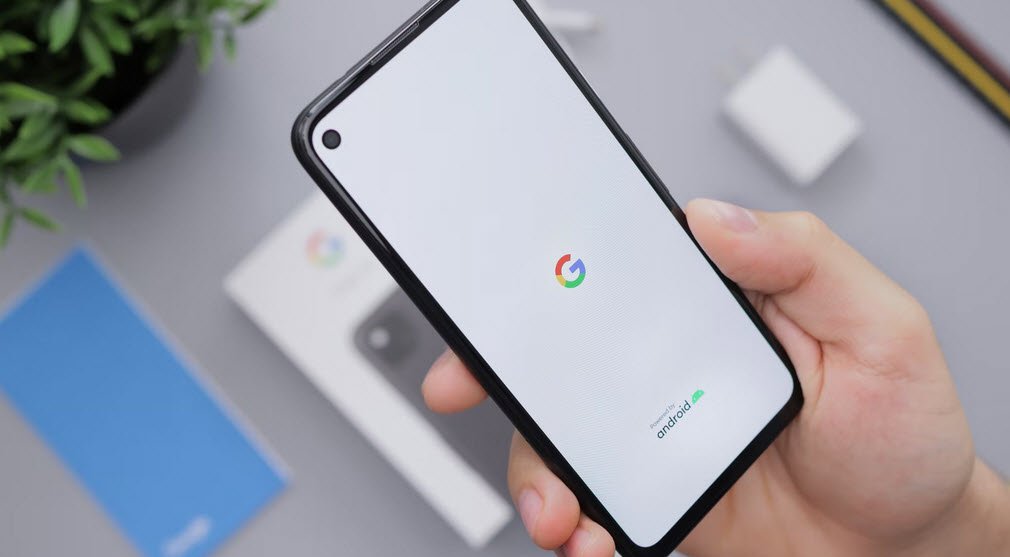
Android devices differ in significant ways. Some devices may have a faster processor than others, some devices may have more memory than others, and some devices may include a Just-In-Time (JIT) compiler, whereas other devices don’t have this technology for speeding up executable code by converting sequences of bytecode instructions to equivalent native code sequences on the fly.
Also Read: Things To Consider While Designing Real Time Android Applications
The following list identifies some things to consider when writing code so that your apps will perform well on any device:
Optimize your code carefully
Strive to write apps with a solid architecture that doesn’t impede performance before thinking about optimizing the code. Once the app is running correctly, profile its code on various devices and look for bottlenecks that slow the app down.
Keep in mind that the emulator will give you a false impression of your app’s performance. For example, its network connection is based on your development platform’s network connection, which is much faster than what you’ll probably encounter on many Android devices.
Minimize object creation
Object creation impacts performance, especially where garbage collection is concerned. You should try to reuse existing objects as much as possible to minimize garbage collection cycles that can temporarily slow down an app.
For example, use a java.lang.StringBuilder object (or a java.lang.StringBuffer object when multiple threads might access this object) to build strings instead of using the string concatenation operator in a loop, which results in unnecessary intermediate String objects being created.
Minimize floating-point operations
Floating-point operations are about twice as slow as integer operations on Android devices; for example, the floating-point-unit-less and JIT-less G1 device.
Also, keep in mind that some devices lack a hardware-based integer division instruction, which means that integer division is performed in software. The resulting slowness is especially bothersome where hashtables (that rely on the remainder operator) are concerned.
Use System.arraycopy() wherever you need to perform a copy
The java.lang.System class’s static void arraycopy(Object src, int srcPos, Object dest, int destPos, int length) method is around nine times faster than a hand-coded loop on a Nexus One with the JIT.
Avoid enums
Although convenient, enums add to the size of a .dex file and can impact speed. For example, public enum Directions { UP, DOWN, LEFT, RIGHT } adds several hundred bytes to a .dex file, compared to the equivalent class with four public static final ints.
Use the enhanced for loop syntax
In general, the enhanced for loop (such as for (String s: strings) {}) is faster than the regular for loop (such as for (int i = 0; i < strings.length; i++)) on a device without a JIT and no slower then a regular for loop when a JIT is involved.
Because the enhanced for loop tends to be slower when iterating over a java.util.ArrayList instance, however, a regular for loop should be used instead for arraylist traversal.
You’ll also want to choose algorithms and data structures carefully.
For example, the linear search algorithm (which searches a sequence of items from start to finish, comparing each item to a search value) examines half of the items on average, whereas the binary search algorithm uses a recursive division technique to locate the search value with few comparisons.
For example, where a linear search of 4 billion items averages 2 billion comparisons, binary search performs 32 comparisons at most.
You may also like:- Six Ways to Speed Up Your iPhone – A Comprehensive Guide
- Top 3 Android Obfuscators Tools
- Essential Guidelines for Enhanced Security in Mobile Apps
- Top Issues Facing Mobile Devices
- Top 11 Essential Tips for Secure Mobile Application Development
- Things To Consider While Designing Real Time Android Applications
- [India] Top 10 Smartphone Apps Of All The Time