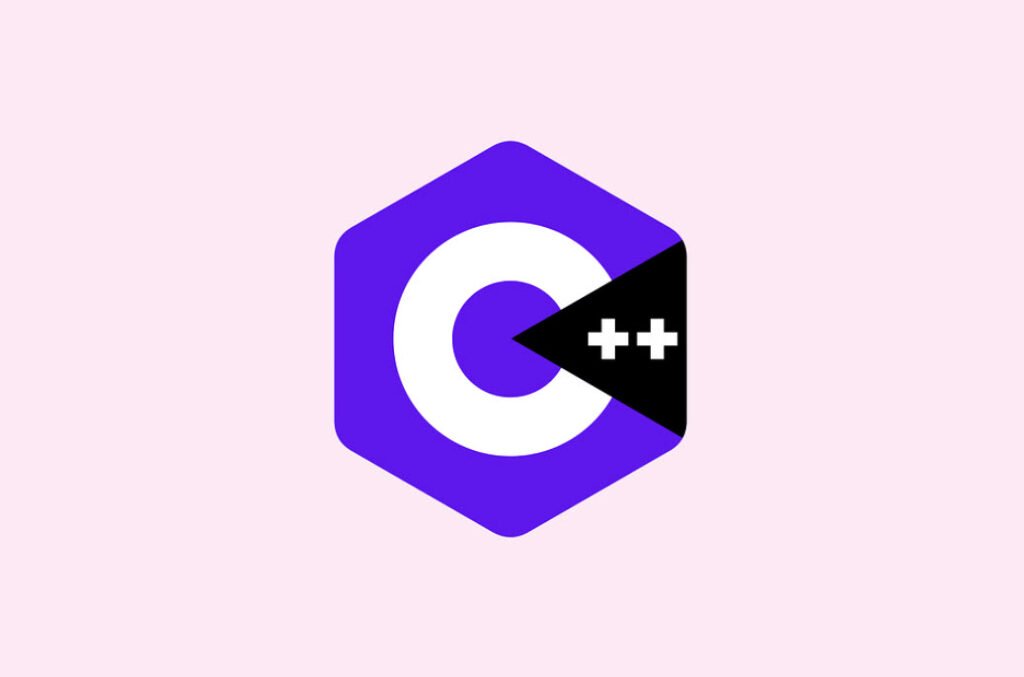
C++ is a powerful programming language. Every programming language follows certain rules which are required for writing even a simple error free programs. In order to write any C++ program, we must be aware of the available keywords, datatypes and have knowledge of variables, constants etc. Since C++ is a superset of C so most of the rules and regulations of C language is also valid in C++.
Index:
- [#1] – Introduction to C++ – Questions
- [#2] – Preparing and Running C++ Program – Questions
- [#3] – C++ Fundamentals – Questions
- [#4] – Data Input and Output – Questions
- [#5] – Control Structures – Questions
- [#6] – Functions – Questions
- [#7] – Arrays and Strings – Questions
- [#8] – Structures and Unions – Questions
- [#9] – Pointers – Questions
- [#10] – Object and Classes – Questions
- [#11] – Constructors and Destructors – Questions
- [#12] – Operator Overloading and Type Conversion – Questions
- [#13] – Inheritance – Questions
- [#14] – Virtual Functions – Questions
- [#15] – C++ Streams and Files – Questions
- [#16] – Templates – Questions
- [#17] – Exception Handling – Questions
In addition to supporting some of the constructs of C, C++ supports certain additional constructs and improvements like strict type checking, allowing declaration of variable anywhere within the program etc.
Key Points To Remember –
- Tokens are the C++ program elements which are identified by the compiler. Token supported in C++ includes keywords, constants, special characters, operators etc.
- A constant is a value that doesn’t change during the program execution. The constants can be classified as integer constants, floating point constants, character constants and string constants.
- An identifier consists of sequence of letters, digits and underscore. They are case sensitive which represents the name of the variables used in a program. An identifier cannot begin with a digit.
- Reserved words that have standard predefined meaning in C++ are called keywords.
- A variable is a symbolic name given to a location in the computer’s memory where a value can be stored which can be used in the program.
- Initializing a variable when it is declared is regarded as a good programming practice.
- The basic difference between the variable declaration and variable definition is that in the former no memory is allocated but in later memory is allocated to the variable. A variable definition consists of a data type followed by one or more variable names ending with a semicolon
- An expression is a combination of constants, variables and operators to form an executable statement which computers a value.
- The symbols used to perform certain operations are called operators and the combination of operators, constants and variables are called expressions.
- In C++ we can declare a variable anywher4e within the program. However, It is recommended to declare as close as possible to its first use.
- The various operators available in C++ are Arithmetic, Operators, Relational operators, conditional operators, unary operators and some additional C++ operators.
- A logical operator evaluates the logical expression to true or false depending upon expression evaluates to true or false.
- The compound assignment operators +=, -=, *=, /= and %= perform an arithmetic operations on a variable and store the results back into the variable.
- The increment (++) and decrement (–) operators are used for incrementing and decrementing integral and floating-point variables.
- Type conversion is the property of converting a variable of one data type to another data type. It is usually classified into two categories.
- Implicit type conversion
- Explicit type conversion or Type Casting
- In implicit type conversion, the operand of lower data type is converted to operand of higher data type automatically before the expression is evaluated.
- Type casting is a form of type conversion in which type of variable is forced to be changed by using cast operator.
- When a floating-point value is stored is an integer variable, then the floating-point value is converted to an integer value by truncating its decimal value.
- The hierarchy of operators refers to the order in which operates are evaluated within an expression.
- Associativity refers to the order in which the consecutive operations within the same precedence group are carried out.
Viva Voce Questions –
Here are commonly asked questions with answers:
- What is the difference between ‘B’ and “B” ?
- ‘B’ is a character constant which contains only ‘B’. However, “B” is a string constant which contains two characters ‘B’ and ‘/0’.
- Suppose i is an integer variable which is assigned 32767. If we increment value of i by 1 then what will be the output ?
- As we know that range of integrs is till.32767 so on adding I will make it out of range and it will start backwards so output will be -32768.
- Why will the output of the statement c = 5/9* ( f-32), always be 0 where c and f are temperatures in degree Celsius and Fahrenheit ?
- As 5/9 is always equal to 0 because both are integer values. So type casting is necessary.
- What is the difference between Ivalue and rvalue ?
- The lvalue of a variable is the address in memory. We may think lvalue as location value. The rvalue is the value of the variable. A variable can act as both lvalue and rvalue whereas a literal constant can only act as rvalue.Consider the following statements
int a, b;
a = 6;
b = a;In the first assignment variable a serve as lvalue and in the second statement variable a act as rvalue.
- The lvalue of a variable is the address in memory. We may think lvalue as location value. The rvalue is the value of the variable. A variable can act as both lvalue and rvalue whereas a literal constant can only act as rvalue.Consider the following statements
- What is the difference between precedence and associativity of operators ?
- The hierarchy of operators refers to the order in which operators are evaluated within an expression whereas associativity refers to the order in which the consecutive operations within the same precedence group are carried out.
- How can you access global variable in the local block of the program if both local and global variable have same name ?
- Using Scope Resolution operator (:)
- What will be the output of statement cout<< ( 10 > 20 ) ? 10:20; ?
- 0, the reason is that insertion operator (<<) has higher precedence than conditional operator (?:)
- Classify the types of operators based on the number of operands ?
- Unary : The operator that uses a single operand.
- Binary : The operator that uses two operands.
- Ternary : The operator that uses three operands.
Other Similar Questions –
- What are tokens ? Why are they useful ?
- What is an identifier ? How is user defined identifier different from standard identifier ?
- What are the rules for declaring valid Identifiers ?
- What are different types of constants used in C++ ?
- How are variables different from constants ?
- What are the character constants ? How does they differ from Integer Constants ?
- What is the difference between ‘D and “D” ?
- What is a variable ? What is the difference between variable, constants and keywords ?
- What are the basic data types available in C++ ?
- How do you calculate the integer data type ?
- Write short notes on the following data types:
- Char
- bool
- float
- double
- What is the difference between variable declaration and variable definition ?
- List the various escape sequences ?
- What is an operator ? List out of the various operators available in C++ ?
- What is an arithmetic operator ? Discuss the use of various arithmetic operators.
- What is the difference between unary and binary operators ?
- What is the basic differences between relational and logical operators ?
- What is a bitwise operator ? List various bitwise operator .
- How does assignment operator differs from equality operator ?
- Why conditional operator is known as ternary operator ?
- What is the difference between prefix and postfix increment operators ?
- Discuss the various unary operators ?
- Why do we need scope resolution operator ?
- What are different types of type conversion ?
- Using example explain the concept of Implicit type conversion ?
- What is type casting ?
- What is the importance of using precedence rules in C++ operators ?
- Explain the term Associativity ? When is it necessary ?
- List of operators having right to left associativity.
- List of operators having left to right associativity.
- Using examples explain the hierarchy of operators ?
- Write ‘True or ‘False’ against the following statements :
- C++ has characters from ASCII character set.
- A token is the smallest program element meaningful to the compiler.
- Keywords are identifiers.
- It is essential to append ‘f‘ or ‘F‘ at the end of the constant of type ‘float’.
- Escape sequences are string constants.
- The range of integer types lie in the range -2x-1 and 2x-1 where x is the number of bits for that type.
- Float is the default floating-point type.
- Variable initialization is same as variable assignment.
- Explicit conversion is also known as widening conversion.
- Unary operators have higher precedence than binary operators.
- Determine weather the following variable names are valid or invalid. If invalid, give the reason why.
- flag
- num
- 12ab
- while
- pay rate
- average
- max_amount
- valid
- total
- numIII
- xyz
- $tax
- FOR
- tta
- max-item
- Write the declarations for the following statements:
- Variable max_item that can store an integer value.
- Variable percentage that can store a floating-point value.
- Variable flag that can store boolean variables ( true or false).
- Variables first, second and third that can store integer values.
- Variables amount, tax and price that can store floating-point numbers.
- An int constant SIZE with value 25.
- A integer variable sum initialized to O.
- A floating-point variable product initialized to 1.
- Evaluate the following expressions :
Suppose x is an integer variable and s is a floating point variable. Assume x= 2 and Y=2.0- x = 44/6
- x= 44%6 + 5*6-4
- x= 50 + 46/7 * (12*5%2)
- x= %= 4/x + 5
- y + 5 + y * y * 10
- y += 4 * 1.6 + (++x)
- y = x++ + 3 * 1.6
- Write a C++ program that defines five integer variables and initializes them to 5, 10, 15, 20, 25 and display them on a single line separated by commas.
- Write a program that extract and prints the rightmost digit of the integral portion of a float.
- If x= 5, y=o and z= -5, what is the value of each of the following expressions.
- x || y && z
- (x || y) && z
- (x && y) || z
- x && y || z
- 80 Most Important Network Fundamentals Questions With Answers
- 100 Most Important SOC Analyst Interview Questions
- Top 40 Cyber Security Questions and Answers
- Top 10 React JS Interview Theory Questions and Answers
- CISSP – Practice Test Questions – 2024 – Set 20 (53 Questions)
- Part 2: Exploring Deeper into CCNA – Wireless (145 Practice Test Questions)
- Part 1: Mastering CCNA – Wireless (145 Practice Test Questions)
- [1z0-1085-20] Oracle Cloud Infrastructure Foundations 2020 Associate MCQ Questions – Part 3
- [1z0-1085-20] Oracle Cloud Infrastructure Foundations 2020 Associate MCQ Questions – Part 2
- [1z0-1085-20] Oracle Cloud Infrastructure Foundations 2020 Associate MCQ Questions – Part 1