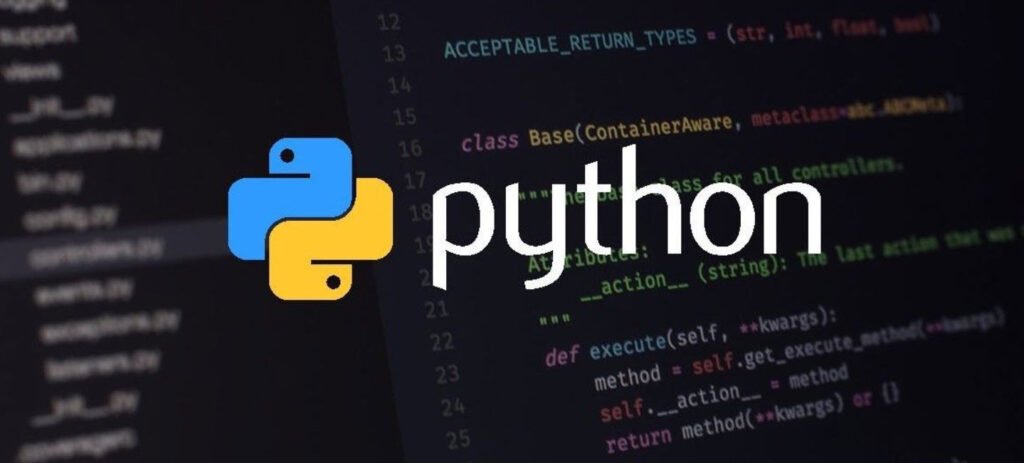
In the world of programming, Python stands out as one of the most popular and versatile languages. One of its fundamental concepts is the use of variables, which serve as containers for storing and managing data. In Python, variables play a crucial role in making your code more organized, readable, and efficient.
In this article, we will explore how to store strings in variables in Python and understand why it is a valuable practice.
Understanding Variables in Python
Before delving into string storage, it’s essential to grasp the concept of variables in Python. Variables are like symbolic labels that you attach to specific data values, allowing you to refer to and manipulate those values more easily. They act as placeholders for data, making it simpler to work with complex programs.
In Python, you can create a variable by following these simple rules:
- Choose a name for your variable, which should be descriptive and follow Python’s naming conventions (e.g., avoid starting with a number or using special characters).
- Use the assignment operator (=) to associate the variable name with a value.
- The value can be of various types, including numbers, strings, lists, or more complex data structures.
Storing Strings in Variables
Strings, as a fundamental data type in Python, can be easily stored in variables. Here’s how you can do it:
my_str = "Hello World"
In the above line of code:
- `my_str` is the variable name.
- `=` is the assignment operator.
- `”Hello World”` is the string value assigned to the variable.
Now that the string “Hello World” is stored in the variable `my_str`, you can easily access and manipulate it in your Python code.
The Benefits of Using Variables for Strings
Why should you bother storing strings in variables when you can use them directly in your code? There are several compelling reasons:
1. Readability: Using descriptive variable names makes your code more readable and self-explanatory. Instead of seeing the same string repeated throughout your code, you can use a meaningful variable name that provides context.
2. Maintainability: If you need to change the string value, you only have to do it in one place—where the variable is defined. This reduces the risk of introducing errors when making updates.
3. Efficiency: By storing strings in variables, you save memory and processing power. If you repeatedly use the same string in your code without storing it in a variable, Python has to recreate that string each time it’s used, consuming unnecessary resources.
4. Consistency: Variables ensure that you always refer to the same string throughout your program, preventing accidental variations or typos that might occur when using the same string multiple times.
Accessing Stored Strings
Once you’ve stored a string in a variable, you can access it simply by using the variable name. For example:
print(my_str) # Output: Hello World
This way, you retrieve the string stored in `my_str` and display it to the console.
Conclusion
Storing strings in variables is a fundamental practice in Python programming that enhances code readability, maintainability, efficiency, and consistency. By encapsulating strings within variables, you not only make your code more organized but also set the stage for building more complex and powerful Python programs.
So, the next time you work with strings in Python, remember to harness the power of variables to make your coding journey smoother and more enjoyable.
You may also like:- 22 Useful JavaScript Functions You Need To Know
- CSS3 nth-child Selector – A Comprehensive Guide
- PHP Loops – A Comprehensive Guide
- Different Types of Functions in PHP
- Various String Types in PHP – Utilizing ctype Functions
- Understanding Conditional Statements in PHP
- Mastering PHP Arrays – A Comprehensive Guide
- Exploring Strings in PHP – A Comprehensive Guide
- Performing CRUD Operations with PHP and MySQL
- A Guide to PHP File Operations – Opening, Reading, Creating, Writing, and Closing Files