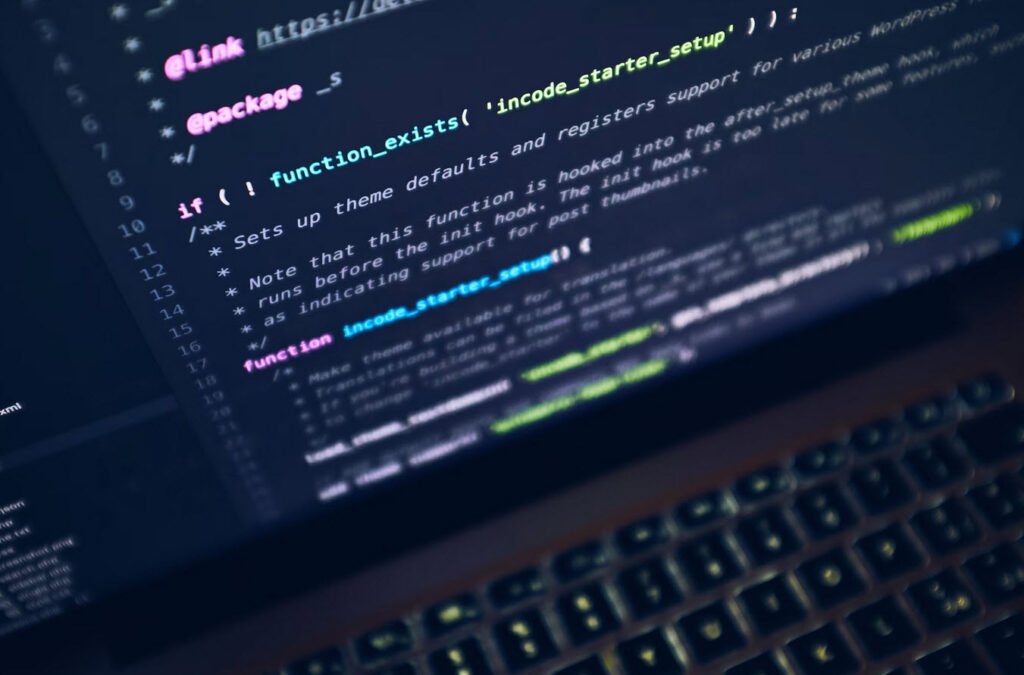
Arrays are one of the most versatile and widely used data structures in programming. They allow developers to store and organize multiple values under a single variable name, making it easier to manage and manipulate data efficiently. In PHP, arrays come in various forms, including indexed arrays, associative arrays, and multidimensional arrays.
This article will explore how to declare arrays in PHP and demonstrate different array types and their usage.
1. Declaring an Indexed Array in PHP:
Indexed arrays are the simplest form of arrays in PHP, where elements are stored with numeric indices starting from 0.
$arr = array("John", "Doe", "Lorem", "Ipsum");
or, using the short syntax introduced in PHP 5.4:
$arr = ["John", "Doe", "Lorem", "Ipsum"];
Indexed arrays are useful when you need a sequential list of items, and you can access elements by their numeric index.
2. Declaring an Associative Array in PHP:
Associative arrays are arrays where elements are stored with named keys instead of numeric indices.
$arr = array("John"=>"10", "Doe"=>"200", "Doe"=>"3000", "Ipsum"=>"40000");
or, using the short syntax:
$arr = ["John"=>"10", "Doe"=>"200", "Doe"=>"3000", "Ipsum"=>"40000"];
Note that in the associative array above, the key “Doe” is duplicated. In PHP, duplicate keys are not allowed, and the last occurrence of the key will overwrite the earlier ones.
Associative arrays are ideal for representing data with key-value pairs, such as database records or configuration settings.
3. Declaring a Multidimensional Array in PHP:
A multidimensional array is an array that contains one or more arrays as its elements. It allows you to create complex data structures with multiple levels of nesting.
$arr = array (
array("John",100,180),
array("Doe",150,130),
array("Lorem",500,200),
array("Ipsum",170,150)
);
or, using the short syntax:
$arr = [
["John",100,180],
["Doe",150,130],
["Lorem",500,200],
["Ipsum",170,150],
];
In the above example, we have a multidimensional array with each sub-array representing data for a person, such as name, age, and height.
Multidimensional arrays are useful for handling complex data structures like tables or matrices.
4. Sorting an Array in PHP:
PHP provides several built-in functions to sort arrays in different orders.
- `sort($arr)` - Sorts indexed arrays in ascending order.
- `rsort($arr)` - Sorts indexed arrays in descending order.
- `asort($arr)` - Sorts associative arrays in ascending order, according to the value.
- `ksort($arr)` - Sorts associative arrays in ascending order, according to the key.
- `arsort($arr)` - Sorts associative arrays in descending order, according to the value.
- `krsort($arr)` - Sorts associative arrays in descending order, according to the key.
Example:
$numbers = [5, 2, 8, 1, 3];
sort($numbers); // [1, 2, 3, 5, 8]
rsort($numbers); // [8, 5, 3, 2, 1]
Associative arrays are sorted based on their values or keys, respectively.
Conclusion
Arrays are a fundamental part of PHP, providing a flexible way to store and manage data efficiently. In this article, we covered how to declare indexed, associative, and multidimensional arrays in PHP, using both the traditional and short syntax. Additionally, we explored various array sorting functions that allow you to order arrays in different ways.
Understanding arrays and their manipulation methods is essential for PHP developers to build robust and dynamic applications effectively.
You may also like:- 22 Useful JavaScript Functions You Need To Know
- CSS3 nth-child Selector – A Comprehensive Guide
- PHP Loops – A Comprehensive Guide
- Different Types of Functions in PHP
- Various String Types in PHP – Utilizing ctype Functions
- Understanding Conditional Statements in PHP
- Mastering PHP Arrays – A Comprehensive Guide
- Exploring Strings in PHP – A Comprehensive Guide
- Performing CRUD Operations with PHP and MySQL
- A Guide to PHP File Operations – Opening, Reading, Creating, Writing, and Closing Files