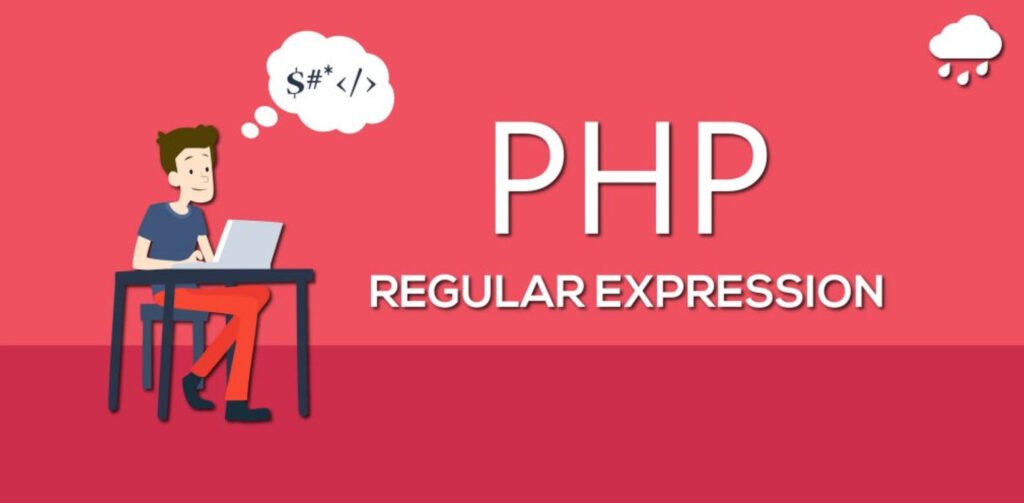
Regular expressions are powerful tools used for pattern matching and string manipulation in PHP. They allow developers to perform complex searches, replacements, and validations on text data using pattern-based rules. PHP provides several built-in functions to work with regular expressions, offering immense flexibility and efficiency in handling text processing tasks.
In this article, we will explore the most commonly used PHP regular expression functions and demonstrate their functionalities with examples.
- preg_match()
- preg_match_all()
- preg_replace()
- preg_split()
- preg_grep()
- preg_quote()
1. preg_match():
The `preg_match()` function searches a string for a specific pattern defined by a regular expression. It returns true if the pattern is found at least once in the string, and false if no match is found.
$string = "The quick brown fox jumps over the lazy dog.";
if (preg_match('/fox/', $string)) {
echo "Pattern found!";
} else {
echo "Pattern not found!";
}
2. preg_match_all():
The `preg_match_all()` function is similar to `preg_match()`, but it matches all occurrences of the pattern in the input string.
$string = "The quick brown fox jumps over the lazy dog.";
preg_match_all('/\b\w+\b/', $string, $matches);
print_r($matches[0]);
3. preg_replace():
The `preg_replace()` function performs a search and replace operation using regular expressions. It allows you to replace all occurrences of the pattern with a specified replacement string.
$string = "The quick brown fox jumps over the lazy dog.";
$newString = preg_replace('/fox/', 'cat', $string);
echo $newString;
4. preg_split():
`preg_split()` allows you to split a string into an array using regular expressions as the delimiter. This is useful for complex text parsing and data extraction.
$string = "apple,orange,banana";
$fruits = preg_split('/,/', $string);
print_r($fruits);
5. preg_grep():
The `preg_grep()` function searches an array for elements that match a given regular expression. It returns an array containing all matching elements.
$words = ['apple', 'banana', 'orange', 'grape', 'pear'];
$matches = preg_grep('/^a/', $words);
print_r($matches);
6. preg_quote():
The `preg_quote()` function is used to quote regular expression characters in a given string. It ensures that the input string is treated as a literal string and not interpreted as a regular expression pattern.
$specialChars = '[test]';
$quotedString = preg_quote($specialChars, '/');
echo $quotedString; // Output: \[test\]
Conclusion
PHP regular expression functions provide a powerful and flexible way to manipulate strings and perform pattern matching in a variety of scenarios. They offer immense utility in tasks such as data validation, search and replace, and text parsing.
By mastering these PHP regular expression functions and understanding the syntax of regular expressions, developers can efficiently handle complex text processing tasks and create more sophisticated and dynamic applications. However, it is important to use regular expressions judiciously and test them thoroughly to ensure accurate and efficient text processing without causing unintended side effects.
You may also like:- 22 Useful JavaScript Functions You Need To Know
- CSS3 nth-child Selector – A Comprehensive Guide
- PHP Loops – A Comprehensive Guide
- Different Types of Functions in PHP
- Various String Types in PHP – Utilizing ctype Functions
- Understanding Conditional Statements in PHP
- Mastering PHP Arrays – A Comprehensive Guide
- Exploring Strings in PHP – A Comprehensive Guide
- Performing CRUD Operations with PHP and MySQL
- A Guide to PHP File Operations – Opening, Reading, Creating, Writing, and Closing Files