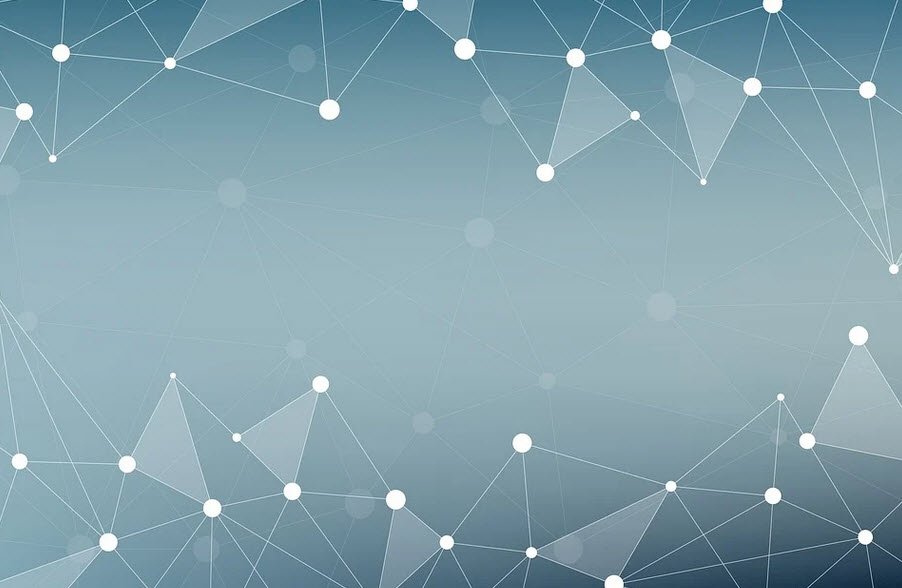
Generally students are asked to write programs which solve simple problems and the small amount of data. Therefore, they need not concern themselves about how the data are stored in computer’s main memory and how slowly or quickly the operations of retrieval and updations are performed.
However, when a complex and time-consuming problem is to be solved or when a large amount of data is to be used then it is very important that the data be organized in main memory so as to give faster access to data and the program be written accordingly.
Also Read:
- Cyber Security and Cyber Forensics – Interview Questions
- List of Most Important 500+ DBMS Interview Questions
- Top 250+ Technical Software Engineering Interview Questions
- Top Questions to Ask During a Computer Forensics Interview
- Top 77 Linux Interview Questions to Ask Candidates
- A to Z – Computer Security Terms and Definitions
- Top 50 Software Engineering Books To Read in 2021
- Important Terms Related To Software Engineering
- [Top 30] Checkpoint Firewall Questions with Answers
- Information Security Policy Related Questions with Answers
- Wireless Networks – Questions With Answers
- 100+ Computer and Network Security Abbreviations
- Windows Server 2016 – Multiple Choice Questions (MCQ) With Answers
Otherwise, main memory space as well as computer time required for various operations may be wasted. In main memory, time to access any location is the same whereas time to access any location is not the same on secondary storage, therefore organization of data in main memory and organization of data in secondary memory ave been discussed separately.
Here is the list of few Data Structure related questions:
- Define
- Data Item
- Record
- File
- Data Structure
- Explain various data structure operation.
- What is the need for data structure ?
- Differentiate between
- Linear and Non-Linear data structure
- Homogenous and Non- Homogenous data structure
- Primitive and Non-Primitive data structure
- Static and dynamic data structure
- What are the various memory management techniques ? Explain
- Define an algorithm. Write the characteristic of an algorithm.
- Briefly describe the notions of
- The complexity of an algorithm.
- The space time tradeoff of algorithms
- Define string and explain the string operation by giving suitable examples.
- Write the algorithm for linear search and find its complexity.
- Briefly describe the big O notation.
- Explain various methods to store a string in memory.
- What do you mean by algorithm complexity? How it is measured?
- What is the role of time-space trade off.
- Define
- Array
- Stack
- Queue
- Linked List
- What is a Pointer? Explain the use of pointer by using example.
- What is a Record? How can you represent a file in memory?
- Define an array. Explain the sequential allocation method.
- Derive the formula to find the address of elements of one-dimensional array.
- Given the following linear arrays :
- A [-5 : 18], B [1967: 2002]
Find the number of elements in each of the arrays A and B.
- A [-5 : 18], B [1967: 2002]
- Given the base address of array A [200 : 250] as 200 and size of each element is 3 words of memory, find addresses of A [215] and A[241].
- Discuss the various operation on linear array.
- Write an algorithm for inserting an element into a linear array.
- Write an algorithm for deleting an element from a linear array.
- Explain sequential representation of two-dimensional arrays and derive the formula for address computation of elements of two-dimensional array. A is a two-dimensional array with 25 rows and 4 columns. Each element of array is stored in 4 memory locations. If base address is 500, then compute the location of A [12, 3]. The organization is row-major.
- Assume that a two-dimensional array A has been stored column-wise. If the element A [2, 2] is stored at location 130 and A [7, 5] at location 156, find out the location where the elements A [6, 6] be located. Each element requires one byte of storage. Can you find the number of rows and columns of the
array A. - Suppose A [2 : 8, – 4 : 1, 6 : 10] is a multi-dimensional array. Suppose base address is 200 and there are 4 words per element. Find the position of the element A [5, -1, 8] when element of the array are stored rowwise.
- Explain general multi-dimensional array.
- Explain the sparse array. How can you store the sparse array in memory? Explain by giving suitable example.
- State the limitation of Arrays.
- What is a stack? Describe any two applications of stack.
- What are the disadvantages of using array representation of stack?
- Define and explain linked stack.
- Write algorithm of PUSH and POP Operations.
- Write algorithm to perform following operations on linked stack
- PUSH
- POP
- Explain the method of evaluating postfix expression by giving suitable example.
- Explain the procedure for transforming infix expression into postfix expression by giving suitable example.
- Describe the concept of Polish notation.
- Translate by inspection and hand each infix expression into its equivalent prefix expression.
- (A-B) (D*E)
- (A- B ↑ C) / (D – E) + F
- Translate the infix expression
- ( (A+B) * C) ↑ (D – E)
into its equivalent postfix expression.
- ( (A+B) * C) ↑ (D – E)
- Evaluate the following postfix expression.
- E = 5 6 2 + * 8 4 / –
- Describe the algorithm of Tower of Hanoi.
- What is Recursion ? Write the recursion algorithm and iterative algorithm for finding the factorial of a number N.
- Write an algorithm for matching of parenthesis
- Check the following expressions of nested parenthesis using stack.
- x = ( () () )
- x = ( ( ( ) )
- Explain the various drawbacks of array.
- What is linked list ? How can you represent a linked list in memory ?
- Explain various operations of linked list.
- Write the algorithm of traversing a linked list.
- Write the algorithm of searching an element from a linked list.
- Write the algorithm of searching an element from a sorted linked list.
- Explain the insertion and deletion operations of linked list by giving suitable example.
- Describe the concept of Free-Storage list.
- Explain the concept of Garbage collection.
- Write the algorithm of a linked list for
- insertion at the beginning
- insertion at the end
- insertion after a given node
- insertion into sorted linked list
- Write the algorithm for copying operation of a linked list.
- Write the deletion algorithm of a linked list.
- Explain the advantages and disadvantages of circular list.
- What is a doubly linked list ? How can you represent a doubly linked list in memory.
- Explain the insertion and deletion operations of a doubly linked list by giving suitable examples.
- Write the insertion algorithm of a doubly linked list.
- Write the deletion algorithm of a doubly linked list.
- Write the insertion and deletion algorithm of a two – way header list.
- Explain the various application of linked list.
- Describe the difference between sequential and linked storage allocations.
- Distinguish between linear and non-linear data structures.
- Define Queue. Give some examples of queues in computer.
- Explain the difference between stack and queue.
- Write the algorithm of INSERT and DELETE operation of the queue.
- Explain the difference between queue and circular queue by giving suitable example.
- Write the algorithm of insert and delete operation of circular queue.
- What do you mean by deque and priority queue?
- State some applications of queue.
- What do you mean by linked queue?
- What are the advantages of linked representation of queues over array representation of queues?
- Define tree and write the properties of a tree.
- Give
- level,
- degree and
- height of each node of the following tree :
- Define binary tree, full binary tree and strictly binary tree. Write the properties of binary tree also.
- Explain the various methods of representation a binary tree in memory.
- Explain the various methods of tree traversal by giving suitable example.
- Write the algorithm of preorder, Inorder and postorder traversal.
- Describe binary search trees and its applications.
- Write the algorithm for searching and inserting a node in binary search tree.
- Write the algorithm for deletion from a binary search tree.
- Draw a diagram of a binary tree T if the preorder and inorder traversal of T yield the following sequence of nodes.
Inorder : E A C K F H D B G
Preorder: F A E K C D H G B - Consider the algebraic expression
E = ( 2 m+n) (3 a – b)2 - Draw the tree which corresponds to the expression E.
- Suppose the following list of letters is inserted in order into an empty binary search tree
J R D G T E M H P A F Q
Find the final tree T. - Given the following binary search tree. Find the tree after the node G is deleted.
- Explain the Traversal Algorithms using stack.
- What do you mean by Searching? What are its types?
- Explain Linear and Binary Search using examples.
- Write an algorithm of Linear Search.
- Write an algorithm of Binary Search.
- Distinguish between Linear and Binary Search.
- What do you mean by Sorting? What are its types?
- Explain Selection and Bubble Sort by using examples.
- Write an algorithm of Selection Sort.
- Write an algorithm of Bubble Sort.
- Explain Insertion Sort. Write its algorithm also.
- Explain algorithm of Merge Sort.
- Explain Quick Sort.
- Write an algorithm of Quick Sort.
- Explain the algorithm of Radix Sort.
- Compare various Sorting Techniques.
- 80 Most Important Network Fundamentals Questions With Answers
- 100 Most Important SOC Analyst Interview Questions
- Top 40 Cyber Security Questions and Answers
- Top 10 React JS Interview Theory Questions and Answers
- CISSP – Practice Test Questions – 2024 – Set 20 (53 Questions)
- Part 2: Exploring Deeper into CCNA – Wireless (145 Practice Test Questions)
- Part 1: Mastering CCNA – Wireless (145 Practice Test Questions)
- [1z0-1085-20] Oracle Cloud Infrastructure Foundations 2020 Associate MCQ Questions – Part 3
- [1z0-1085-20] Oracle Cloud Infrastructure Foundations 2020 Associate MCQ Questions – Part 2
- [1z0-1085-20] Oracle Cloud Infrastructure Foundations 2020 Associate MCQ Questions – Part 1