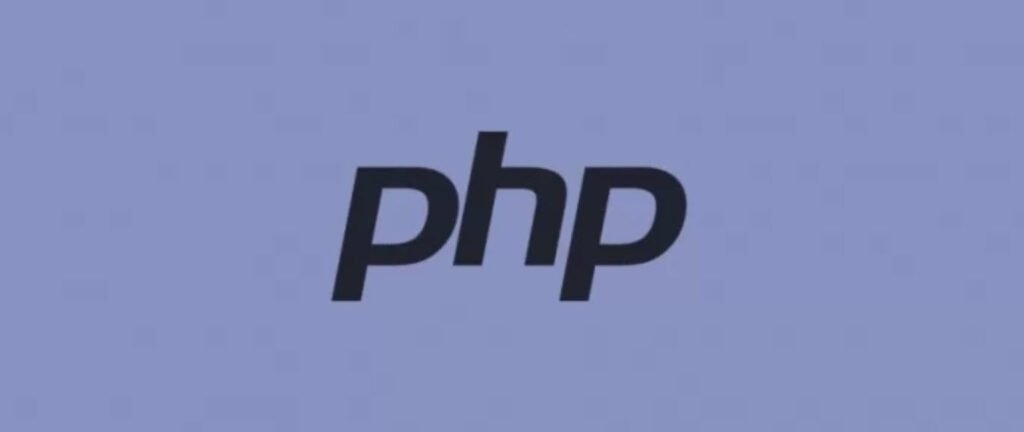
As a PHP developer, you often encounter tasks that require data validation or string manipulation. Luckily, PHP offers a wide range of functions and regular expressions to handle these tasks efficiently.
In this article, we’ll explore some useful PHP code snippets that can be used for validating email addresses, usernames, domains, extracting domain names from URLs, and highlighting words in content.
1. Validating Email Addresses:
To validate email addresses, PHP provides the `filter_var` function along with the `FILTER_VALIDATE_EMAIL` filter. It offers a simple and reliable way to check if an email address has a valid syntax.
$email = 'test+email@fexample.com';
if (filter_var($email, FILTER_VALIDATE_EMAIL)) {
echo "Your email is valid.";
} else {
echo "Wrong email address format.";
}
2. Validating Usernames:
To validate usernames, you can use regular expressions to enforce certain rules, such as minimum and maximum character lengths and allowed characters.
$username = "user_name12";
if (preg_match('/^[a-z\d_]{5,20}$/i', $username)) {
echo "Your username is valid.";
} else {
echo "Wrong username format.";
}
3. Validating Domains:
To validate domains, you can use regular expressions to check for valid URL formats, including http, https, and ftp protocols.
$url = "http://domain-name.com/";
if (preg_match('/^(http|https|ftp):\/\/([A-Z0-9][A-Z0-9_-]*(?:\.[A-Z0-9][A-Z0-9_-]*)+):?(\d+)?\/?/i', $url)) {
echo "Your URL is valid.";
} else {
echo "Wrong URL.";
}
4. Extracting Domain Names from URLs:
To extract domain names from URLs, you can use regular expressions along with `preg_match` to capture the desired part of the URL.
$url = "http://domain-name.com/index.html";
preg_match('@^(?:http://)?([^/]+)@i', $url, $matches);
$host = $matches[1];
echo $host; // Output: domain-name.com
5. Highlighting a Word in Content:
To highlight specific words in content, you can use `preg_replace` along with regular expressions and word boundaries (`\b`) to replace the target word with the desired content.
$text = "A regular expression (shortened as regex) is a sequence of characters that define a search pattern. Usually such patterns are used by string-searching algorithms for 'find' or 'find and replace' operations on strings, or for input validation.";
$text = preg_replace("/\b(regex)\b/i", 'replaced content', $text);
echo $text;
// Output: A regular expression (shortened as replaced content) is a sequence of characters that define a search pattern. Usually such patterns are used by string-searching algorithms for 'find' or 'find and replace' operations on strings, or for input validation.
Conclusion
These PHP code snippets demonstrate how to perform common validation tasks and string manipulation using regular expressions and built-in functions. With these tools, PHP developers can efficiently validate user input, extract useful information from URLs, and manipulate content to enhance user experiences and data processing capabilities.
It is essential to use these snippets judiciously and adapt them to suit the specific requirements of your PHP applications. Regular expressions are powerful, but they can also become complex, so make sure to test and validate their behavior thoroughly. By leveraging these useful PHP code snippets, you can streamline your development process and create more robust and dynamic web applications.
You may also like:- 22 Useful JavaScript Functions You Need To Know
- CSS3 nth-child Selector – A Comprehensive Guide
- PHP Loops – A Comprehensive Guide
- Different Types of Functions in PHP
- Various String Types in PHP – Utilizing ctype Functions
- Understanding Conditional Statements in PHP
- Mastering PHP Arrays – A Comprehensive Guide
- Exploring Strings in PHP – A Comprehensive Guide
- Performing CRUD Operations with PHP and MySQL
- A Guide to PHP File Operations – Opening, Reading, Creating, Writing, and Closing Files