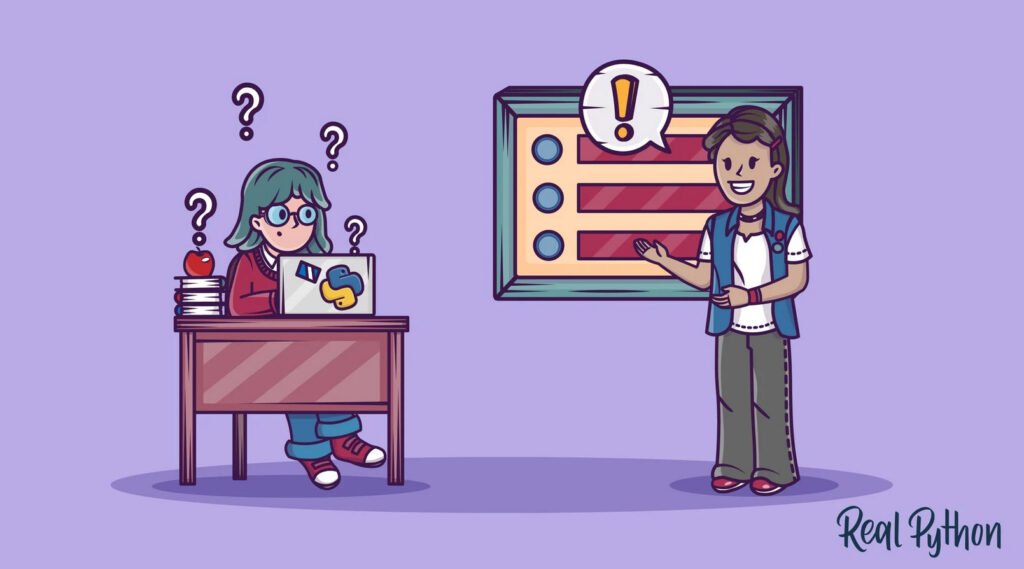
In Python, keywords are reserved words that have specific meanings and functionalities within the language. They cannot be used as identifiers (variable names, function names, etc.) because they play a crucial role in defining the syntax and structure of the Python code. As of Python 3.8, there are 35 keywords.
In this article, we will explore these keywords, categorize them, and understand their individual purposes in Python programming.
Value Keywords
1. True: It represents the boolean value for “not False” or 1.
2. False: This keyword represents the boolean value for “not True” or 0.
3. None: It is used to denote the absence of a value and is often used to initialize variables.
Operator Keywords
4. and: It returns True if both operands are True (similar to the logical “&&” operator in some other programming languages).
5. or: This keyword returns True if either of the operands is True (similar to the logical “||” operator).
6. in: The “in” keyword is used to check if a value is present in an iterator like a list, tuple, or string.
7. is: It returns True if the id of two variables is the same, indicating they refer to the same object in memory.
8. not: This operator returns the opposite Boolean value. If the expression is True, “not” makes it False, and vice versa.
Conditional Keywords
9. if: The “if” keyword is used to create conditional statements. The code within the block following “if” will be executed if the expression is True.
10. elif: Short for “else if”, “elif” is used when multiple conditions need to be checked.
11. else: This block is executed if none of the previous conditions (associated with “if” and “elif”) are True.
Iteration Keywords
12. for: The “for” keyword is used to create loops, particularly for iterating over elements in a sequence like lists or strings.
13. while: The “while” keyword creates a loop that continues as long as the given condition is True.
14. break: It is used to exit or break out of a loop prematurely.
15. continue: The “continue” keyword skips the rest of the loop and proceeds to the next iteration based on a specific condition.
Structure Keywords
16. def: This keyword is used to define user-defined functions.
17. class: The “class” keyword is used to define user-defined classes, which serve as blueprints for objects.
18. lambda: It is used to create anonymous functions or small, one-line functions without a formal name.
19. with: The “with” keyword is used to execute code within the context manager’s scope. It is typically used for resource management.
20. as: It is used to create an alias for modules, classes, or functions.
21. pass: The “pass” keyword is used as a placeholder for creating empty structures (e.g., function or class definitions) that will be filled later.
Returning Keywords
22. return: This keyword is used to get value(s) from a function and exit the function.
23. yield: It is used within functions to create generators, which can produce a sequence of values one at a time, rather than returning a single value.
Import Keywords
24. import: The “import” keyword is used to import modules, libraries, or packages into the current Python script.
25. from: It is used with “import” to import specific functions or classes from a module or package.
Exception Handling Keywords
26. try: This keyword is used to create a block of code that might cause an exception.
27. except: The “except” keyword is used to define a block of code that will be executed if an exception occurs within the “try” block.
28. finally: The “finally” keyword is used to define a block of code that will be executed no matter whether an exception occurred or not.
29. raise: It is used to explicitly raise an exception with a specified message or error.
30. assert: The “assert” keyword checks if a given condition is True and raises an AssertionError if the condition is False.
Asynchronous Programming Keywords
31. async: This keyword is used to define asynchronous functions or coroutines that can be executed concurrently.
32. await: It is used inside asynchronous functions to specify points where control can be returned to the event loop.
Variable Handling Keywords
33. del: The “del” keyword is used to delete or unset a user-defined data, like variables or objects.
34. global: It allows access to variables defined outside the current function’s scope.
35. nonlocal: The “nonlocal” keyword is used to modify variables from different scopes, particularly from an enclosing function.
Understanding these keywords is essential for writing efficient and meaningful Python code. While some keywords define basic programming constructs like loops and conditionals, others play a crucial role in managing exceptions, imports, and defining functions and classes.
As you delve deeper into Python programming, mastering these keywords will enable you to write more sophisticated and robust applications with ease.
Happy coding!
You may also like:- 22 Useful JavaScript Functions You Need To Know
- CSS3 nth-child Selector – A Comprehensive Guide
- PHP Loops – A Comprehensive Guide
- Different Types of Functions in PHP
- Various String Types in PHP – Utilizing ctype Functions
- Understanding Conditional Statements in PHP
- Mastering PHP Arrays – A Comprehensive Guide
- Exploring Strings in PHP – A Comprehensive Guide
- Performing CRUD Operations with PHP and MySQL
- A Guide to PHP File Operations – Opening, Reading, Creating, Writing, and Closing Files