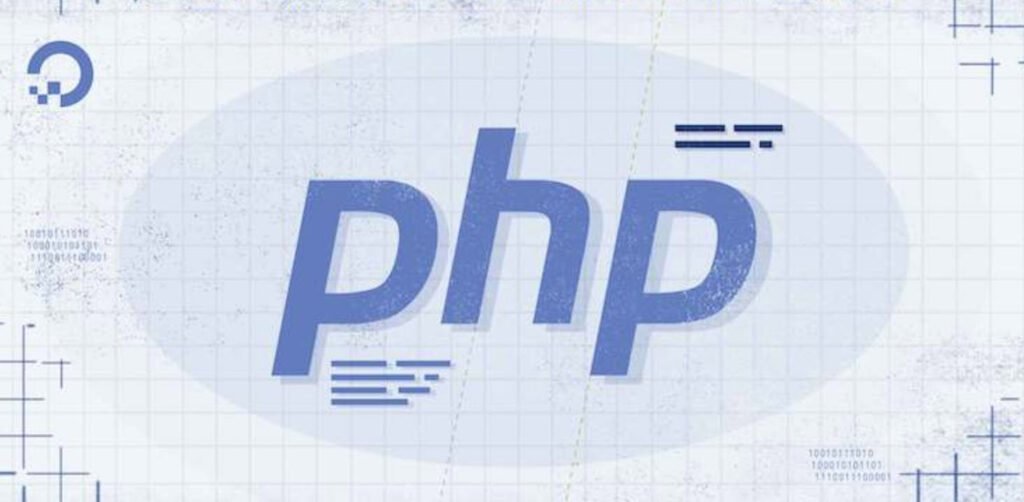
Functions are one of the core building blocks of any programming language, including PHP. They allow developers to encapsulate reusable blocks of code, making it easier to organize and manage complex tasks. In PHP, functions are defined using the `function` keyword, and they can accept parameters, have return types, and support various advanced features introduced in PHP 8.
This article will explore PHP functions and demonstrate their usage with examples.
1. Simple Function:
A simple function in PHP is defined using the `function` keyword, followed by the function name and a pair of parentheses. Parameters, if any, are specified inside the parentheses.
function greet() {
echo "Hello, World!";
}
2. Function with Return Type:
You can specify the return type of a function by adding a colon `:` followed by the desired type after the parameter list. The return type indicates the data type that the function will return.
function add($a, $b) : int {
return $a + $b;
}
3. Function with Optional Parameter:
In PHP, you can define a function with optional parameters by assigning a default value to the parameter in the function signature.
function greetUser($name = 'Guest') : string {
return "Hello, $name!";
}
4. Function with Typed Parameter:
With PHP 7, you can enforce the data type of a function parameter by specifying it in the function signature. Adding a question mark `?` before the type allows the parameter to be nullable.
function printLength(?string $text) : ?int {
return $text ? strlen($text) : null;
}
5. Function with Union Types (PHP >= 8.0):
PHP 8 introduced union types, allowing you to accept multiple data types for a parameter or specify multiple possible return types for a function.
function processValue(int|string $value) : int|string {
if (is_int($value)) {
return $value * 2;
} else {
return "Invalid";
}
}
6. Function Call:
To call a function, simply use its name followed by parentheses containing the required arguments.
greet(); // Outputs: "Hello, World!"
$result = add(5, 3);
echo $result; // Outputs: 8
$greeting = greetUser("John");
echo $greeting; // Outputs: "Hello, John!"
7. Null Safe Operator (PHP >= 8.0):
The null safe operator (`?->`) allows you to safely access methods and properties of an object without causing an error if the object is null.
class MyClass {
public function getName() : ?string {
return "Alice";
}
}
$myObject = new MyClass();
$name = $myObject?->getName();
echo $name; // Outputs: "Alice"
$myObject = null;
$name = $myObject?->getName();
echo $name; // Outputs: Nothing, no error thrown
Conclusion
PHP functions are powerful tools that help organize code and promote reusability. They allow developers to create modular and maintainable applications by breaking complex tasks into smaller, manageable components.
With the addition of advanced features like return types, optional parameters, typed parameters, union types, and the null safe operator in PHP 8, functions have become more versatile and robust. By understanding the various functionalities of PHP functions and their usage, developers can write cleaner, more efficient, and error-resistant code, leading to more reliable and scalable PHP applications.
You may also like:- 22 Useful JavaScript Functions You Need To Know
- CSS3 nth-child Selector – A Comprehensive Guide
- PHP Loops – A Comprehensive Guide
- Different Types of Functions in PHP
- Various String Types in PHP – Utilizing ctype Functions
- Understanding Conditional Statements in PHP
- Mastering PHP Arrays – A Comprehensive Guide
- Exploring Strings in PHP – A Comprehensive Guide
- Performing CRUD Operations with PHP and MySQL
- A Guide to PHP File Operations – Opening, Reading, Creating, Writing, and Closing Files