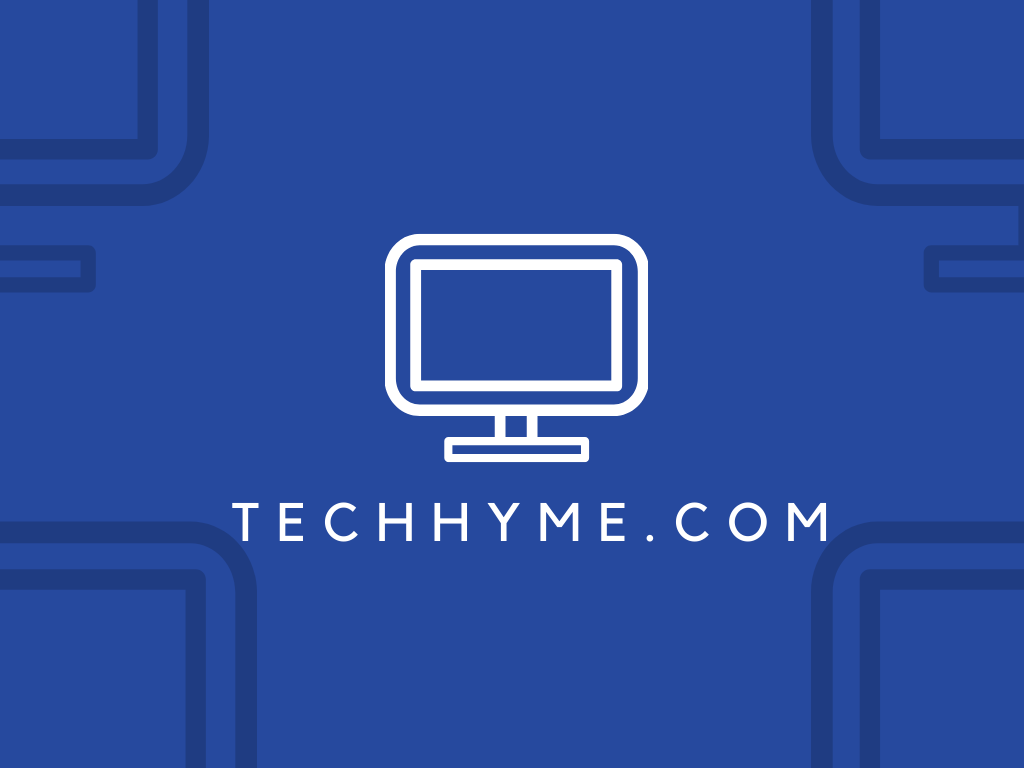
If your teacher asked you to solve an arithmetic problem and you are not familiar with the steps involved in solving the problem, you will not be able to solve it. The same principle applies to writing computer programs also. A programmer cannot write the instructions for a computer to execute unless the programmer knows how to solve the program manually.
If you know the steps for solving a problem but while solving it, you forget to apply some of the steps or you apply the steps in the wrong sequence, you will get a wrong answer.
Similarly, while writing a computer program if the programmer leaves out some of the instructions for the computer or writes the instructions in the wrong sequence, the computer will produce a wrong answer.
Hence, to produce an effective computer program it is necessary to write every instruction in the correct sequence. However, the instruction sequence (logic) of a computer program may be very complex. Hence, to ensure that the instructions of a program are appropriate for the problem at hand and are in the correct sequence, we must plan the program before writing it.
Also Read:
- [#1] – Introduction to Computer Fundamentals
- [#2] – Basic computer organization
- [#3] – Number systems
- [#4] – Computer Codes
- [#5] – Computer Arithmetic
- [#6] – Processor and Memory
- [#7] – Secondary Storage Devices
- [#8] – Input-Output Devices
- [#9] – Computer software
- [#10] – Planning the Computer Program
- [#11] – Computer Languages
- [#12] – System Implementation and Operation
- [#13] – Operating Systems
- [#14] – Application Software packages
- [#15] – Business Data Processing
- [#16] – Data Communications and Computer Networks
- [#17] – The Internet
- [#18] – Multimedia
- [#19] – Classification of Computers
- [#20] – Introduction to C Programming Language
Points To Remember:
- To ensure that the program instructions are appropriate for the problem at hand and in the correct sequence, programs must be planned before they are written.
- The term algorithm refers to the logic of a program. It is a step-by-step description of how to arrive at a solution to a given problem.
- An algorithm represented in the form of a programming language is a program. Hence, any program is an algorithm, although the reverse is not true.
- Other than programs, the use of flowcharts and pseudocodes is also common for representation of algorithms. They are frequently used by programmers for program planning.
- A flowchart is a pictorial representation of an algorithm. Programmers often use it as a program-planning tool for visually organizing a sequence of steps necessary to solve a problem using a computer.
- Pseudocode is a program-planning tool that allows programmers to plan program logic by writing program instructions in an ordinary natural language, such as English.
- Structured Programming is a more scientific approach to solving a programming problem by using only the three basic logic (control) structures namely, sequence logic, selection logic, and iteration (or looping) logic.
- Structured programming helps in producing programs that are easy to understand and maintain. It also helps in reducing program errors and the time spent in program testing.
- Sequence logic is used of performing instructions one after another in sequence.
- Selection logic is used for selecting the proper path out of two or more alternative paths in program logic. It uses three control structures called IF…THEN, IF….THEN….ELSE, and CASE.
- Iteration (or looping) logic is used for producing loops in program logic when one or more instructions may be executed several times depending on some condition. It uses two control structures called DO…WHILE and REPEAT…UNTIL.
List of Questions
- Why it is advisable to plan the logic of a program before writing it?
- What is an algorithm? What are the characteristics necessary for a sequence of instructions to qualify as an algorithm?
- What are the commonly used ways to represent algorithms? Which of these can be used to solve the corresponding problem on a computer?
- Any program is an algorithm, although the reverse is not true. Discuss this statement.
- What is a program-planning tool? How it is useful? Name two commonly used program-planning tools.
- What is a flowchart?
- How does a flowchart help a programmer in program development?
- Can a person draw a flowchart for a task if he/she does not know how to perform the task manually? Discuss.
- What are the various basic symbols used in flowcharting? Draw their pictorial representation.
- Describe the functions of the various basic flowcharting symbols.
- What is a record? What is a trailer record?
- What is a sentinel value? Discuss its use.
- What is a process loop? What is an infinite loop?
- Why it is necessary to avoid infinite loops in program design?
- “A loop consists of a body, a test for exit condition, and a return provision.” Discuss this statement.
- What is a generalized algorithm? Why should programs be general in nature?
- Discuss the difference between loop control by counting and loop control by use of sentinel value. Which is preferable and why?
- Illustrate the use of a counter to keep track of the number of times a loop has been executed.
- Can there be more than one flowchart for a given problem? Write reasons for your answer.
- What is the difference between a macro flowchart and a micro flowchart? Illustrate with an example.
- What are the various guidelines to be followed while drawing a flowchart?
- Discuss the advantages and limitations of flowcharting.
- Why is proper documentation of a program necessary?
- What are program bugs? What is debugging?
- What is testing of a program? How it is done?
- What is a pseudocode? Why it is so called? Write another name for pseudocode.
- What are the three basic logic structures used in writing structured programs. Discuss the use of each.
- What is structured programming? What are its main advantages?
- Draw flowcharts for the two commonly used structures for selection logic.
- What is the difference between IF…THEN and IF…THEN…ELSE structures?
- Draw flowcharts for the two commonly used structures for iteration logic.
- Both DO…WHILE and REPEAT…UNTIL are used for looping. Discuss the difference between the two structures.
- What is the purpose of ENDIF and ENDDO statements?
- What is indentation? Why it is used in writing pseudocodes?
- Discuss the advantages and limitations of pseudocode.
- Three numbers denoted by the variables A,B, and C are supplied as input data. Draw a flowchart for the logic to identify and print the largest one.
- Draw a flowchart to print a list of all students over the age of 20 in a class. The input records contain the name and age of the students. Assume a sentinel value of 99 for the age field of the trailer record.
- Draw a flowchart to print the name and age of the oldest student and the youngest student in a class. The input records contain the name and age of the students. Assume a sentinel value of 99 for the age field of the trailer record.
- Draw a flowchart for an algorithm to read and print the first 20 records in a data set. Make sure that the processing stops after the twentieth record.
- Input data of all employees of a company has been supplied. The first field of each input record contains the employee number (EmployeeNo). Assume that a trailer record having a sentinel value of 99999 for EmployeeNo terminates the input data of all the employees. Draw a flowchart for the logic to count and print the total number of employees.
- For the problem of question 40, we want to count and print the number of only male employees in the age group of 25 to 30. Assume that the input records contain SexCode and Age fields to provide this information. Draw a flowchart for an algorithm to process this requirement.
- A population survey has been carried out in a city and the collected information has been stored in a computer. Each record contains the name, address, sex, age, profession, etc., of one citizen. We want to print the details of all adults (aged 18 years or more) and finally the total number of adults. Assume a suitable sentinle value for any field in the trailer record, and draw a flowchart for an algorithm to process this requirement.
- A set of examination papers graded with scores from 0 to 100 is to be searched to find how many of them are above 90. The total has to be printed. Draw a flowchart for an algorithm to process this requirement. Assume a suitable sentinel value for the trailer record.
- Each paper in a set of examination papers includes a grade of A, B, C, D, or E. We want to have separate counts of the total number of papers having grade A and those having grade E. The total counts of both types have to be printed at the end. Draw a flowchart for an algorithm to process this requirement. Assume a suitable sentinel value for the trailer record.
- A shopkeeper wants to have a general program for his personal computer that will prepare bills for each customer whenever he sells goods to them. His idea is that as soon as a customer purchases some goods from his shop, he will supply the description, unit price, and the quantity purchased for each item as input to the computer. He wants that with this information the computer should print each item along with its unit price, quantity purchased, and total price. Finally, the computer should also print the total cost of all the items purchased by the customer. Assuming a sentinel value of zero for the quantity purchased field in the trailer record, draw a flowchart for an algorithm to process this requirement.
- An employer plans to pay bonus to each employee. Those earning Rs. 2000 or above are to be paid 10 percent of their salary and those earning less than Rs. 2000 are to be paid Rs. 200. The input records contain the employee number, name, and salary of the employees. The output to be printed should contain the employee number, name, and the amount of bonus to be paid to each employee. Draw a flowchart for an algorithm to process this requirement. Assume a suitable sentinel value for any of the fields in the trailer record.
- An employee’s pay record includes hours worked and pay rate. The gross pay is to be calculated as hours worked times pay rate, and is to be printed for each employee. For the number of hours worked in excess of 40, overtime rate of 1.5 times the regular rate is to be paid. Draw a flowchart for an algorithm to process this requirement. Assume a suitable sentinel value for any of the input fields in the trailer record.
- A data file contains a set of examination scores followed by a trailer record with the value of -1. Draw a flowchart for an algorithm to calculate and print the average of the scores.
- The data file of Question 48 is expanded to include several sets of data each requiring calculation of its average. Each data set is followed by a trailer record with the value of -1, and the last data set is followed by a trailer record with a value of -2. Draw a flowchart for an algorithm to process this requirement.
- Five numbers denoted by the variables A, B, C, D, and E are supplied as input. Draw a flowchart for an algorithm to print these numbers in descending order of magnitude.
- Draw a flowchart for an algorithm to add up all the even numbers between 0 and 100 (both inclusive) and print the result.
- Draw a flowchart for an algorithm to find out whether a given triangle ABC is isosceles. Assume that the angles of the triangle are supplied as input. Print the answer as yes or no.
- Draw a flowchart for an algorithm to find out whether a given triangle ABC is a right-angled triangle. Assume that the sides of the triangle are supplied as input. Print the answer as yes or no.
- Draw a flowchart for an algorithm to find out whether a given quadrilateral ABCD is a rectangle. Assume that all the four angles and four sides of the quadrilateral are supplied as input. Print the answer as yes or no.
- Draw a flowchart for an algorithm to convert a number from base 10 to a new base using the division-remainder technique.
- Draw a flowchart for an algorithm to convert a number form another base to base 10.
- Write a pseudocode to solve the problem described in Question 37.
- Write a pseudocode to solve the problem described in Question 38.
- Write a pseudocode to solve the problem described in Question 41.
- Write a pseudocode to solve the problem described in Question 44.
- Write a pseudocode to solve the problem described in Question 45.
- Write a pseudocode to solve the problem described in Question 46.
- Write a pseudocode to solve the problem described in Question 47.
- Write a pseudocode to solve the problem described in Question 51.
- Write a pseudocode to solve the problem described in Question 52.
- Top 30 Linux Questions (MCQs) with Answers and Explanations
- 75 Important Cybersecurity Questions (MCQs with Answers)
- 260 One-Liner Information Security Questions and Answers for Fast Learning
- Top 20 HTML5 Interview Questions with Answers
- 80 Most Important Network Fundamentals Questions With Answers
- 100 Most Important SOC Analyst Interview Questions
- Top 40 Cyber Security Questions and Answers
- Top 10 React JS Interview Theory Questions and Answers
- CISSP – Practice Test Questions – 2024 – Set 20 (53 Questions)
- Part 2: Exploring Deeper into CCNA – Wireless (145 Practice Test Questions)