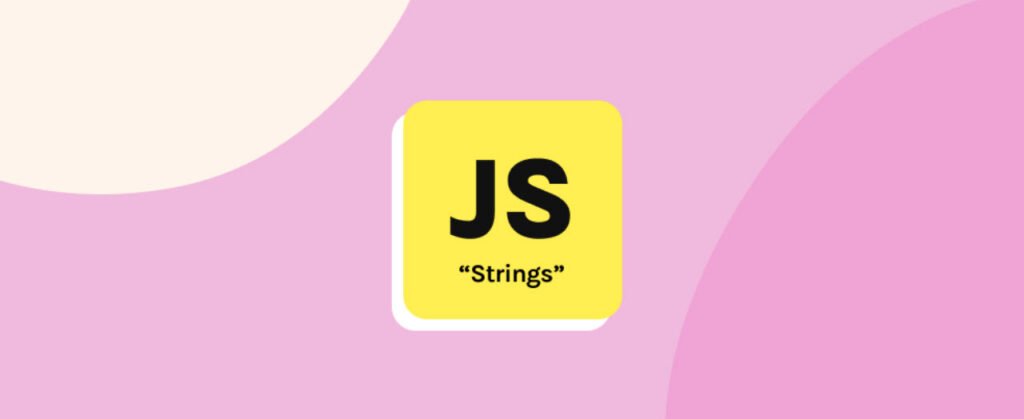
String manipulation is an important aspect of programming, and JavaScript provides a wide range of built-in methods for working with strings. In this article, we will discuss some of the most commonly used string methods in JavaScript and their applications.
- chartAt()
- concat()
- includes()
- indexOf()
- lastIndexOf()
- match()
- replace()
- slice()
- split()
- substr()
- substring()
- toLowerCase()
- toUpperCase()
- trim()
- startWith()
1. charAt()
The charAt() method is used to return the character at a specific index in a string. This method takes an index as an argument and returns the character at that index. For example:
let str = "Hello World!";
console.log(str.charAt(1)); // Output: "e"
2. concat()
The concat() method is used to concatenate two or more strings. This method takes one or more strings as arguments and returns a new string that is a combination of all the strings. For example:
let str1 = "Hello";
let str2 = "World";
let str3 = str1.concat(" ", str2);
console.log(str3); // Output: "Hello World"
3. includes()
The includes() method checks whether a string contains a specified substring and returns a boolean value. This method takes a substring as an argument and returns true if the substring is found in the string, otherwise it returns false. For example:
let str = "Hello World!";
console.log(str.includes("World")); // Output: true
4. indexOf()
The indexOf() method returns the index of the first occurrence of a specified substring in a string. This method takes a substring as an argument and returns the index of the first occurrence of that substring. If the substring is not found in the string, it returns -1. For example:
let str = "Hello World!";
console.log(str.indexOf("o")); // Output: 4
5. lastIndexOf()
The lastIndexOf() method returns the index of the last occurrence of a specified substring in a string. This method takes a substring as an argument and returns the index of the last occurrence of that substring. If the substring is not found in the string, it returns -1. For example:
let str = "Hello World!";
console.log(str.lastIndexOf("o")); // Output: 7
6. match()
The match() method searches a string for a specified pattern and returns an array of matches. This method takes a regular expression as an argument and returns an array of all the matches. For example:
let str = "The rain in Spain falls mainly on the plain";
console.log(str.match(/ain/g)); // Output: ["ain", "ain", "ain"]
7. replace()
The replace() method is used to replace a specified substring with another string. This method takes two arguments: the substring to be replaced, and the new string to replace it with. For example:
let str = "Hello World!";
console.log(str.replace("World", "Universe")); // Output: "Hello Universe!"
8. slice()
The slice() method extracts a section of a string and returns it as a new string. This method takes two arguments: the starting index and the ending index of the section to be extracted. For example:
let str = "Hello World!";
console.log(str.slice(0, 5)); // Output: "Hello"
9. split()
The split() method splits a string into an array of substrings based on a specified separator. This method takes a separator as an argument and returns an array of all the substrings. For example:
let str = "Hello World!";
console.log(str.split(" ")); // Output: ["Hello", "World!"]
10. substr()
The substr() method returns a specified number of characters from a string, starting at a specified index. This method takes two arguments: the starting index and the number of characters to be extracted. For example:
let str = "Hello World!";
console.log(str.substr(6, 5)); // Output: "World"
11. substring()
The substring() method returns a substring of a string between two specified indices. This method takes two arguments: the starting index and the ending index of the substring. For example:
let str = "Hello World!";
console.log(str.substring(6, 11)); // Output: "World"
12. toLowerCase()
The toLowerCase() method is used to convert a string to lowercase. This method returns a new string with all the characters in lowercase. For example:
let str = "Hello World!";
console.log(str.toLowerCase()); // Output: "hello world!"
13. toUpperCase()
The toUpperCase() method is used to convert a string to uppercase. This method returns a new string with all the characters in uppercase. For example:
let str = "Hello World!";
console.log(str.toUpperCase()); // Output: "HELLO WORLD!"
14. trim()
The trim() method removes whitespace from both ends of a string. This method returns a new string with all the whitespace removed. For example:
let str = " Hello World! ";
console.log(str.trim()); // Output: "Hello World!"
15. startsWith()
The startsWith() method checks whether a string starts with a specified substring and returns a boolean value. This method takes a substring as an argument and returns true if the string starts with that substring, otherwise it returns false. For example:
let str = "Hello World!";
console.log(str.startsWith("Hello")); // Output: true
In conclusion, these string methods are essential tools for manipulating strings in JavaScript. By using these methods, you can perform a wide range of operations on strings, such as finding substrings, replacing characters, and converting cases.
You may also like:- 22 Useful JavaScript Functions You Need To Know
- CSS3 nth-child Selector – A Comprehensive Guide
- PHP Loops – A Comprehensive Guide
- Different Types of Functions in PHP
- Various String Types in PHP – Utilizing ctype Functions
- Understanding Conditional Statements in PHP
- Mastering PHP Arrays – A Comprehensive Guide
- Exploring Strings in PHP – A Comprehensive Guide
- Performing CRUD Operations with PHP and MySQL
- A Guide to PHP File Operations – Opening, Reading, Creating, Writing, and Closing Files