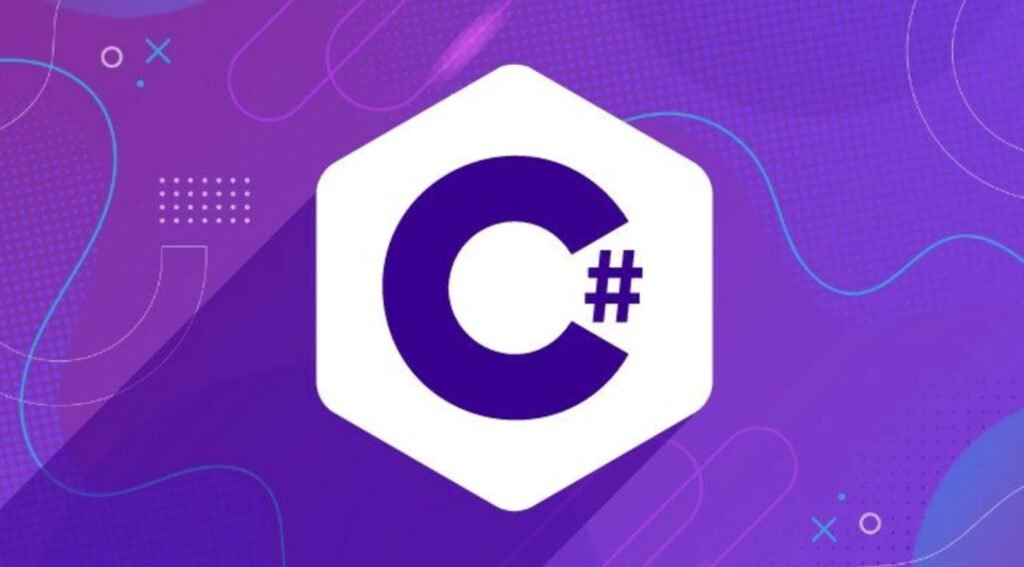
Arrays are fundamental data structures in programming that allow us to store multiple values of the same data type in a single variable. In C#, arrays provide an efficient way to manage collections of elements and access them using index-based notation.
In this article, we will explore the basics of arrays in C#, including their declaration, initialization, and various array operations.
1. Declaration and Initialization
1.1 Initializing Arrays
There are several ways to initialize arrays in C#:
1.1.1 Initializing with Values
int[] nameArray = { 100, 101, 102 };
In this method, we initialize the array ‘nameArray’ with three integer values: 100, 101, and 102. The size of the array is determined automatically based on the number of elements provided.
1.1.2 Initializing an Empty Array
int[] nameArray = new int[3];
In this case, we create an empty integer array named ‘nameArray’ with a size of 3. The elements are initialized to their default values (in this case, integers are initialized to 0).
1.1.3 Accessing Array Elements
int[] nameArray = new int[10];
int firstNumber = nameArray[0];
nameArray[1] = 20;
To access individual elements of an array, we use the square bracket notation along with the index of the element we want to access. In this example, ‘firstNumber’ will be assigned the value at index 0, and we update the value at index 1 to 20.
1.1.4 Multidimensional Arrays
C# also supports multidimensional arrays, which allow us to create arrays with multiple dimensions (rows and columns).
int[,] matrix = new int[2, 2];
matrix[0, 0] = 1;
matrix[0, 1] = 2;
matrix[1, 0] = 3;
matrix[1, 1] = 4;
Here, we declare a 2×2 integer array named ‘matrix’ and assign values to its elements. We use the comma (,) to specify the indices for each dimension.
Alternatively, we can initialize a multidimensional array with predefined values like this:
int[,] predefinedMatrix = new int[2, 2] { { 1, 2 }, { 3, 4 } };
2. Array Operations
2.1 Sorting Arrays
C# provides built-in methods to sort arrays easily:
2.1.1 Sorting in Ascending Order
Array.Sort(nameArray);
This function sorts the elements of ‘nameArray’ in ascending order.
2.1.2 Sorting a Specific Range
Array.Sort(nameArray, 6, 20);
With this method, we can sort a specific portion of the array. In this example, sorting begins at index 6 and covers 20 elements.
2.1.3 Sorting Two Arrays Together
string[] values = { "Juan", "Victor", "Elena" };
string[] keys = { "Jimenez", "Martin", "Ortiz" };
Array.Sort(keys, values);
We can use the `Array.Sort` method to sort one array (‘keys’) and use it as a key to sort another array (‘values’). This sorts both arrays simultaneously, maintaining their correlation.
2.2 Clearing and Copying Arrays
C# also allows us to clear elements from an array and copy elements from one array to another:
2.2.1 Clearing Elements in an Array
Array.Clear(nameArray, 0, nameArray.Length);
The `Array.Clear` method allows us to set a range of elements in ‘nameArray’ to their default values. In this example, we clear all elements in ‘nameArray’.
2.2.2 Copying Elements between Arrays
Array.Copy(sourceArray, targetArray, numberOfElements);
The `Array.Copy` method lets us copy a specified number of elements from ‘sourceArray’ to ‘targetArray’. It is essential to ensure that the ‘targetArray’ has enough space to accommodate the copied elements.
Conclusion
Arrays in C# are versatile and powerful data structures that play a crucial role in managing collections of elements efficiently. By understanding their declaration, initialization, and common operations, you can use arrays effectively in your C# programs to store, manipulate, and process data in various scenarios.
Take advantage of the array operations provided by C# to efficiently sort, clear, and copy elements, making your code more concise and optimized.
You may also like:- 22 Useful JavaScript Functions You Need To Know
- CSS3 nth-child Selector – A Comprehensive Guide
- PHP Loops – A Comprehensive Guide
- Different Types of Functions in PHP
- Various String Types in PHP – Utilizing ctype Functions
- Understanding Conditional Statements in PHP
- Mastering PHP Arrays – A Comprehensive Guide
- Exploring Strings in PHP – A Comprehensive Guide
- Performing CRUD Operations with PHP and MySQL
- A Guide to PHP File Operations – Opening, Reading, Creating, Writing, and Closing Files