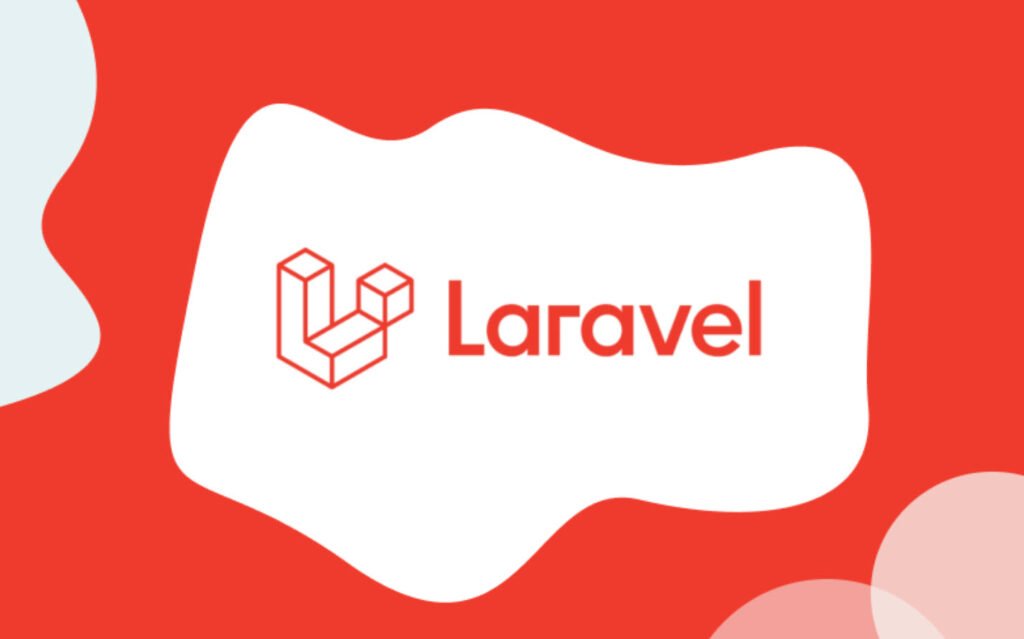
Laravel’s HTTP client, built on top of the Guzzle HTTP Client library, is a powerful tool for making HTTP requests. With its expressive syntax and convenient features, it streamlines communication with external APIs or within your own application. One of its key features is the ability to handle HTTP response codes elegantly.
In this cheat sheet, we’ll explore the Laravel HTTP client’s response codes and the corresponding methods provided by the `Illuminate\Http\Client\Response` object.
HTTP Response Codes and Corresponding Methods
200 OK
$response->ok()
201 Created
$response->created()
202 Accepted
$response->accepted()
204 No Content
$response->noContent()
301 Moved Permanently
$response->movedPermanently()
302 Found
$response->found()
400 Bad Request
$response->badRequest()
401 Unauthorized
$response->unauthorized()
402 Payment Required
$response->paymentRequired()
403 Forbidden
$response->forbidden()
404 Not Found
$response->notFound()
408 Request Timeout
$response->requestTimeout()
409 Conflict
$response->conflict()
422 Unprocessable Entity
$response->unprocessableEntity()
429 Too Many Requests
$response->tooManyRequests()
500 Internal Server Error
$response->serverError()
How to Use the Cheat Sheet
When making HTTP requests with Laravel’s HTTP client, the response from the server is encapsulated in a `Illuminate\Http\Client\Response` object. This object provides a set of convenient methods for checking the response status without manually inspecting the response code.
For example, if you want to check whether the response code is a successful `200 OK`, you can use the following code:
if ($response->ok()) {
// Handle a successful response
} else {
// Handle an unsuccessful response
}
This cheat sheet serves as a quick reference guide, allowing you to seamlessly integrate these methods into your code, enhancing readability and simplifying the handling of HTTP responses in your Laravel applications.
You may also like:- 22 Useful JavaScript Functions You Need To Know
- CSS3 nth-child Selector – A Comprehensive Guide
- PHP Loops – A Comprehensive Guide
- Different Types of Functions in PHP
- Various String Types in PHP – Utilizing ctype Functions
- Understanding Conditional Statements in PHP
- Mastering PHP Arrays – A Comprehensive Guide
- Exploring Strings in PHP – A Comprehensive Guide
- Performing CRUD Operations with PHP and MySQL
- A Guide to PHP File Operations – Opening, Reading, Creating, Writing, and Closing Files