting RESTful APIs.
API Blueprint
API Blueprint is a format for specifying Web APIs in a clear and concise manner. Various tools can generate documentation from these blueprints.
Postman Documentation
Postman can generate documentation for your APIs based on your requests and responses, making it easy for developers to understand and test your API.
14. Real-Time Applications
Node.js excels in building real-time applications that require constant data updates and interactivity:
WebSockets (Socket.io)
Socket.io is a popular library for implementing real-time functionality through WebSockets. It simplifies bidirectional communication between the server and clients.
Server-Sent Events (SSE)
Server-Sent Events are a simpler alternative to WebSockets for real-time data delivery, especially for one-way data flow from the server to the client.
WebRTC for Video Calls
WebRTC is a technology that enables real-time communication, including video calls and peer-to-peer data sharing. Node.js can be used to implement WebRTC applications.
15. Performance Optimization
Node.js can be optimized for better performance and scalability:
Caching Strategies (in-memory, Redis)
Caching frequently requested data in-memory or using a dedicated caching system like Redis can significantly improve response times.
Load Balancing (Nginx, HAProxy)
Load balancing distributes incoming traffic across multiple Node.js server instances to ensure even utilization of resources.
Profiling and Optimization Tools (Node Clinic, New Relic)
Tools like Node Clinic and New Relic provide insights into your application’s performance bottlenecks, helping you optimize it for better efficiency.
16. Deployment and Hosting
Deploying and hosting Node.js applications is straightforward:
Deploying Node.js Apps (PM2, Forever)
Process managers like PM2 and Forever simplify the deployment and management of Node.js applications, ensuring they run continuously.
Hosting Platforms (AWS, Heroku, DigitalOcean)
Several cloud providers, including AWS, Heroku, and DigitalOcean, offer Node.js hosting services, making it easy to deploy your applications.
Continuous Integration and Deployment (Jenkins, Travis CI)
Integrating CI/CD pipelines with your Node.js projects using tools like Jenkins and Travis CI automates the deployment process, ensuring that your code is tested and deployed consistently.
17. RESTful API Design
Designing RESTful APIs is a crucial aspect of server-side development:
Best Practices
Follow RESTful best practices, such as using meaningful URLs, HTTP methods, and status codes to ensure clear and consistent API design.
API Versioning
Versioning your APIs allows you to make changes without breaking existing clients. Common versioning methods include URL and header-based approaches.
HATEOAS (Hypermedia as the Engine of Application State)
HATEOAS is a concept where APIs provide hyperlinks to related resources, enabling clients to navigate the application state dynamically.
18. Middleware and Custom Modules
Creating custom middleware and organizing code into modules helps keep your Node.js projects maintainable:
Creating Custom Middleware
Custom middleware can be designed to meet your application’s specific needs, enhancing its functionality and improving code organization.
Organizing Code into Modules
Dividing your code into modules and following good practices for structuring your projects ensures readability and maintainability.
Publish and Use Private NPM Packages
If you have code that can be reused across different projects, consider publishing it as a private NPM package to simplify code sharing.
19. Logging
Logging is crucial for monitoring and debugging applications:
Winston Logger
Winston is a versatile logging library for Node.js, providing various transports and formats to suit your logging needs.
Morgan Middleware
Morgan is an HTTP request logger middleware that can be used with Express to track and log incoming requests.
Log Rotation Strategies
Log rotation ensures that your log files don’t consume all available storage. Implementing log rotation is essential for maintaining healthy production environments.
20. Streaming and Buffers
Node.js excels in handling data streams and buffers:
Readable and Writable Streams
Node.js supports readable streams for reading data and writable streams for writing data. These streams allow you to work with data in chunks, making it suitable for applications like file uploads and real-time data processing.
Buffers
Buffers are used for handling binary data. They can be converted into various encodings, making them versatile for working with files and network protocols.
Transform Streams
Transform streams in Node.js allow you to manipulate data as it flows through the stream. This is useful for tasks like data compression and decompression.
21. Error Handling and Monitoring
Proper error handling and monitoring are essential for maintaining application health:
Sentry and Error Tracking
Sentry is a popular error tracking and monitoring tool for Node.js. It helps you identify and diagnose issues in your application.
Health Checks and Monitoring Endpoints
Implementing health checks and monitoring endpoints in your application allows you to proactively monitor its status and detect issues before they impact users.
22. Microservices Architecture
Node.js is well-suited for microservices architecture, which is an approach to building applications as a collection of small, independently deployable services:
Principles of Microservices
Microservices follow principles like modularity, independence, and decentralized data management, allowing for scalability and flexibility.
Communication Patterns (REST, gRPC)
Microservices communicate with each other using various patterns, including RESTful APIs and gRPC for high-performance, language-agnostic communication.
Service Discovery and Load Balancing in Microservices
Service discovery tools like Consul and load balancers like Nginx are essential for routing requests to the appropriate microservices and ensuring high availability.
In conclusion, Node.js has emerged as a powerful, versatile, and community-supported platform for server-side development. Its ability to handle asynchronous operations, coupled with an extensive ecosystem of packages and libraries, makes it a compelling choice for a wide range of web and network applications.
Whether you’re building web servers, APIs, real-time applications, or microservices, Node.js equips you with the tools and flexibility to tackle the challenges of modern server-side development.