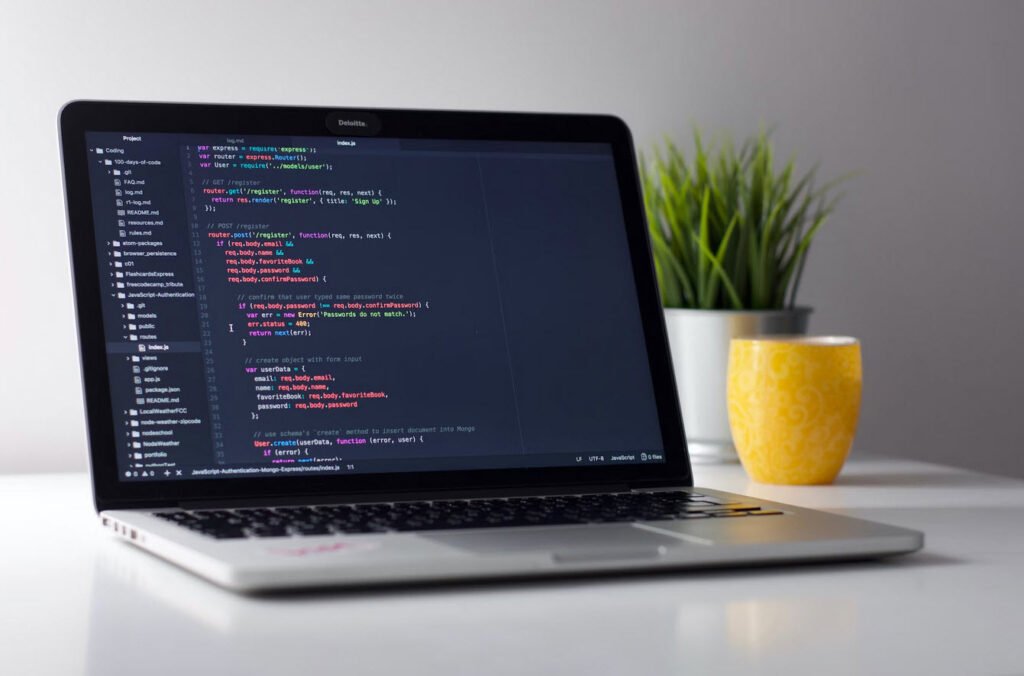
Python, a versatile, high-level, interpreted programming language, has become a powerhouse in various domains, including machine learning, web development, and data science. Aspiring developers and data scientists often encounter a set of fundamental Python interview questions that assess their knowledge of the language’s syntax, concepts, and best practices.
Let’s explore some of these key questions and delve into the underlying concepts that make Python a preferred language for diverse applications.
1. What exactly is Python?
Answer: Python is a general-purpose, high-level, interpreted programming language known for its simplicity and readability. It supports multiple programming paradigms, including procedural, object-oriented, and functional programming.
2. What are the advantages of Python?
Answer: Python’s advantages include a simple syntax, ease of learning, open-source nature, and a rich set of libraries. It supports modular programming, promotes code reuse, and is widely used for rapid application development.
3. What is the definition of a dynamically typed language?
Answer: Python is dynamically typed, meaning the type of a variable is interpreted during runtime. In contrast to statically typed languages, where types are checked before execution, Python allows flexibility in assigning and changing variable types during program execution.
4. What is the definition of an Interpreted Language?
Answer: Python is an interpreted language, meaning that the source code is executed line by line without a separate compilation phase. The interpreter reads and executes the code directly, facilitating rapid development and debugging.
5. What is the meaning of PEP 8, and how significant is it?
Answer: PEP 8, or Python Enhancement Proposal 8, is the style guide for Python code. It outlines conventions for formatting, indentation, and naming conventions, promoting code readability and consistency. Adherence to PEP 8 is crucial for collaborative development within the Python community.
6. What is the definition of scope in Python?
Answer: In Python, each object has its scope, which defines the block of code where the object is relevant. Scopes include local (within a function), global (from the beginning of code execution), module-level (global objects of the current module), and outermost (built-in names).
7. What is the meaning of `pass` in Python?
Answer: The `pass` keyword in Python denotes a null operation. It is used as a placeholder for code blocks that are intended to execute during runtime but have not been written yet. Using `pass` helps avoid issues during code execution.
8. How does Python handle memory?
Answer: Python’s Memory Manager manages memory allocation in the form of a private heap. The private heap holds all Python objects, and the built-in garbage collection system recycles unneeded memory. Python provides basic API methods for working with the private memory area.
9. What are namespaces in Python? What is their purpose?
Answer: Namespaces in Python ensure that object names are unique and used without conflict. They are implemented as dictionaries with names as keys and objects as values. Namespaces include local (within a function), global (current module), and built-in (Python built-in functions) namespaces.
10. What is Python’s Scope Resolution?
Answer: Python’s scope resolution distinguishes objects with the same name but different functions. It prevents conflicts and ensures the correct reference to objects within different scopes.
11. Explain the definition of decorators in Python?
Answer: Decorators in Python are functions that add functionality to an existing function without modifying its structure. They are denoted by the `@decorator` syntax and are applied from the bottom up. Decorators often use inner nested functions to encapsulate additional functionality.
12. What are the definitions of dict and list comprehensions?
Answer: Comprehensions in Python provide concise syntax for creating modified and filtered lists, dictionaries, and sets. Dict comprehensions create dictionaries, and list comprehensions create lists based on specified conditions or transformations.
13. What is the definition of lambda in Python? What is its purpose?
Answer: A lambda function in Python is an anonymous function with a concise syntax. It is often used for short-term tasks and can accept any number of parameters but contains only one expression. Lambda functions are useful for quick, one-time operations.
14. In Python, how do you make a copy of an object?
Answer: Python’s assignment statement (`=`) doesn’t duplicate objects; it establishes a reference. To create copies, the `copy` module is used. Shallow copy duplicates object values, while deep copy recursively replicates all values, including nested objects.
15. What are the definitions of pickling and unpickling?
Answer: Pickling in Python refers to the serialization process, converting objects into a byte stream for storage. Unpickling is the opposite process, reconstructing objects from the stored byte stream. The `pickle` module is used for these operations.
16. What is PYTHONPATH?
Answer: PYTHONPATH is an environment variable that specifies additional directories where Python should look for modules and packages. It helps in managing Python libraries that aren’t installed in the global default location.
17. What are the functions `help()` and `dir()` used for?
Answer: The `help()` function displays information about modules, classes, functions, keywords, and other objects. The `dir()` function attempts to return a list of the object’s attributes and methods, providing insights into available functionality.
18. How can you tell the difference between .py and .pyc files?
Answer: .py files contain Python source code, while .pyc files store the bytecode generated by compiling the .py files. The bytecode (.pyc) is an optimized version of the code that the Python interpreter can execute more efficiently.
19. What does the Python interpreter interpret?
Answer: The Python interpreter interprets the bytecode generated from Python source code. Python is not strictly interpreted or compiled; it uses an intermediate format known as bytecode, executed by the Python interpreter.
20. In Python, how are arguments delivered by value or reference?
Answer: Python passes arguments by reference, meaning a reference to the real object is passed. However, it behaves differently from pass-by-reference in other languages. If the value of the referenced object is changed inside a function, it doesn’t affect the original object outside the function.
Navigating through these Python interview questions and concepts provides a solid foundation for developers and data scientists aiming to showcase their proficiency in this versatile programming language. Whether mastering basic syntax, understanding memory management, or exploring advanced concepts, Python’s adaptability and readability make it a sought-after language for various applications.
You may also like:- 80 Most Important Network Fundamentals Questions With Answers
- 100 Most Important SOC Analyst Interview Questions
- Top 40 Cyber Security Questions and Answers
- Top 10 React JS Interview Theory Questions and Answers
- CISSP – Practice Test Questions – 2024 – Set 20 (53 Questions)
- Part 2: Exploring Deeper into CCNA – Wireless (145 Practice Test Questions)
- Part 1: Mastering CCNA – Wireless (145 Practice Test Questions)
- [1z0-1085-20] Oracle Cloud Infrastructure Foundations 2020 Associate MCQ Questions – Part 3
- [1z0-1085-20] Oracle Cloud Infrastructure Foundations 2020 Associate MCQ Questions – Part 2
- [1z0-1085-20] Oracle Cloud Infrastructure Foundations 2020 Associate MCQ Questions – Part 1