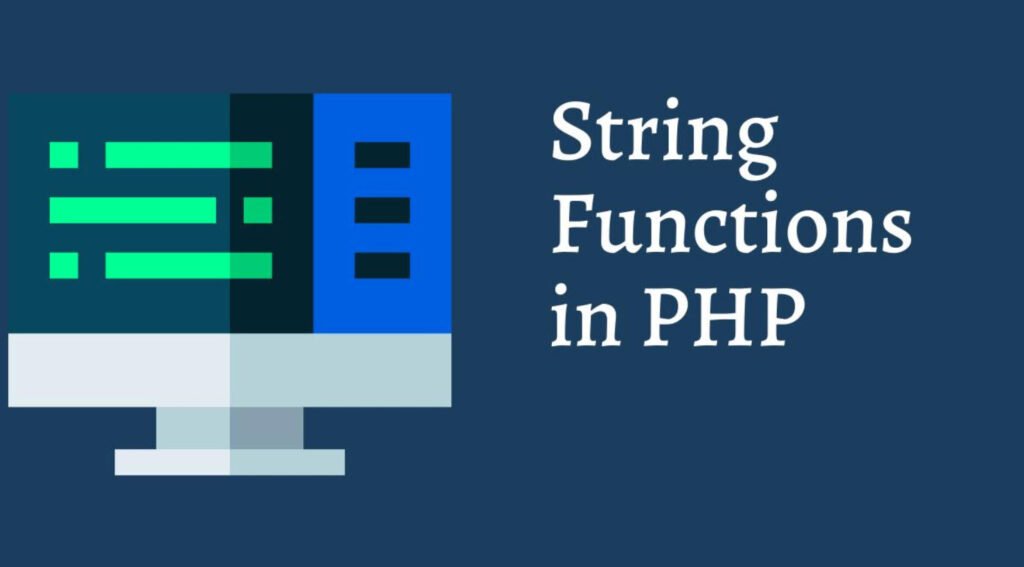
String manipulation is a fundamental aspect of programming in PHP and is used extensively in web development, data processing, and various other applications. PHP offers a wide range of built-in functions to perform various operations on strings efficiently.
In this article, we will explore some of the most useful string manipulation methods in PHP, showcasing their functionalities and how they can be utilized effectively.
1. str_contains($string, $substring) [PHP >= 8.0]
The `str_contains()` function is a handy addition in PHP 8.0 that allows you to check if a string contains a specified substring. It returns a boolean value, `true` if the substring is found, and `false` if not.
$string = 'Hello World';
if (str_contains($string, 'cheat')) {
echo "Substring found!";
} else {
echo "Substring not found!";
}
2. str_replace($search, $replace, $subject)
The `str_replace()` function is widely used to replace all occurrences of a search string with a given replacement string within a subject string.
$string = 'Hello World';
$newString = str_replace('Hello', 'Bonjour', $string);
echo $newString; // Output: Bonjour World
3. strcmp($str1, $str2)
`strcmp()` compares two strings and returns 0 if they are equal. It returns a negative value if the first string is less than the second, and a positive value if the first string is greater.
$string = 'Hello World';
$result = strcmp($string, 'Awesome cheatsheets');
if ($result == 0) {
echo "Strings are equal.";
} elseif ($result < 0) {
echo "First string is less than the second.";
} else {
echo "First string is greater than the second.";
}
4. strpos($haystack, $needle, $offset)
`strpos()` is used to find the position of the first occurrence of a substring within a string. It returns the position (index) of the substring if found, or `false` if the substring is not present.
$string = 'Hello World';
$position = strpos($string, 'o');
echo $position; // Output: 4
5. str_split($string, $split_length)
The `str_split()` function divides a string into an array of smaller chunks, each with a specified length (`$split_length`). If the second parameter is omitted, the default chunk length is 1.
$string = 'Hello World';
$chunks = str_split($string, 2);
print_r($chunks); // Output: Array ( [0] => He [1] => ll [2] => o [3] => W [4] => or [5] => ld )
6. strrev($string)
`strrev()` reverses a string, effectively flipping it backward.
$string = 'Hello World';
$reversedString = strrev($string);
echo $reversedString; // Output: dlroW olleH
7. trim($string)
The `trim()` function removes whitespace (or other characters specified) from the beginning and end of a string.
$string = ' Hello World ';
$trimmedString = trim($string);
echo $trimmedString; // Output: 'Hello World'
8. ucfirst($string) and lcfirst($string)
`ucfirst()` capitalizes the first character of a string, while `lcfirst()` makes the first character lowercase.
$string = 'hello world';
$ucfirst = ucfirst($string); // Output: 'Hello world'
$lcfirst = lcfirst($string); // Output: 'hello world'
9. substr($string, $start, $length)
The `substr()` function extracts a part of a string starting from the specified index (`$start`) and optionally up to a specified length (`$length`).
$string = 'Hello World';
$substring = substr($string, 0, 5);
echo $substring; // Output: 'Hello'
Conclusion
String manipulation is an essential aspect of PHP programming, and mastering the various string functions can greatly enhance your ability to handle and manipulate textual data effectively. In this article, we covered some of the most useful string manipulation methods in PHP, including finding substrings, replacing text, comparing strings, splitting, reversing, and altering cases.
By incorporating these functions into your PHP projects, you can perform complex string operations efficiently and build more robust and dynamic applications.
You may also like:- 22 Useful JavaScript Functions You Need To Know
- CSS3 nth-child Selector – A Comprehensive Guide
- PHP Loops – A Comprehensive Guide
- Different Types of Functions in PHP
- Various String Types in PHP – Utilizing ctype Functions
- Understanding Conditional Statements in PHP
- Mastering PHP Arrays – A Comprehensive Guide
- Exploring Strings in PHP – A Comprehensive Guide
- Performing CRUD Operations with PHP and MySQL
- A Guide to PHP File Operations – Opening, Reading, Creating, Writing, and Closing Files