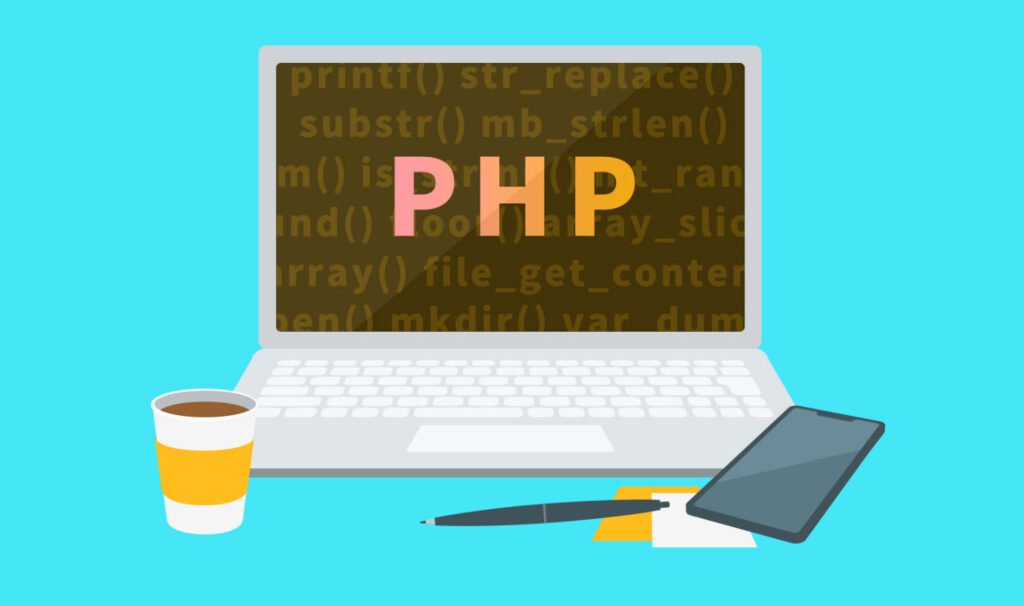
Old functions like utf8_encode and utf8_decode are the functions used in programming languages such as PHP to handle encoding and decoding of Unicode characters.
utf8_encode is a function that takes a string that may contain characters outside of the ASCII range (i.e. characters with code points above 127) and encodes it into a string of bytes using the UTF-8 encoding. UTF-8 is a variable-length encoding scheme that can represent all Unicode code points using one to four bytes. This function is typically used when sending data over a network or storing it in a database, as it ensures that the data is represented in a standard encoding that can be understood by different systems.
utf8_decode, on the other hand, is a function that takes a string of bytes encoded in UTF-8 and decodes it back into a string of Unicode characters. This function is useful when receiving data from an external source that uses UTF-8 encoding, as it allows the program to interpret the data correctly and display it to the user.
Both of these functions are important for ensuring that data is correctly encoded and decoded when working with non-ASCII characters, which are becoming increasingly important as the world becomes more interconnected and diverse.
In PHP 8.2, the functions utf8_encode and utf8_decode have been deprecated and are no longer recommended for use. This means that although they still exist in the language, developers are encouraged to use alternative functions instead.
The reason for this deprecation is that utf8_encode and utf8_decode were designed specifically for working with the UTF-8 encoding, which is only one of several possible encodings for Unicode characters. As a result, these functions could lead to unexpected behavior when working with characters in other encodings, such as UTF-16 or UTF-32.
In place of utf8_encode and utf8_decode, PHP now provides several new functions for working with Unicode characters, including mb_convert_encoding, mb_encode_numericentity, and mb_decode_numericentity. These functions are more flexible and can handle a wider range of encodings, making them a better choice for developers who need to work with non-ASCII characters in their PHP code.
If you are starting a new project, avoid using these two utf8_encode and utf8_decode functions. Instead, you can use the mb_convert_encoding function of the mb extension, or transcode function of the intl extension, or the iconv function.
Check first if any PHP extensions (mb, intl, iconv) are available in your PHP environment to decide which function you can use. If you have a PHP function that still uses the utf8_encode and utf8_decode functions, what you can do to make a smooth transition in just 3 steps:
1. Create a Wrapper Function
The wrapper function can be like this:
function my_utf8_encode($text)
{
return utf8_encode($text);
}
function my_utf8_decode($text)
{
return utf8_decode($text);
}
Then change the implementation of the my_utf8_encode and my_utf8_decode functions to use functions of one of the extensions mentioned above that can do the text encoding conversion.
For instance, if you have the mb extension available in your PHP environment, change the functions like this:
function my_utf8_encode($text)
{
return mb_convert_encoding($text, 'UTF-8', 'ISO-8859-1');
}
function my_utf8_decode($text)
{
return mb_convert_encoding($text, 'ISO-8859-1', 'UTF-8');
}
It’s worth noting that although utf8_encode and utf8_decode have been deprecated, they will still work in PHP 8.2 and future versions of the language. However, developers are encouraged to update their code to use the newer functions to ensure that it remains compatible with future versions of PHP and to avoid any potential issues with character encoding.
You may also like:- 22 Useful JavaScript Functions You Need To Know
- CSS3 nth-child Selector – A Comprehensive Guide
- PHP Loops – A Comprehensive Guide
- Different Types of Functions in PHP
- Various String Types in PHP – Utilizing ctype Functions
- Understanding Conditional Statements in PHP
- Mastering PHP Arrays – A Comprehensive Guide
- Exploring Strings in PHP – A Comprehensive Guide
- Performing CRUD Operations with PHP and MySQL
- A Guide to PHP File Operations – Opening, Reading, Creating, Writing, and Closing Files