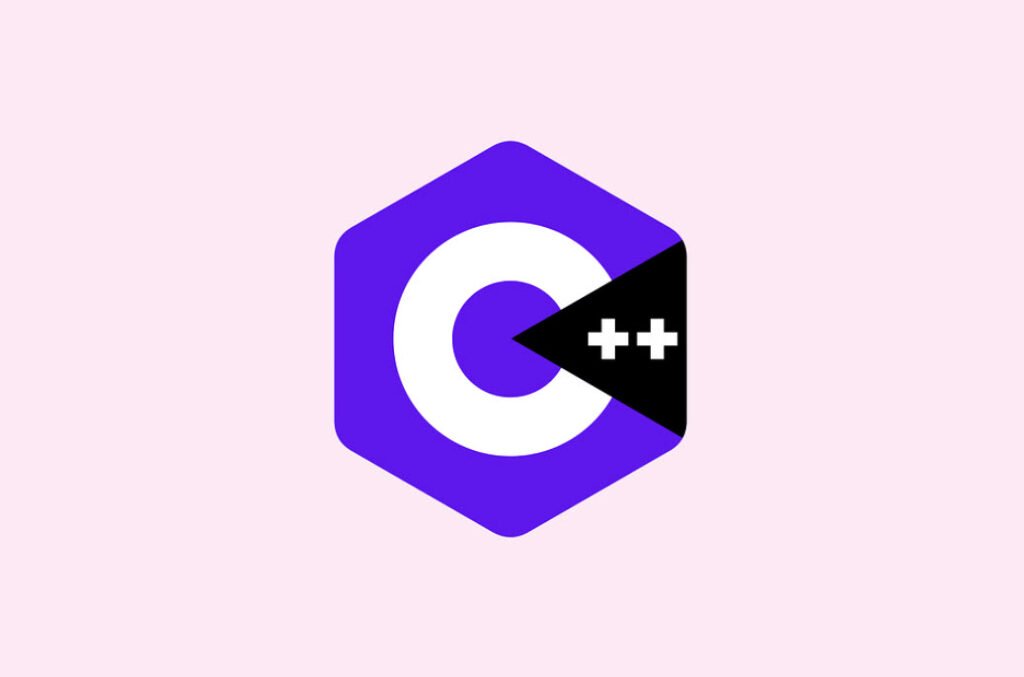
C++ is an Object Oriented Programming language. The key concepts of OOPs include classes, objects, data hiding, encapsulation, abstraction, polymorphism and inheritance. They key concept of Object Oriented Programming language C++ is a class. A class is a fundamental language construct for creating the complex user-defined data types. It distinguishes C++ from C language.
Index:
- [#1] – Introduction to C++ – Questions
- [#2] – Preparing and Running C++ Program – Questions
- [#3] – C++ Fundamentals – Questions
- [#4] – Data Input and Output – Questions
- [#5] – Control Structures – Questions
- [#6] – Functions – Questions
- [#7] – Arrays and Strings – Questions
- [#8] – Structures and Unions – Questions
- [#9] – Pointers – Questions
- [#10] – Object and Classes – Questions
- [#11] – Constructors and Destructors – Questions
- [#12] – Operator Overloading and Type Conversion – Questions
- [#13] – Inheritance – Questions
- [#14] – Virtual Functions – Questions
- [#15] – C++ Streams and Files – Questions
- [#16] – Templates – Questions
- [#17] – Exception Handling – Questions
Its relative importance can be seen from the fact that before the name C++ was coined, the language was called C with classes. Classes with C++ evolved from the concept of structures in C.
Key Points To Remember –
- A class is a logical way of grouping together data and associated functions into a single unit. The body of a class consists of data members and associated member functions which are collectively known as members.
- The access specifiers private and public defines the scope of associated members. The members which are private in a class can only be accessed by member functions of the same class and not from outside the class. On the other hand, public members are not only accessible are private by default.
- An object is a real world entity which is an instance of a class. It can uniquely be identified by its name and it defines a state which is represented by the value(s) of its data member(s) at a particular point of time.
- The private members of a class can only be accessed through its member functions simply by referring their name in the class. Private members cannot be accessed directly from outside the class. However only public members can be accessed from outside using the dot operator(.) which is identical to accessing members of a structure.
- The member function(s) of a class can be either defined inside or outside the class body. They are defined inside as ordinary functions. However, if they are defined outside the class then they are defined using the scope resolution operator.
- The private member function(s) are usually used to hide certain operations of a class from the outsider and serve as utility functions to other functions of the class.
- Data Hiding is a mechanism that prevents direct access of class’s data from the outside world. This is achieved by declaring the data members of a class as private.
- Encapsulation binds data and its associated function into a single unit. In C++, this achieved using a class.
- Data Abstraction is a process of separating the interfaces of a class from its implementation details. It is implemented by declaring the member function inside a class and defining those member functions outside.
- Each object of a class has its own copy of the data members whereas member function gets memory only once corresponding to the class specification.
- An array of objects is a collection of objects of the same class type that are stored in the contiguous memory locations.
- Objects can be passed as an argument by value, by reference or by address.
- A static data member(s) is the data member(s) that gets memory only once for the whole class no matter how many objects(s)of class are created. This common copy is shared by all objects of its class.
- The static member function can access only static members(s) declared in the class.
- When a member function doesn’t modify any any data members of its class then such a member function are declared as const functions.
Viva Voce Questions –
Here are commonly asked questions with answers:
- What are the two major parts of a class ?
- Data and its associated functions.
- How does a structure in C++ differs from that of classes ?
- Both structure and classes in C++ combine data and its associated functions together into a single unit. The basic difference is that structures are specified using struct keyword and all its members are public by default whereas classes are specified using class keyword and all its members are private by default. Moreover, advanced object oriented concepts are not implemented using structures.
- What is an access specifier ?
- It controls which part of the program can access a member of the class.
- What is a difference between public and private access specifier ?
- The member which are public are not only accessed inside the class but also from outside the class. However private member can only be accessed from inside the class.
- What is class instantiation ?
- It is a process of creating objects of a class.
- Is it always necessary to specify access specifier in a class?
- No, if you don’t specify any access specifier then it is private by default.
- Can you define member function outside the class body ?
- Yes, it is possible by declaring the member function inside and defining outside the class body using scope resolution operator.
- Are the member functions defined inside the class inline by default ?
- Yes, provided it contains few statements and doesn’t have control structures like loops, switch, if.
- How much memory is allocated when we create an object of a class ?
- Memory allocated to an object is equal to sum of the size of the its data members.
- Should the data members of a class must always be private ?
- No, it can be public as well as protected.
- How is data hiding implemented in C++ ?
- Data Hiding is implemented by declaring the data members of class as private.
- How is encapsulation implemented in C++ ?
- Encapsulation is implemented using class which binds the data and its associated functions into a single unit.
- How is abstraction implemented in C++ ?
- Abstraction is implemented by declaring the member functions inside the class and defining outside which separate the interfaces of the class from its implementations. The header file (such as math.h) that contains declaration of built-in functions (sin(), sqrt() etc.) provide interfaces to the actual implementations present in the library file.
- What happens when we pass an object by reference ?
- When an object is passed by reference, an alias of the original passed object is created in the called function so any modification to it in the called function are automatically reflected back to the original object.
- Why passing an object by reference is more efficient than by passing a value ?
- While passing an object by reference, only the address of the object is passed and not its entire copy as in case of passing object by value. So, lot of memory is saved especially in case of large objects.
- What is the difference between passing an object by reference to that of pointer ?
- The main difference is that in case of passing object by reference, the address of the object is passed implicitly while passing an object by pointer, the address of the object is passed explicitly to the function.
- Why data members of a class cannot be initialized at the point of declaration ?
- Since memory is allocated to the data members only when an object of a class is created and not during the class specification, so there is no sense to initialize data members at the point of their declaration in the class.
- How are static data members different from non-static data members ?
- Static data members gets memory only once no matter how many objects of a class are created, whereas non-static data members gets separate memory corresponding to each object. Moreover, unlike non-static data members, a static data member can appear as a default argument to the member function of the class.
- Can a data member of a class be an object of the same class ?
- No, because class is just a blue-print and blueprint does not create any object. Objects of a class are created only when the class is defined.
- Can a data member of a class be a static object of the same class ?
- Yes, because static object as a data member of the same class is only declared within the class and is defined (allocated memory) outside the class.
- How will you specify a member function which cannot modify class’s object data member ?
- It is possible by declaring the member function as const. E.g. int avg() const;
- What is a mutuable data member ?
- A data member which is not const even when it is a member of a const member function.
- Can a non-const member function be overloaded with a const member function ?
- Yes, For example,
int display() const,
int display ();
- Yes, For example,
Other Similar Questions –
- What are structures ? How do structures in C differs from C++ ?
- Why do we use class instead of structures ?
- What is the difference between structures in C++ and a class ?
- What is a class ? How is it specified ?
- What do you understand by data members and member functions of a class ?
- Explain access specifiers and why do we need it ?
- What is difference between public and private member functions ?
- What is an object ? How objects can be defined and accessed in C++ ?
- ‘An object is an instance of a class’ . Explain.
- Describe how data members and member function are accessed inside
- main() program
- member function of same class
- inside member function of another class
- What is a scope resolution operator ? How is it useful for defining the members of a class ?
- How will you define a member function inside and outside the class ?
- Create the following classes
- Class – Employee
Data members – employee identification number, employee name, basic pay, dearness allowance, house rent allowance.
Member functions – Input the data , calculate the total salary and display it along with the employee details. - Class – Student
Data members – Roll number, marks of three subjects (100 each).
Member Functions – Input the data, compute average marks in three subjects, compute and display grade of the student.
- Class – Employee
- Explain the concept of inline member functions.
- How does a class promote data hiding, abstraction and encapsulation ?
- How is memory allocated to the data members and member functions of the class ?
- Explain the difference between the interface and the implementation of a class ?
- How and why the scope resolution operator used in classes ?
- What is an array of class objects ? How are they defined in C++ ?
- What are difference ways of passing objects as arguments to the function ?
- Explain the following statements:
- t3. addtime(t1,t2); where t1, t2, t3 are objects of class time having data members hours and minutes. The member function addtime () calculates the sum of two times.
- t3=t1.addtime (t2);
- What do you understand by static date members ? Why we need it ? explain using example.
- What is need of static member functions ?
- When do we use ‘const’ member functions ?
- 80 Most Important Network Fundamentals Questions With Answers
- 100 Most Important SOC Analyst Interview Questions
- Top 40 Cyber Security Questions and Answers
- Top 10 React JS Interview Theory Questions and Answers
- CISSP – Practice Test Questions – 2024 – Set 20 (53 Questions)
- Part 2: Exploring Deeper into CCNA – Wireless (145 Practice Test Questions)
- Part 1: Mastering CCNA – Wireless (145 Practice Test Questions)
- [1z0-1085-20] Oracle Cloud Infrastructure Foundations 2020 Associate MCQ Questions – Part 3
- [1z0-1085-20] Oracle Cloud Infrastructure Foundations 2020 Associate MCQ Questions – Part 2
- [1z0-1085-20] Oracle Cloud Infrastructure Foundations 2020 Associate MCQ Questions – Part 1