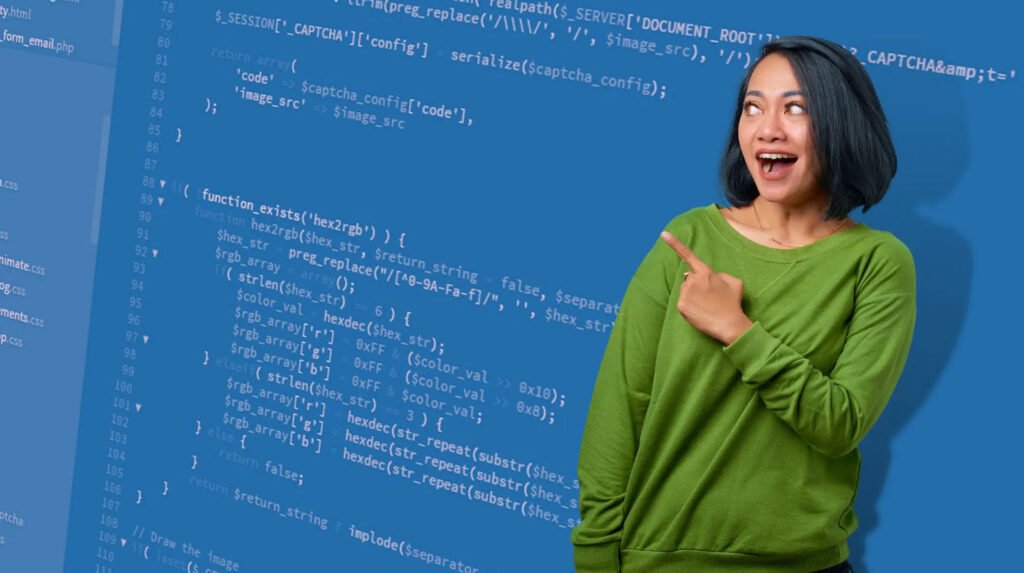
In PHP, null is a distinct data type representing a variable with no assigned value. Whether a variable is set to null explicitly or through the `settype()` function, it signifies the absence of a meaningful value.
This article explores various techniques to handle null values in PHP, offering a comprehensive guide for developers.
1. The `is_null()` Function
The `is_null()` function serves as a fundamental tool to check whether a variable is null or not. It returns `true` if the variable is null and `false` otherwise.
$foo = null;
if (is_null($foo)) {
echo '$foo is null';
}
// Output: $foo is null
Note that `is_null()` solely checks for null values and does not determine whether a variable is set. To verify if a variable is set, combine it with the `isset()` function.
2. The Null Coalescing Operator (??)
Introduced in PHP 7.0, the null coalescing operator (`??`) provides an elegant way to handle null values. It returns the value of the variable if it’s not null; otherwise, it returns the value of the second operand.
$foo = null;
echo $foo ?? 'bar';
// Output: bar
Chaining the null coalescing operator allows checking multiple variables in succession.
$foo = null;
echo $foo ?? $bar ?? 'baz';
// Output: baz
Be cautious, as the null coalescing operator will return the second operand even if the variable is not set or undefined.
echo $bar ?? 'foo';
// Output: foo
3. The Null Coalescing Assignment Operator (??=)
Introduced as part of PHP 7.4, the null coalescing assignment operator (`??=`) simplifies assigning a value to a variable only if it’s null.
$foo = null;
$foo ??= 'bar';
echo $foo;
// Output: bar
4. The Nullsafe Operator (?->)
Introduced in PHP 8.0, the nullsafe operator (`?->`) facilitates safe method/property calls on a variable. It returns the method value if the variable is not null and null otherwise.
class User
{
public function getName()
{
return 'John Doe';
}
}
$user = null;
echo $user?->getName();
// Output: null
$user = new User;
echo $user?->getName();
// Output: John Doe
5. The Ternary Operator
While not as recommended for readability, the ternary operator can still be used to check if a variable is null.
$foo = null;
echo $foo ? $foo : 'bar';
// Output: bar
6. Nullable Types
Since PHP 7.1, you can explicitly declare function parameters or return types as nullable using the `?` operator.
function foo(?string $bar): ?string
{
return $bar;
}
echo foo(null);
// Output: null
echo foo('bar');
// Output: bar
7. Null as a Standalone Type (PHP 8.2 onwards)
Starting from PHP 8.2, you can use `null` as a standalone type. This allows declaring variables or function return types explicitly as `null`.
class Nil
{
public null $nil = null;
public function isNull(null $nil): null
{
return null;
}
}
$nil = new Nil;
$nil->isNull(null);
// Returns: null
$nil->isNull('foo');
// Fatal error: Argument #1 ($nil) must be of type null, string given
Embracing these techniques equips developers with a versatile set of tools for handling null values in PHP, promoting cleaner, more readable code that gracefully manages variables without assigned values.
You may also like:- 22 Useful JavaScript Functions You Need To Know
- CSS3 nth-child Selector – A Comprehensive Guide
- PHP Loops – A Comprehensive Guide
- Different Types of Functions in PHP
- Various String Types in PHP – Utilizing ctype Functions
- Understanding Conditional Statements in PHP
- Mastering PHP Arrays – A Comprehensive Guide
- Exploring Strings in PHP – A Comprehensive Guide
- Performing CRUD Operations with PHP and MySQL
- A Guide to PHP File Operations – Opening, Reading, Creating, Writing, and Closing Files